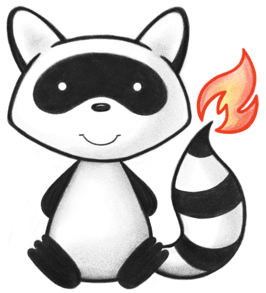
001package ca.uhn.fhir.util; 002 003/*- 004 * #%L 005 * HAPI FHIR - Core Library 006 * %% 007 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 008 * %% 009 * Licensed under the Apache License, Version 2.0 (the "License"); 010 * you may not use this file except in compliance with the License. 011 * You may obtain a copy of the License at 012 * 013 * http://www.apache.org/licenses/LICENSE-2.0 014 * 015 * Unless required by applicable law or agreed to in writing, software 016 * distributed under the License is distributed on an "AS IS" BASIS, 017 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 018 * See the License for the specific language governing permissions and 019 * limitations under the License. 020 * #L% 021 */ 022 023import com.google.common.collect.Streams; 024import jakarta.annotation.Nonnull; 025 026import java.util.ArrayList; 027import java.util.Collection; 028import java.util.Iterator; 029import java.util.List; 030import java.util.function.Consumer; 031import java.util.stream.Stream; 032 033/** 034 * This utility takes an input collection, breaks it up into chunks of a 035 * given maximum chunk size, and then passes those chunks to a consumer for 036 * processing. Use this to break up large tasks into smaller tasks. 037 * 038 * @since 6.6.0 039 * @param <T> The type for the chunks 040 */ 041public class TaskChunker<T> { 042 043 public static <T> void chunk(List<T> theInput, int theChunkSize, Consumer<List<T>> theBatchConsumer) { 044 if (theInput.size() <= theChunkSize) { 045 theBatchConsumer.accept(theInput); 046 return; 047 } 048 chunk((Collection<T>) theInput, theChunkSize, theBatchConsumer); 049 } 050 051 public static <T> void chunk(Collection<T> theInput, int theChunkSize, Consumer<List<T>> theBatchConsumer) { 052 List<T> input; 053 if (theInput instanceof List) { 054 input = (List<T>) theInput; 055 } else { 056 input = new ArrayList<>(theInput); 057 } 058 059 for (int i = 0; i < input.size(); i += theChunkSize) { 060 int to = i + theChunkSize; 061 to = Math.min(to, input.size()); 062 List<T> batch = input.subList(i, to); 063 theBatchConsumer.accept(batch); 064 } 065 } 066 067 @Nonnull 068 public static <T> Stream<List<T>> chunk(Stream<T> theStream, int theChunkSize) { 069 return StreamUtil.partition(theStream, theChunkSize); 070 } 071 072 public static <T> void chunk(Iterator<T> theIterator, int theChunkSize, Consumer<List<T>> theListConsumer) { 073 chunk(Streams.stream(theIterator), theChunkSize).forEach(theListConsumer); 074 } 075}