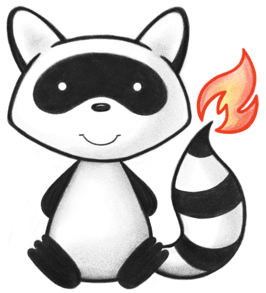
001/* 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.validation; 021 022import java.util.Collections; 023import java.util.HashMap; 024import java.util.Map; 025 026public enum ResultSeverityEnum { 027 028 /** 029 * The issue has no relation to the degree of success of the action 030 */ 031 INFORMATION("information"), 032 033 /** 034 * The issue is not important enough to cause the action to fail, but may cause it to be performed suboptimally or in a way that is not as desired 035 */ 036 WARNING("warning"), 037 038 /** 039 * The issue is sufficiently important to cause the action to fail 040 */ 041 ERROR("error"), 042 043 /** 044 * The issue caused the action to fail, and no further checking could be performed 045 */ 046 FATAL("fatal"); 047 048 private static Map<String, ResultSeverityEnum> ourValues; 049 private String myCode; 050 051 private ResultSeverityEnum(String theCode) { 052 myCode = theCode; 053 } 054 055 public String getCode() { 056 return myCode; 057 } 058 059 public static ResultSeverityEnum fromCode(String theCode) { 060 if (ourValues == null) { 061 HashMap<String, ResultSeverityEnum> values = new HashMap<String, ResultSeverityEnum>(); 062 for (ResultSeverityEnum next : values()) { 063 values.put(next.getCode(), next); 064 } 065 ourValues = Collections.unmodifiableMap(values); 066 } 067 return ourValues.get(theCode); 068 } 069 070 /** 071 * Convert from Enum ordinal to Enum type. 072 * 073 * Usage: 074 * 075 * <code>ResultSeverityEnum resultSeverityEnum = ResultSeverityEnum.values[ordinal];</code> 076 */ 077 public static final ResultSeverityEnum values[] = values(); 078}