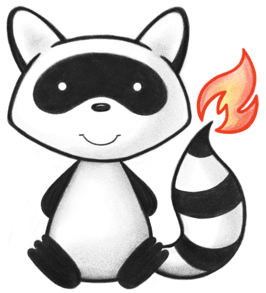
001/* 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.util; 021 022import ca.uhn.fhir.i18n.Msg; 023import ca.uhn.fhir.rest.server.exceptions.InvalidRequestException; 024import ca.uhn.fhir.rest.server.exceptions.ResourceNotFoundException; 025import ca.uhn.fhir.rest.server.exceptions.UnprocessableEntityException; 026 027import static org.apache.commons.lang3.StringUtils.isBlank; 028import static org.apache.commons.lang3.StringUtils.length; 029 030public class ValidateUtil { 031 032 /** 033 * Throws {@link IllegalArgumentException} if theValue is <= theMinimum 034 */ 035 public static void isGreaterThan(long theValue, long theMinimum, String theMessage) { 036 if (theValue <= theMinimum) { 037 throw new IllegalArgumentException(Msg.code(1762) + theMessage); 038 } 039 } 040 041 /** 042 * Throws {@link IllegalArgumentException} if theValue is < theMinimum 043 */ 044 public static void isGreaterThanOrEqualTo(long theValue, long theMinimum, String theMessage) { 045 if (theValue < theMinimum) { 046 throw new IllegalArgumentException(Msg.code(1763) + theMessage); 047 } 048 } 049 050 public static void isNotBlankOrThrowIllegalArgument(String theString, String theMessage) { 051 if (isBlank(theString)) { 052 throw new IllegalArgumentException(Msg.code(1764) + theMessage); 053 } 054 } 055 056 public static void isNotBlankOrThrowInvalidRequest(String theString, String theMessage) { 057 if (isBlank(theString)) { 058 throw new InvalidRequestException(Msg.code(1765) + theMessage); 059 } 060 } 061 062 public static void isNotBlankOrThrowUnprocessableEntity(String theString, String theMessage) { 063 if (isBlank(theString)) { 064 throw new UnprocessableEntityException(Msg.code(1766) + theMessage); 065 } 066 } 067 068 public static void isNotNullOrThrowUnprocessableEntity(Object theObject, String theMessage, Object... theValues) { 069 if (theObject == null) { 070 throw new UnprocessableEntityException(Msg.code(1767) + String.format(theMessage, theValues)); 071 } 072 } 073 074 public static void isNotTooLongOrThrowIllegalArgument(String theString, int theMaxLength, String theMessage) { 075 if (length(theString) > theMaxLength) { 076 throw new IllegalArgumentException(Msg.code(1768) + theMessage); 077 } 078 } 079 080 public static void isTrueOrThrowInvalidRequest(boolean theSuccess, String theMessage, Object... theValues) { 081 if (!theSuccess) { 082 throw new InvalidRequestException(Msg.code(1769) + String.format(theMessage, theValues)); 083 } 084 } 085 086 public static void isTrueOrThrowResourceNotFound(boolean theSuccess, String theMessage, Object... theValues) { 087 if (!theSuccess) { 088 throw new ResourceNotFoundException(Msg.code(2494) + String.format(theMessage, theValues)); 089 } 090 } 091 092 public static void exactlyOneNotNullOrThrowInvalidRequestException(Object[] theObjects, String theMessage) { 093 int count = 0; 094 for (Object next : theObjects) { 095 if (next != null) { 096 count++; 097 } 098 } 099 if (count != 1) { 100 throw new InvalidRequestException(Msg.code(1770) + theMessage); 101 } 102 } 103}