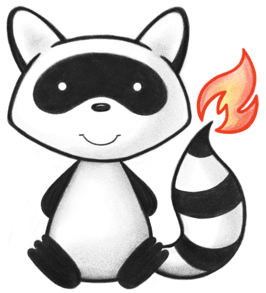
001/* 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.util; 021 022import javax.xml.namespace.NamespaceContext; 023import javax.xml.stream.XMLStreamException; 024import javax.xml.stream.XMLStreamWriter; 025 026public class NonPrettyPrintWriterWrapper implements XMLStreamWriter { 027 028 private static final String PRE = "pre"; 029 private XMLStreamWriter myTarget; 030 private int myInsidePre = 0; 031 032 public NonPrettyPrintWriterWrapper(XMLStreamWriter target) { 033 myTarget = target; 034 } 035 036 @Override 037 public void flush() throws XMLStreamException { 038 myTarget.flush(); 039 } 040 041 @Override 042 public void close() throws XMLStreamException { 043 myTarget.close(); 044 } 045 046 @Override 047 @CoverageIgnore 048 public String getPrefix(String theUri) throws XMLStreamException { 049 return myTarget.getPrefix(theUri); 050 } 051 052 @Override 053 @CoverageIgnore 054 public void setPrefix(String thePrefix, String theUri) throws XMLStreamException { 055 myTarget.setPrefix(thePrefix, theUri); 056 } 057 058 @Override 059 @CoverageIgnore 060 public void setDefaultNamespace(String theUri) throws XMLStreamException { 061 myTarget.setDefaultNamespace(theUri); 062 } 063 064 @Override 065 @CoverageIgnore 066 public void setNamespaceContext(NamespaceContext theContext) throws XMLStreamException { 067 myTarget.setNamespaceContext(theContext); 068 } 069 070 @Override 071 @CoverageIgnore 072 public NamespaceContext getNamespaceContext() { 073 return myTarget.getNamespaceContext(); 074 } 075 076 @Override 077 public void writeStartElement(String theLocalName) throws XMLStreamException { 078 if (PRE.equals(theLocalName) || myInsidePre > 0) { 079 myInsidePre++; 080 } 081 myTarget.writeStartElement(theLocalName); 082 } 083 084 @Override 085 public void writeStartElement(String theNamespaceURI, String theLocalName) throws XMLStreamException { 086 if (PRE.equals(theLocalName) || myInsidePre > 0) { 087 myInsidePre++; 088 } 089 myTarget.writeStartElement(theNamespaceURI, theLocalName); 090 } 091 092 @Override 093 public void writeStartElement(String thePrefix, String theLocalName, String theNamespaceURI) 094 throws XMLStreamException { 095 if (PRE.equals(theLocalName) || myInsidePre > 0) { 096 myInsidePre++; 097 } 098 myTarget.writeStartElement(thePrefix, theLocalName, theNamespaceURI); 099 } 100 101 @Override 102 @CoverageIgnore 103 public void writeEmptyElement(String theNamespaceURI, String theLocalName) throws XMLStreamException { 104 myTarget.writeEmptyElement(theNamespaceURI, theLocalName); 105 } 106 107 @Override 108 @CoverageIgnore 109 public void writeEmptyElement(String thePrefix, String theLocalName, String theNamespaceURI) 110 throws XMLStreamException { 111 myTarget.writeEmptyElement(thePrefix, theLocalName, theNamespaceURI); 112 } 113 114 @Override 115 @CoverageIgnore 116 public void writeEmptyElement(String theLocalName) throws XMLStreamException { 117 myTarget.writeEmptyElement(theLocalName); 118 } 119 120 @Override 121 public void writeEndElement() throws XMLStreamException { 122 if (myInsidePre > 0) { 123 myInsidePre--; 124 } 125 myTarget.writeEndElement(); 126 } 127 128 @Override 129 public void writeEndDocument() throws XMLStreamException { 130 myTarget.writeEndDocument(); 131 } 132 133 @Override 134 public void writeAttribute(String theLocalName, String theValue) throws XMLStreamException { 135 myTarget.writeAttribute(theLocalName, theValue); 136 } 137 138 @Override 139 @CoverageIgnore 140 public void writeAttribute(String thePrefix, String theNamespaceURI, String theLocalName, String theValue) 141 throws XMLStreamException { 142 myTarget.writeAttribute(thePrefix, theNamespaceURI, theLocalName, theValue); 143 } 144 145 @Override 146 @CoverageIgnore 147 public void writeAttribute(String theNamespaceURI, String theLocalName, String theValue) throws XMLStreamException { 148 myTarget.writeAttribute(theNamespaceURI, theLocalName, theValue); 149 } 150 151 @Override 152 public void writeNamespace(String thePrefix, String theNamespaceURI) throws XMLStreamException { 153 myTarget.writeNamespace(thePrefix, theNamespaceURI); 154 } 155 156 @Override 157 public void writeDefaultNamespace(String theNamespaceURI) throws XMLStreamException { 158 myTarget.writeDefaultNamespace(theNamespaceURI); 159 } 160 161 @Override 162 public void writeComment(String theData) throws XMLStreamException { 163 myTarget.writeComment(theData); 164 } 165 166 @Override 167 @CoverageIgnore 168 public void writeProcessingInstruction(String theTarget) throws XMLStreamException { 169 myTarget.writeProcessingInstruction(theTarget); 170 } 171 172 @Override 173 @CoverageIgnore 174 public void writeProcessingInstruction(String theTarget, String theData) throws XMLStreamException { 175 myTarget.writeProcessingInstruction(theTarget, theData); 176 } 177 178 @Override 179 @CoverageIgnore 180 public void writeCData(String theData) throws XMLStreamException { 181 myTarget.writeCData(theData); 182 } 183 184 @Override 185 @CoverageIgnore 186 public void writeDTD(String theDtd) throws XMLStreamException { 187 myTarget.writeDTD(theDtd); 188 } 189 190 @Override 191 @CoverageIgnore 192 public void writeEntityRef(String theName) throws XMLStreamException { 193 myTarget.writeEntityRef(theName); 194 } 195 196 @Override 197 @CoverageIgnore 198 public void writeStartDocument() throws XMLStreamException { 199 myTarget.writeStartDocument(); 200 } 201 202 @Override 203 @CoverageIgnore 204 public void writeStartDocument(String theVersion) throws XMLStreamException { 205 myTarget.writeStartDocument(theVersion); 206 } 207 208 @Override 209 public void writeStartDocument(String theEncoding, String theVersion) throws XMLStreamException { 210 myTarget.writeStartDocument(theEncoding, theVersion); 211 } 212 213 @Override 214 public void writeCharacters(String theText) throws XMLStreamException { 215 if (myInsidePre > 0) { 216 myTarget.writeCharacters(theText); 217 } else { 218 writeCharacters(theText.toCharArray(), 0, theText.length()); 219 } 220 } 221 222 @Override 223 public void writeCharacters(char[] theText, int theStart, int theLen) throws XMLStreamException { 224 writeCharacters(theText, theStart, theLen, myTarget, myInsidePre); 225 } 226 227 static void writeCharacters(char[] theText, int theStart, int theLen, XMLStreamWriter target, int insidePre) 228 throws XMLStreamException { 229 if (theLen > 0) { 230 if (insidePre > 0) { 231 target.writeCharacters(theText, theStart, theLen); 232 } else { 233 int initialEnd = theStart + (theLen - 1); 234 int start = theStart; 235 int end = initialEnd; 236 while (Character.isWhitespace(theText[start]) && start < end) { 237 start++; 238 } 239 while (Character.isWhitespace(theText[end]) && end > start) { 240 end--; 241 } 242 if (start == end) { 243 if (Character.isWhitespace(theText[start])) { 244 target.writeCharacters(" "); 245 return; 246 } 247 } 248 if (start > theStart) { 249 target.writeCharacters(" "); 250 } 251 target.writeCharacters(theText, start, (end - start) + 1); 252 if (end < initialEnd) { 253 target.writeCharacters(" "); 254 } 255 } 256 } 257 } 258 259 @Override 260 @CoverageIgnore 261 public Object getProperty(String theName) throws IllegalArgumentException { 262 return myTarget.getProperty(theName); 263 } 264}