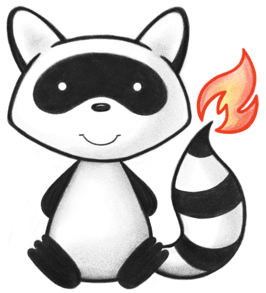
001/*- 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.util.bundle; 021 022import ca.uhn.fhir.context.BaseRuntimeChildDefinition; 023import ca.uhn.fhir.context.BaseRuntimeElementCompositeDefinition; 024import ca.uhn.fhir.context.FhirContext; 025import ca.uhn.fhir.util.ParametersUtil; 026import org.hl7.fhir.instance.model.api.IBase; 027import org.hl7.fhir.instance.model.api.IBaseResource; 028import org.hl7.fhir.instance.model.api.IPrimitiveType; 029 030public class BundleEntryMutator { 031 private final IBase myEntry; 032 private final BaseRuntimeChildDefinition myRequestChildDef; 033 private final BaseRuntimeElementCompositeDefinition<?> myRequestChildContentsDef; 034 private final FhirContext myFhirContext; 035 private final BaseRuntimeElementCompositeDefinition<?> myEntryDefinition; 036 037 public BundleEntryMutator( 038 FhirContext theFhirContext, 039 IBase theEntry, 040 BaseRuntimeChildDefinition theRequestChildDef, 041 BaseRuntimeElementCompositeDefinition<?> theChildContentsDef, 042 BaseRuntimeElementCompositeDefinition<?> theEntryDefinition) { 043 myFhirContext = theFhirContext; 044 myEntry = theEntry; 045 myRequestChildDef = theRequestChildDef; 046 myRequestChildContentsDef = theChildContentsDef; 047 myEntryDefinition = theEntryDefinition; 048 } 049 050 void setRequestUrl(FhirContext theFhirContext, String theRequestUrl) { 051 BaseRuntimeChildDefinition requestUrlChildDef = myRequestChildContentsDef.getChildByName("url"); 052 IPrimitiveType<?> url = ParametersUtil.createUri(theFhirContext, theRequestUrl); 053 for (IBase nextRequest : myRequestChildDef.getAccessor().getValues(myEntry)) { 054 requestUrlChildDef.getMutator().addValue(nextRequest, url); 055 } 056 } 057 058 @SuppressWarnings("unchecked") 059 public void setFullUrl(String theFullUrl) { 060 IPrimitiveType<String> value = (IPrimitiveType<String>) 061 myFhirContext.getElementDefinition("uri").newInstance(); 062 value.setValue(theFullUrl); 063 064 BaseRuntimeChildDefinition fullUrlChild = myEntryDefinition.getChildByName("fullUrl"); 065 fullUrlChild.getMutator().setValue(myEntry, value); 066 } 067 068 public void setResource(IBaseResource theUpdatedResource) { 069 BaseRuntimeChildDefinition resourceChild = myEntryDefinition.getChildByName("resource"); 070 resourceChild.getMutator().setValue(myEntry, theUpdatedResource); 071 } 072 073 public void setRequestIfNoneExist(FhirContext theFhirContext, String theIfNoneExist) { 074 BaseRuntimeChildDefinition requestUrlChildDef = myRequestChildContentsDef.getChildByName("ifNoneExist"); 075 IPrimitiveType<?> url = ParametersUtil.createString(theFhirContext, theIfNoneExist); 076 for (IBase nextRequest : myRequestChildDef.getAccessor().getValues(myEntry)) { 077 requestUrlChildDef.getMutator().addValue(nextRequest, url); 078 } 079 } 080}