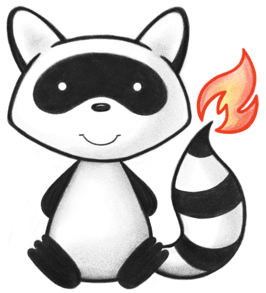
001/* 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.validation.schematron; 021 022import ca.uhn.fhir.context.FhirContext; 023import ca.uhn.fhir.i18n.Msg; 024import ca.uhn.fhir.util.CoverageIgnore; 025import ca.uhn.fhir.validation.FhirValidator; 026import ca.uhn.fhir.validation.IValidatorModule; 027 028import java.lang.reflect.Constructor; 029 030public class SchematronProvider { 031 032 private static final String I18N_KEY_NO_PH_WARNING = FhirValidator.class.getName() + ".noPhWarningOnStartup"; 033 private static final org.slf4j.Logger ourLog = org.slf4j.LoggerFactory.getLogger(FhirValidator.class); 034 035 @CoverageIgnore 036 public static boolean isSchematronAvailable(FhirContext theFhirContext) { 037 try { 038 Class.forName("com.helger.schematron.ISchematronResource"); 039 return true; 040 } catch (ClassNotFoundException e) { 041 ourLog.info(theFhirContext.getLocalizer().getMessage(I18N_KEY_NO_PH_WARNING)); 042 return false; 043 } 044 } 045 046 @SuppressWarnings("unchecked") 047 @CoverageIgnore 048 public static Class<? extends IValidatorModule> getSchematronValidatorClass() { 049 try { 050 return (Class<? extends IValidatorModule>) 051 Class.forName("ca.uhn.fhir.validation.schematron.SchematronBaseValidator"); 052 } catch (ClassNotFoundException e) { 053 throw new IllegalStateException(Msg.code(1973) + "Cannot resolve schematron validator ", e); 054 } 055 } 056 057 @CoverageIgnore 058 public static IValidatorModule getSchematronValidatorInstance(FhirContext myContext) { 059 try { 060 Class<? extends IValidatorModule> cls = getSchematronValidatorClass(); 061 Constructor<? extends IValidatorModule> constructor = cls.getConstructor(FhirContext.class); 062 return constructor.newInstance(myContext); 063 } catch (Exception e) { 064 throw new IllegalStateException(Msg.code(1974) + "Cannot construct schematron validator ", e); 065 } 066 } 067}