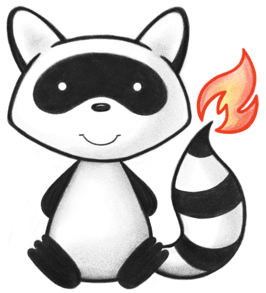
001/* 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package org.hl7.fhir.instance.model.api; 021 022import java.io.Serializable; 023import java.util.List; 024 025/** 026 * This interface is a simple marker for anything which is an HL7 027 * structure of some kind. It is provided mostly to simplify convergence 028 * between the HL7.org structures and the HAPI ones. 029 */ 030public interface IBase extends Serializable { 031 032 boolean isEmpty(); 033 034 /** 035 * Returns <code>true</code> if any comments would be returned by {@link #getFormatCommentsPre()} 036 * or {@link #getFormatCommentsPost()} 037 * 038 * @since 1.5 039 */ 040 boolean hasFormatComment(); 041 042 /** 043 * Returns a list of comments appearing immediately before this element within the serialized 044 * form of the resource. Creates the list if it does not exist, so this method will not return <code>null</code> 045 * 046 * @since 1.5 047 */ 048 List<String> getFormatCommentsPre(); 049 050 /** 051 * Returns a list of comments appearing immediately after this element within the serialized 052 * form of the resource. Creates the list if it does not exist, so this method will not return <code>null</code> 053 * 054 * @since 1.5 055 */ 056 List<String> getFormatCommentsPost(); 057 058 /** 059 * Returns the FHIR type name for the given element, e.g. "Patient" or "unsignedInt" 060 */ 061 default String fhirType() { 062 return null; 063 } 064 065 /** 066 * Retrieves any user suplied data in this element 067 */ 068 Object getUserData(String theName); 069 070 /** 071 * Sets a user supplied data value in this element 072 */ 073 void setUserData(String theName, Object theValue); 074}