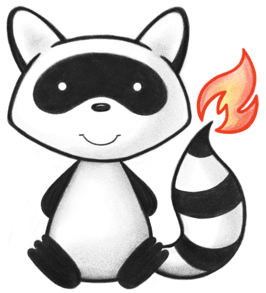
001/* 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.util; 021 022import ca.uhn.fhir.system.HapiSystemProperties; 023import org.apache.commons.lang3.StringUtils; 024 025import java.io.InputStream; 026import java.util.Properties; 027 028import static org.apache.commons.lang3.StringUtils.defaultIfBlank; 029 030/** 031 * Used internally by HAPI to log the version of the HAPI FHIR framework 032 * once, when the framework is first loaded by the classloader. 033 */ 034public class VersionUtil { 035 036 private static final org.slf4j.Logger ourLog = org.slf4j.LoggerFactory.getLogger(VersionUtil.class); 037 private static String ourVersion; 038 private static String ourBuildNumber; 039 private static String ourBuildTime; 040 private static boolean ourSnapshot; 041 042 static { 043 initialize(); 044 } 045 046 public static boolean isSnapshot() { 047 return ourSnapshot; 048 } 049 050 public static String getBuildNumber() { 051 return ourBuildNumber; 052 } 053 054 public static String getBuildTime() { 055 return ourBuildTime; 056 } 057 058 public static String getVersion() { 059 return ourVersion; 060 } 061 062 private static void initialize() { 063 try (InputStream is = VersionUtil.class.getResourceAsStream("/ca/uhn/fhir/hapi-fhir-base-build.properties")) { 064 065 Properties p = new Properties(); 066 if (is != null) { 067 p.load(is); 068 } 069 070 ourVersion = p.getProperty("hapifhir.version"); 071 ourVersion = defaultIfBlank(ourVersion, "(unknown)"); 072 073 ourSnapshot = ourVersion.contains("SNAPSHOT"); 074 075 ourBuildNumber = StringUtils.left(p.getProperty("hapifhir.buildnumber"), 10); 076 ourBuildTime = p.getProperty("hapifhir.timestamp"); 077 078 if (!HapiSystemProperties.isSuppressHapiFhirVersionLogEnabled()) { 079 String buildNumber = ourBuildNumber; 080 if (isSnapshot()) { 081 buildNumber = buildNumber + "/" + getBuildDate(); 082 } 083 084 ourLog.info("HAPI FHIR version {} - Rev {}", ourVersion, buildNumber); 085 } 086 087 } catch (Exception e) { 088 ourLog.warn("Unable to determine HAPI version information", e); 089 } 090 } 091 092 public static String getBuildDate() { 093 return ourBuildTime.substring(0, 10); 094 } 095}