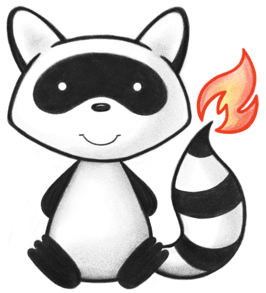
001/*- 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.interceptor.api; 021 022import ca.uhn.fhir.interceptor.executor.SupplierFilterHookWrapper; 023import com.google.common.collect.Lists; 024import org.apache.commons.lang3.Validate; 025 026import java.util.List; 027import java.util.function.Supplier; 028 029public interface IBaseInterceptorBroadcaster<POINTCUT extends IPointcut> { 030 031 /** 032 * Invoke registered interceptor hook methods for the given Pointcut. 033 * 034 * @return Returns <code>false</code> if any of the invoked hook methods returned 035 * <code>false</code>, and returns <code>true</code> otherwise. 036 */ 037 boolean callHooks(POINTCUT thePointcut, HookParams theParams); 038 039 /** 040 * A supplier-based callHooks() for lazy construction of the HookParameters. 041 * @return false if any hook methods return false, return true otherwise. 042 */ 043 default boolean ifHasCallHooks(POINTCUT thePointcut, Supplier<HookParams> theParamsSupplier) { 044 if (hasHooks(thePointcut)) { 045 HookParams params = theParamsSupplier.get(); 046 return callHooks(thePointcut, params); 047 } 048 return true; // callHooks returns true when none present 049 } 050 051 /** 052 * Invoke registered interceptor hook methods for the given Pointcut. This method 053 * should only be called for pointcuts that return a type other than 054 * <code>void</code> or <code>boolean</code> 055 * 056 * @return Returns the object returned by the first hook method that did not return <code>null</code> 057 */ 058 Object callHooksAndReturnObject(POINTCUT thePointcut, HookParams theParams); 059 060 /** 061 * A supplier-based version of callHooksAndReturnObject for lazy construction of the params. 062 * 063 * @return Returns the object returned by the first hook method that did not return <code>null</code> or <code>null</code> 064 */ 065 default Object ifHasCallHooksAndReturnObject(POINTCUT thePointcut, Supplier<HookParams> theParams) { 066 if (hasHooks(thePointcut)) { 067 HookParams params = theParams.get(); 068 return callHooksAndReturnObject(thePointcut, params); 069 } 070 return null; 071 } 072 073 default void runWithFilterHooks(POINTCUT thePointcut, HookParams theHookParams, Runnable theRunnable) { 074 runWithFilterHooks(thePointcut, theHookParams, () -> { 075 theRunnable.run(); 076 return null; 077 }); 078 } 079 080 default <T> T runWithFilterHooks(POINTCUT thePointcut, HookParams theHookParams, Supplier<T> theSupplier) { 081 Validate.isTrue( 082 thePointcut.getReturnType() == IInterceptorFilterHook.class, 083 "Only pointcuts that return IInterceptorFilterHook can be used with this method"); 084 085 List<IInvoker> invokers = getInvokersForPointcut(thePointcut); 086 087 Supplier<T> supplier = theSupplier; 088 089 // Build a linked list of wrappers. 090 // We traverse the invokers in reverse order because the first wrapper will be called last in sequence. 091 for (IInvoker nextInvoker : Lists.reverse(invokers)) { 092 IInterceptorFilterHook filter = (IInterceptorFilterHook) nextInvoker.invoke(theHookParams); 093 supplier = new SupplierFilterHookWrapper<>(supplier, filter, nextInvoker::getHookDescription); 094 } 095 096 return supplier.get(); 097 } 098 099 /** 100 * Does this broadcaster have any hooks for the given pointcut? 101 * 102 * @param thePointcut The poointcut 103 * @return Does this broadcaster have any hooks for the given pointcut? 104 * @since 4.0.0 105 */ 106 boolean hasHooks(POINTCUT thePointcut); 107 108 List<IInvoker> getInvokersForPointcut(POINTCUT thePointcut); 109 110 interface IInvoker extends Comparable<IInvoker> { 111 112 Object invoke(HookParams theParams); 113 114 int getOrder(); 115 116 Object getInterceptor(); 117 118 default String getHookDescription() { 119 return toString(); 120 } 121 } 122 /** 123 * A filter hook is a hook that wraps a system call, and 124 * allows a hook to run code before and after the supplied function. 125 * Filter hooks must call the runnable passed in themselves, similar to Java Servlet Filters. 126 * 127 * @see IInterceptorBroadcaster#runWithFilterHooks(IPointcut, HookParams, Supplier) 128 */ 129 @FunctionalInterface 130 interface IInterceptorFilterHook { 131 void wrapCall(Runnable theRunnable); 132 } 133}