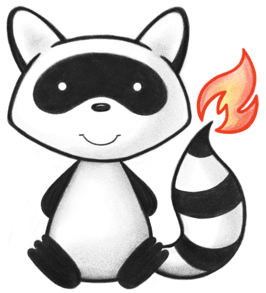
001/* 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.model.api.annotation; 021 022import ca.uhn.fhir.model.api.IElement; 023 024import java.lang.annotation.ElementType; 025import java.lang.annotation.Retention; 026import java.lang.annotation.RetentionPolicy; 027import java.lang.annotation.Target; 028 029/** 030 * Field annotation for fields within resource and datatype definitions, indicating 031 * a child of that type. 032 */ 033@Retention(RetentionPolicy.RUNTIME) 034@Target(value = {ElementType.FIELD}) 035public @interface Child { 036 037 /** 038 * Constant value to supply for {@link #order()} when the order is defined 039 * elsewhere 040 */ 041 int ORDER_UNKNOWN = -1; 042 043 /** 044 * Constant value to supply for {@link #max()} to indicate '*' (no maximum) 045 */ 046 int MAX_UNLIMITED = -1; 047 048 /** 049 * Constant value to supply for {@link #order()} to indicate that this child should replace the 050 * entry in the superclass with the same name (and take its {@link Child#order() order} value 051 * in the process). This is useful if you wish to redefine an existing field in a resource/type 052 * definition in order to constrain/extend it. 053 */ 054 int REPLACE_PARENT = -2; 055 056 /** 057 * The name of this field, as it will appear in serialized versions of the message 058 */ 059 String name(); 060 061 /** 062 * The order in which this field comes within its parent. The first field should have a 063 * value of 0, the second a value of 1, etc. 064 */ 065 int order() default ORDER_UNKNOWN; 066 067 /** 068 * The minimum number of repetitions allowed for this child 069 */ 070 int min() default 0; 071 072 /** 073 * The maximum number of repetitions allowed for this child. Should be 074 * set to {@link #MAX_UNLIMITED} if there is no limit to the number of 075 * repetitions. 076 */ 077 int max() default 1; 078 079 /** 080 * Lists the allowable types for this field, if the field supports multiple 081 * types (otherwise does not need to be populated). 082 * <p> 083 * For example, if this field supports either DateTimeDt or BooleanDt types, 084 * those two classes should be supplied here. 085 * </p> 086 */ 087 Class<? extends IElement>[] type() default {}; 088 089 // Not implemented 090 // /** 091 // * This value is used when extending a built-in model class and defining a 092 // * field to replace a field within the built-in class. For example, the {@link Patient} 093 // * resource has a {@link Patient#getName() name} field, but if you wanted to extend Patient and 094 // * provide your own implementation of {@link HumanNameDt} (most likely your own subclass of 095 // * HumanNameDt which adds extensions of your choosing) you could do that using a replacement field. 096 // */ 097 // String replaces() default ""; 098 099 /** 100 * Is this element a modifier? 101 */ 102 boolean modifier() default false; 103 104 /** 105 * Should this element be included in the summary view 106 */ 107 boolean summary() default false; 108}