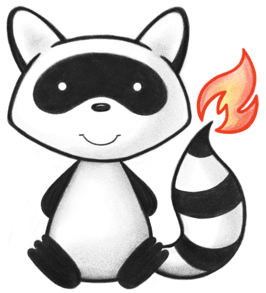
001/*- 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.util; 021 022/** 023 * A utility class for thread sleeps. 024 * Uses non-static methods for easier mocking and unnecessary waits in unit tests 025 */ 026public class SleepUtil { 027 private static final org.slf4j.Logger ourLog = org.slf4j.LoggerFactory.getLogger(SleepUtil.class); 028 029 public void sleepAtLeast(long theMillis) { 030 sleepAtLeast(theMillis, true); 031 } 032 033 @SuppressWarnings("BusyWait") 034 public void sleepAtLeast(long theMillis, boolean theLogProgress) { 035 long start = System.currentTimeMillis(); 036 while (System.currentTimeMillis() <= start + theMillis) { 037 try { 038 long timeSinceStarted = System.currentTimeMillis() - start; 039 long timeToSleep = Math.max(0, theMillis - timeSinceStarted); 040 if (theLogProgress) { 041 ourLog.info("Sleeping for {}ms", timeToSleep); 042 } 043 Thread.sleep(timeToSleep); 044 } catch (InterruptedException e) { 045 Thread.currentThread().interrupt(); 046 ourLog.error("Interrupted", e); 047 } 048 } 049 } 050}