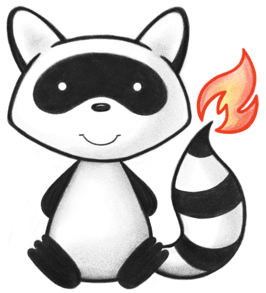
001/* 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package org.hl7.fhir.instance.model.api; 021 022/** 023 * Base interface for ID datatype. 024 * 025 * <p> 026 * <b>Concrete Implementations:</b> This interface is often returned and/or accepted by methods in HAPI's API 027 * where either {@link ca.uhn.fhir.model.primitive.IdDt} (the HAPI structure ID type) or 028 * <code>org.hl7.fhir.instance.model.IdType</code> (the RI structure ID type) will be used, depending on 029 * which version of the strctures your application is using. 030 * </p> 031 */ 032public interface IIdType extends IPrimitiveType<String> { 033 034 void applyTo(IBaseResource theResource); 035 036 /** 037 * Returns the server base URL if this ID contains one. For example, the base URL is 038 * the 'http://example.com/fhir' in the following ID: <code>http://example.com/fhir/Patient/123/_history/55</code> 039 */ 040 String getBaseUrl(); 041 042 /** 043 * Returns only the logical ID part of this ID. For example, given the ID 044 * "http://example,.com/fhir/Patient/123/_history/456", this method would 045 * return "123". 046 */ 047 String getIdPart(); 048 049 /** 050 * Returns the ID part of this ID (e.g. in the ID http://example.com/Patient/123/_history/456 this would be the 051 * part "123") parsed as a {@link Long}. 052 * 053 * @throws NumberFormatException If the value can't be parsed as a long 054 */ 055 Long getIdPartAsLong(); 056 057 String getResourceType(); 058 059 /** 060 * Returns the value of this ID. Note that this value may be a fully qualified URL, a relative/partial URL, or a simple ID. Use {@link #getIdPart()} to get just the ID portion. 061 * 062 * @see #getIdPart() 063 */ 064 @Override 065 String getValue(); 066 067 String getVersionIdPart(); 068 069 /** 070 * Returns the version ID part of this ID (e.g. in the ID http://example.com/Patient/123/_history/456 this would be the 071 * part "456") parsed as a {@link Long}. 072 * 073 * @throws NumberFormatException If the value can't be parsed as a long 074 */ 075 Long getVersionIdPartAsLong(); 076 077 boolean hasBaseUrl(); 078 079 /** 080 * Returns <code>true</code> if this ID contains an actual ID part. For example, the ID part is 081 * the '123' in the following ID: <code>http://example.com/fhir/Patient/123/_history/55</code> 082 */ 083 boolean hasIdPart(); 084 085 boolean hasResourceType(); 086 087 boolean hasVersionIdPart(); 088 089 /** 090 * Returns <code>true</code> if this ID contains an absolute URL (in other words, a URL starting with "http://" or "https://" 091 */ 092 boolean isAbsolute(); 093 094 @Override 095 boolean isEmpty(); 096 097 /** 098 * Returns <code>true</code> if the {@link #getIdPart() ID part of this object} is valid according to the FHIR rules for valid IDs. 099 * <p> 100 * The FHIR specification states: 101 * <code>Any combination of upper or lower case ASCII letters ('A'..'Z', and 'a'..'z', numerals ('0'..'9'), '-' and '.', with a length limit of 64 characters. (This might be an integer, an un-prefixed OID, UUID or any other identifier pattern that meets these constraints.) regex: [A-Za-z0-9\-\.]{1,64}</code> 102 * </p> 103 */ 104 boolean isIdPartValid(); 105 106 /** 107 * Returns <code>true</code> if the {@link #getIdPart() ID part of this object} contains 108 * only numbers 109 */ 110 boolean isIdPartValidLong(); 111 112 /** 113 * Returns <code>true</code> if the ID is a local reference (in other words, it begins with the '#' character) 114 */ 115 boolean isLocal(); 116 117 /** 118 * Returns <code>true</code> if the {@link #getVersionIdPart() version ID part of this object} contains 119 * only numbers 120 */ 121 boolean isVersionIdPartValidLong(); 122 123 @Override 124 IIdType setValue(String theString); 125 126 IIdType toUnqualified(); 127 128 IIdType toUnqualifiedVersionless(); 129 130 IIdType toVersionless(); 131 132 /** 133 * Returns a copy of this object, but with a different {@link #getResourceType() resource type} 134 * (or if this object does not have a resource type currently, returns a copy of this object with 135 * the given resource type). 136 * <p> 137 * Note that if this object represents a local reference (e.g. <code>#foo</code>) or 138 * a URN (e.g. <code>urn:oid:1.2.3.4</code>) this method will simply return a copy 139 * of this object with no modifications. 140 * </p> 141 */ 142 IIdType withResourceType(String theResName); 143 144 /** 145 * Returns a copy of this object, but with a different {@link #getResourceType() resource type} 146 * and {@link #getBaseUrl() base URL} 147 * (or if this object does not have a resource type currently, returns a copy of this object with 148 * the given server base and resource type). 149 * <p> 150 * Note that if this object represents a local reference (e.g. <code>#foo</code>) or 151 * a URN (e.g. <code>urn:oid:1.2.3.4</code>) this method will simply return a copy 152 * of this object with no modifications. 153 * </p> 154 */ 155 IIdType withServerBase(String theServerBase, String theResourceName); 156 157 /** 158 * Returns a copy of this object, but with a different {@link #getVersionIdPart() version ID} 159 * (or if this object does not have a resource type currently, returns a copy of this object with 160 * the given version). 161 * <p> 162 * Note that if this object represents a local reference (e.g. <code>#foo</code>) or 163 * a URN (e.g. <code>urn:oid:1.2.3.4</code>) this method will simply return a copy 164 * of this object with no modifications. 165 * </p> 166 */ 167 IIdType withVersion(String theVersion); 168 169 /** 170 * Sets the value of this ID by combining all of the individual parts. 171 * <p> 172 * <b>Required parameters:</b> The following rules apply to the parameters of this method (in this case, populated means 173 * a non-empty string and not populated means <code>null</code> or an empty string) 174 * </p> 175 * <ul> 176 * <li>All values may be not populated</li> 177 * <li>If <b>theVersionIdPart</b> is populated, <b>theResourceType</b> and <b>theIdPart</b> must be populated</li> 178 * <li>If <b>theBaseUrl</b> is populated and <b>theIdPart</b> is populated, <b>theResourceType</b> must be populated</li> 179 * </ul> 180 * 181 * @return Returns a reference to <code>this</code> for easy method chaining 182 */ 183 IIdType setParts(String theBaseUrl, String theResourceType, String theIdPart, String theVersionIdPart); 184}