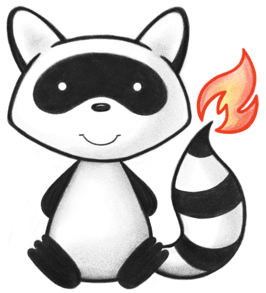
001/*- 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.util; 021 022import ca.uhn.fhir.i18n.Msg; 023import ca.uhn.fhir.system.HapiSystemProperties; 024import com.google.common.annotations.VisibleForTesting; 025import org.slf4j.Logger; 026import org.slf4j.LoggerFactory; 027 028import java.time.Duration; 029 030public class TimeoutManager { 031 private static final Logger ourLog = LoggerFactory.getLogger(TimeoutManager.class); 032 033 private final StopWatch myStopWatch = new StopWatch(); 034 private final String myServiceName; 035 private final Duration myWarningTimeout; 036 private final Duration myErrorTimeout; 037 038 private boolean warned = false; 039 private boolean errored = false; 040 041 public TimeoutManager(String theServiceName, Duration theWarningTimeout, Duration theErrorTimeout) { 042 myServiceName = theServiceName; 043 myWarningTimeout = theWarningTimeout; 044 myErrorTimeout = theErrorTimeout; 045 } 046 047 /** 048 * 049 * @return true if a message was logged 050 */ 051 public boolean checkTimeout() { 052 boolean retval = false; 053 if (myStopWatch.getMillis() > myWarningTimeout.toMillis() && !warned) { 054 ourLog.warn(myServiceName + " has run for {}", myStopWatch); 055 warned = true; 056 retval = true; 057 } 058 if (myStopWatch.getMillis() > myErrorTimeout.toMillis() && !errored) { 059 if (HapiSystemProperties.isUnitTestModeEnabled()) { 060 throw new TimeoutException( 061 Msg.code(2133) + myServiceName + " timed out after running for " + myStopWatch); 062 } else { 063 ourLog.error(myServiceName + " has run for {}", myStopWatch); 064 errored = true; 065 retval = true; 066 } 067 } 068 return retval; 069 } 070 071 @VisibleForTesting 072 void addTimeForUnitTest(Duration theDuration) { 073 myStopWatch.setNowForUnitTest(myStopWatch.getStartedDate().getTime() + theDuration.toMillis()); 074 } 075}