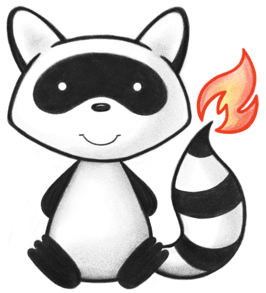
001/*- 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.util; 021 022public enum VersionEnum { 023 V0_1, 024 V0_2, 025 V0_3, 026 V0_4, 027 V0_5, 028 V0_6, 029 V0_7, 030 V0_8, 031 V0_9, 032 033 V1_0, 034 V1_1, 035 V1_2, 036 V1_3, 037 V1_4, 038 V1_5, 039 V1_6, 040 041 V2_0, 042 V2_1, 043 V2_2, 044 V2_3, 045 V2_4, 046 V2_5, 047 V2_5_0, 048 V3_0_0, 049 V3_1_0, 050 V3_2_0, 051 V3_3_0, 052 V3_4_0, 053 V3_5_0, 054 V3_6_0, 055 V3_7_0, 056 V3_8_0, 057 V4_0_0, 058 V4_0_3, 059 V4_1_0, 060 V4_2_0, 061 @Deprecated 062 V4_3_0, // 4.3.0 was renamed to 5.0.0 during the cycle 063 V5_0_0, 064 V5_0_1, 065 V5_0_2, 066 V5_1_0, 067 V5_2_0, 068 V5_2_1, 069 V5_3_0, 070 V5_3_1, 071 V5_3_2, 072 V5_3_3, 073 V5_4_0, 074 V5_4_1, 075 V5_4_2, 076 V5_5_0, 077 V5_5_1, 078 V5_5_2, 079 V5_5_3, 080 V5_5_4, 081 V5_5_5, 082 V5_5_6, 083 V5_5_7, 084 V5_6_0, 085 V5_6_1, 086 V5_6_2, 087 V5_6_3, 088 V5_6_4, 089 V5_7_0, 090 V5_7_1, 091 V5_7_2, 092 V5_7_3, 093 V5_7_4, 094 V5_7_5, 095 V5_7_6, 096 V6_0_0, 097 V6_0_1, 098 V6_0_2, 099 V6_0_3, 100 V6_0_4, 101 V6_0_5, 102 V6_1_0, 103 V6_1_1, 104 V6_1_2, 105 V6_1_3, 106 V6_1_4, 107 V6_2_0, 108 V6_2_1, 109 V6_2_2, 110 V6_2_3, 111 V6_2_4, 112 V6_2_5, 113 // Dev Build 114 V6_3_0, 115 116 V6_4_0, 117 V6_4_1, 118 V6_4_2, 119 V6_4_3, 120 V6_4_4, 121 V6_4_5, 122 V6_4_6, 123 124 V6_5_0, 125 126 V6_6_0, 127 V6_6_1, 128 V6_6_2, 129 130 V6_7_0, 131 V6_8_0, 132 V6_8_1, 133 V6_8_2, 134 V6_8_3, 135 V6_8_4, 136 V6_8_5, 137 V6_8_6, 138 V6_8_7, 139 V6_8_8, 140 141 V6_9_0, 142 143 V6_10_0, 144 V6_10_1, 145 V6_10_2, 146 V6_10_3, 147 V6_10_4, 148 V6_10_5, 149 150 V6_11_0, 151 152 V7_0_0, 153 V7_0_1, 154 V7_0_2, 155 V7_0_3, 156 157 V7_1_0, 158 V7_2_0, 159 V7_2_1, 160 V7_2_2, 161 V7_2_3, 162 163 V7_3_0, 164 V7_4_0, 165 V7_4_1, 166 V7_4_2, 167 V7_4_3, 168 V7_4_4, 169 V7_4_5, 170 171 V7_5_0, 172 V7_6_0, 173 V7_6_1, 174 V7_7_0, 175 V7_8_0; 176 177 public static VersionEnum latestVersion() { 178 VersionEnum[] values = VersionEnum.values(); 179 return values[values.length - 1]; 180 } 181 182 public static VersionEnum forVersion(String theVersionString) { 183 String constantName = "V" + (theVersionString.replace('.', '_')); 184 return valueOf(constantName); 185 } 186 187 public boolean isNewerThan(VersionEnum theVersionEnum) { 188 return ordinal() > theVersionEnum.ordinal(); 189 } 190 191 public String getVersionedDocsSlug() { 192 return this.name().replace("V", "").replaceAll("_", "."); 193 } 194}