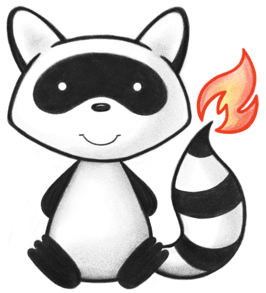
001/* 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.validation; 021 022import org.apache.commons.lang3.builder.EqualsBuilder; 023import org.apache.commons.lang3.builder.HashCodeBuilder; 024import org.apache.commons.lang3.builder.ToStringBuilder; 025import org.apache.commons.lang3.builder.ToStringStyle; 026 027import java.util.List; 028 029public class SingleValidationMessage { 030 031 private Integer myLocationCol; 032 private Integer myLocationLine; 033 private String myLocationString; 034 private String myMessage; 035 private String myMessageId; 036 private ResultSeverityEnum mySeverity; 037 private List<String> mySliceMessages; 038 039 /** 040 * Constructor 041 */ 042 public SingleValidationMessage() { 043 super(); 044 } 045 046 @Override 047 public boolean equals(Object obj) { 048 if (this == obj) { 049 return true; 050 } 051 if (obj == null) { 052 return false; 053 } 054 if (!(obj instanceof SingleValidationMessage)) { 055 return false; 056 } 057 SingleValidationMessage other = (SingleValidationMessage) obj; 058 EqualsBuilder b = new EqualsBuilder(); 059 b.append(myLocationCol, other.myLocationCol); 060 b.append(myLocationLine, other.myLocationLine); 061 b.append(myLocationString, other.myLocationString); 062 b.append(myMessage, other.myMessage); 063 b.append(mySeverity, other.mySeverity); 064 b.append(mySliceMessages, other.mySliceMessages); 065 return b.isEquals(); 066 } 067 068 public Integer getLocationCol() { 069 return myLocationCol; 070 } 071 072 public Integer getLocationLine() { 073 return myLocationLine; 074 } 075 076 public String getLocationString() { 077 return myLocationString; 078 } 079 080 public String getMessage() { 081 return myMessage; 082 } 083 084 public String getMessageId() { 085 return myMessageId; 086 } 087 088 public ResultSeverityEnum getSeverity() { 089 return mySeverity; 090 } 091 092 @Override 093 public int hashCode() { 094 HashCodeBuilder b = new HashCodeBuilder(); 095 b.append(myLocationCol); 096 b.append(myLocationCol); 097 b.append(myLocationString); 098 b.append(myMessage); 099 b.append(mySeverity); 100 b.append(mySliceMessages); 101 return b.toHashCode(); 102 } 103 104 public void setLocationCol(Integer theLocationCol) { 105 myLocationCol = theLocationCol; 106 } 107 108 public void setLocationLine(Integer theLocationLine) { 109 myLocationLine = theLocationLine; 110 } 111 112 public void setLocationString(String theLocationString) { 113 myLocationString = theLocationString; 114 } 115 116 public void setMessage(String theMessage) { 117 myMessage = theMessage; 118 } 119 120 public void setMessageId(String messageId) { 121 myMessageId = messageId; 122 } 123 124 public void setSeverity(ResultSeverityEnum theSeverity) { 125 mySeverity = theSeverity; 126 } 127 128 @Override 129 public String toString() { 130 ToStringBuilder b = new ToStringBuilder(this, ToStringStyle.SHORT_PREFIX_STYLE); 131 if (myLocationCol != null || myLocationLine != null) { 132 b.append("col", myLocationCol); 133 b.append("row", myLocationLine); 134 } 135 if (myLocationString != null) { 136 b.append("locationString", myLocationString); 137 } 138 b.append("message", myMessage); 139 if (myMessageId != null) { 140 b.append(myMessageId); 141 } 142 if (mySeverity != null) { 143 b.append("severity", mySeverity.getCode()); 144 } 145 if (mySliceMessages != null) { 146 b.append("sliceMessages", mySliceMessages); 147 } 148 return b.toString(); 149 } 150 151 public void setSliceMessages(List<String> theSliceMessages) { 152 mySliceMessages = theSliceMessages; 153 } 154 155 public List<String> getSliceMessages() { 156 return mySliceMessages; 157 } 158}