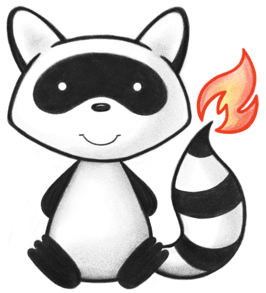
001/*- 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.narrative2; 021 022import org.apache.commons.lang3.builder.ToStringBuilder; 023import org.apache.commons.lang3.builder.ToStringStyle; 024import org.hl7.fhir.instance.model.api.IBase; 025 026import java.util.Collections; 027import java.util.HashSet; 028import java.util.Set; 029 030public class NarrativeTemplate implements INarrativeTemplate { 031 032 private final Set<String> myAppliesToProfiles = new HashSet<>(); 033 private final Set<String> myAppliesToResourceTypes = new HashSet<>(); 034 private final Set<String> myAppliesToDataTypes = new HashSet<>(); 035 private final Set<Class<? extends IBase>> myAppliesToClasses = new HashSet<>(); 036 private final Set<String> myAppliesToFragmentNames = new HashSet<>(); 037 private final Set<String> myAppliesToCode = new HashSet<>(); 038 private String myTemplateFileName; 039 private TemplateTypeEnum myTemplateType = TemplateTypeEnum.THYMELEAF; 040 private String myContextPath; 041 private String myTemplateName; 042 043 @Override 044 public String toString() { 045 return new ToStringBuilder(this, ToStringStyle.SIMPLE_STYLE) 046 .append("name", myTemplateName) 047 .append("fileName", myTemplateFileName) 048 .toString(); 049 } 050 051 public Set<String> getAppliesToDataTypes() { 052 return Collections.unmodifiableSet(myAppliesToDataTypes); 053 } 054 055 public Set<String> getAppliesToFragmentNames() { 056 return Collections.unmodifiableSet(myAppliesToFragmentNames); 057 } 058 059 void addAppliesToFragmentName(String theAppliesToFragmentName) { 060 myAppliesToFragmentNames.add(theAppliesToFragmentName); 061 } 062 063 @Override 064 public String getContextPath() { 065 return myContextPath; 066 } 067 068 public void setContextPath(String theContextPath) { 069 myContextPath = theContextPath; 070 } 071 072 private String getTemplateFileName() { 073 return myTemplateFileName; 074 } 075 076 void setTemplateFileName(String theTemplateFileName) { 077 myTemplateFileName = theTemplateFileName; 078 } 079 080 @Override 081 public Set<String> getAppliesToProfiles() { 082 return Collections.unmodifiableSet(myAppliesToProfiles); 083 } 084 085 @Override 086 public Set<String> getAppliesToCode() { 087 return Collections.unmodifiableSet(myAppliesToCode); 088 } 089 090 void addAppliesToProfile(String theAppliesToProfile) { 091 myAppliesToProfiles.add(theAppliesToProfile); 092 } 093 094 void addAppliesToCode(String theAppliesToCode) { 095 myAppliesToCode.add(theAppliesToCode); 096 } 097 098 @Override 099 public Set<String> getAppliesToResourceTypes() { 100 return Collections.unmodifiableSet(myAppliesToResourceTypes); 101 } 102 103 void addAppliesToResourceType(String theAppliesToResourceType) { 104 myAppliesToResourceTypes.add(theAppliesToResourceType); 105 } 106 107 @Override 108 public Set<Class<? extends IBase>> getAppliesToClasses() { 109 return Collections.unmodifiableSet(myAppliesToClasses); 110 } 111 112 void addAppliesToClass(Class<? extends IBase> theAppliesToClass) { 113 myAppliesToClasses.add(theAppliesToClass); 114 } 115 116 @Override 117 public TemplateTypeEnum getTemplateType() { 118 return myTemplateType; 119 } 120 121 void setTemplateType(TemplateTypeEnum theTemplateType) { 122 myTemplateType = theTemplateType; 123 } 124 125 @Override 126 public String getTemplateName() { 127 return myTemplateName; 128 } 129 130 NarrativeTemplate setTemplateName(String theTemplateName) { 131 myTemplateName = theTemplateName; 132 return this; 133 } 134 135 @Override 136 public String getTemplateText() { 137 return NarrativeTemplateManifest.loadResource(getTemplateFileName()); 138 } 139 140 void addAppliesToDatatype(String theDataType) { 141 myAppliesToDataTypes.add(theDataType); 142 } 143}