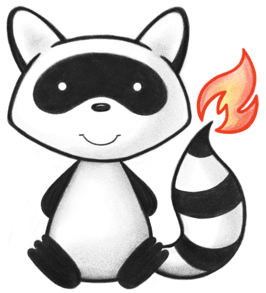
001/* 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package org.hl7.fhir.instance.model.api; 021 022import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 023import ca.uhn.fhir.rest.gclient.DateClientParam; 024import ca.uhn.fhir.rest.gclient.TokenClientParam; 025import ca.uhn.fhir.rest.gclient.UriClientParam; 026 027/** 028 * An IBaseResource that has a FHIR version of DSTU3 or higher 029 */ 030public interface IAnyResource extends IBaseResource { 031 032 String SP_RES_ID = "_id"; 033 /** 034 * Search parameter constant for <b>_id</b> 035 */ 036 @SearchParamDefinition( 037 name = SP_RES_ID, 038 path = "Resource.id", 039 description = "The ID of the resource", 040 type = "token") 041 042 /** 043 * <b>Fluent Client</b> search parameter constant for <b>_id</b> 044 * <p> 045 * Description: <b>the _id of a resource</b><br> 046 * Type: <b>string</b><br> 047 * Path: <b>Resource.id</b><br> 048 * </p> 049 */ 050 TokenClientParam RES_ID = new TokenClientParam(IAnyResource.SP_RES_ID); 051 052 String SP_RES_LAST_UPDATED = "_lastUpdated"; 053 /** 054 * Search parameter constant for <b>_lastUpdated</b> 055 */ 056 @SearchParamDefinition( 057 name = SP_RES_LAST_UPDATED, 058 path = "Resource.meta.lastUpdated", 059 description = "Only return resources which were last updated as specified by the given range", 060 type = "date") 061 062 /** 063 * <b>Fluent Client</b> search parameter constant for <b>_lastUpdated</b> 064 * <p> 065 * Description: <b>The last updated date of a resource</b><br> 066 * Type: <b>date</b><br> 067 * Path: <b>Resource.meta.lastUpdated</b><br> 068 * </p> 069 */ 070 DateClientParam RES_LAST_UPDATED = new DateClientParam(IAnyResource.SP_RES_LAST_UPDATED); 071 072 String SP_RES_TAG = "_tag"; 073 /** 074 * Search parameter constant for <b>_tag</b> 075 */ 076 @SearchParamDefinition( 077 name = SP_RES_TAG, 078 path = "Resource.meta.tag", 079 description = "The tag of the resource", 080 type = "token") 081 082 /** 083 * <b>Fluent Client</b> search parameter constant for <b>_tag</b> 084 * <p> 085 * Description: <b>The tag of a resource</b><br> 086 * Type: <b>token</b><br> 087 * Path: <b>Resource.meta.tag</b><br> 088 * </p> 089 */ 090 TokenClientParam RES_TAG = new TokenClientParam(IAnyResource.SP_RES_TAG); 091 092 String SP_RES_PROFILE = "_profile"; 093 /** 094 * Search parameter constant for <b>_profile</b> 095 */ 096 @SearchParamDefinition( 097 name = SP_RES_PROFILE, 098 path = "Resource.meta.profile", 099 description = "The profile of the resource", 100 type = "uri") 101 102 /** 103 * <b>Fluent Client</b> search parameter constant for <b>_profile</b> 104 * <p> 105 * Description: <b>The profile of a resource</b><br> 106 * Type: <b>uri</b><br> 107 * Path: <b>Resource.meta.profile</b><br> 108 * </p> 109 */ 110 UriClientParam RES_PROFILE = new UriClientParam(IAnyResource.SP_RES_PROFILE); 111 112 String SP_RES_SECURITY = "_security"; 113 /** 114 * Search parameter constant for <b>_security</b> 115 */ 116 @SearchParamDefinition( 117 name = SP_RES_SECURITY, 118 path = "Resource.meta.security", 119 description = "The security of the resource", 120 type = "token") 121 122 /** 123 * <b>Fluent Client</b> search parameter constant for <b>_security</b> 124 * <p> 125 * Description: <b>The security of a resource</b><br> 126 * Type: <b>token</b><br> 127 * Path: <b>Resource.meta.security</b><br> 128 * </p> 129 */ 130 TokenClientParam RES_SECURITY = new TokenClientParam(IAnyResource.SP_RES_SECURITY); 131 132 String getId(); 133 134 IIdType getIdElement(); 135 136 IPrimitiveType<String> getLanguageElement(); 137 138 Object getUserData(String name); 139 140 IAnyResource setId(String theId); 141 142 void setUserData(String name, Object value); 143}