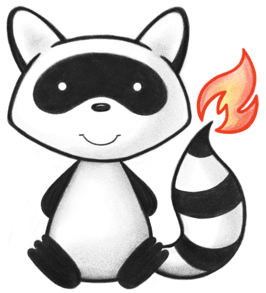
001/* 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package org.hl7.fhir.instance.model.api; 021 022import ca.uhn.fhir.context.FhirVersionEnum; 023import ca.uhn.fhir.model.api.IElement; 024import ca.uhn.fhir.model.api.Include; 025import ca.uhn.fhir.model.api.ResourceMetadataKeyEnum; 026 027import java.util.Arrays; 028import java.util.Collections; 029import java.util.HashSet; 030import java.util.Set; 031 032/** 033 * For now, this is a simple marker interface indicating that a class is a resource type. 034 * There are two concrete types of implementations of this interrface. The first are 035 * HL7.org's Resource structures (e.g. 036 * <code>org.hl7.fhir.instance.model.Patient</code>) and 037 * the second are HAPI's Resource structures, e.g. 038 * <code>ca.uhn.fhir.model.dstu.resource.Patient</code>) 039 */ 040public interface IBaseResource extends IBase, IElement { 041 042 IBaseMetaType getMeta(); 043 044 /** 045 * Include constant for <code>*</code> (return all includes) 046 */ 047 Include INCLUDE_ALL = new Include("*", false).toLocked(); 048 049 /** 050 * Include set containing only {@link #INCLUDE_ALL} 051 */ 052 Set<Include> WILDCARD_ALL_SET = Collections.unmodifiableSet(new HashSet<>(Arrays.asList(INCLUDE_ALL))); 053 054 IIdType getIdElement(); 055 056 IBaseResource setId(String theId); 057 058 IBaseResource setId(IIdType theId); 059 060 FhirVersionEnum getStructureFhirVersionEnum(); 061 062 /** 063 * @return <code>true</code> if this resource has been deleted 064 */ 065 default boolean isDeleted() { 066 return ResourceMetadataKeyEnum.DELETED_AT.get(this) != null; 067 } 068}