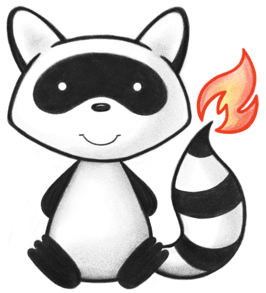
001/*- 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.util; 021 022import org.slf4j.Logger; 023import org.slf4j.LoggerFactory; 024 025import java.io.Reader; 026import java.io.StringReader; 027 028public class XmlDetectionUtil { 029 030 private static final Logger ourLog = LoggerFactory.getLogger(XmlDetectionUtil.class); 031 private static Boolean ourStaxPresent; 032 033 /** 034 * This method will return <code>true</code> if a StAX XML parsing library is present 035 * on the classpath 036 */ 037 public static boolean isStaxPresent() { 038 Boolean retVal = ourStaxPresent; 039 if (retVal == null) { 040 try { 041 Class.forName("javax.xml.stream.events.XMLEvent"); 042 Class<?> xmlUtilClazz = Class.forName("ca.uhn.fhir.util.XmlUtil"); 043 xmlUtilClazz.getMethod("createXmlReader", Reader.class).invoke(xmlUtilClazz, new StringReader("")); 044 ourStaxPresent = Boolean.TRUE; 045 retVal = Boolean.TRUE; 046 } catch (Throwable t) { 047 ourLog.info("StAX not detected on classpath, XML processing will be disabled"); 048 ourStaxPresent = Boolean.FALSE; 049 retVal = Boolean.FALSE; 050 } 051 } 052 return retVal; 053 } 054}