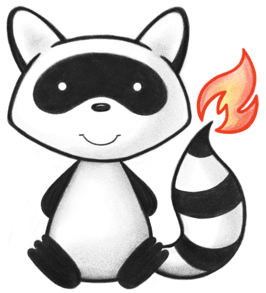
001/* 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.parser; 021 022import ca.uhn.fhir.context.BaseRuntimeChildDefinition; 023import ca.uhn.fhir.context.BaseRuntimeDeclaredChildDefinition; 024import ca.uhn.fhir.context.BaseRuntimeElementCompositeDefinition; 025import ca.uhn.fhir.context.BaseRuntimeElementDefinition; 026import ca.uhn.fhir.context.BaseRuntimeElementDefinition.ChildTypeEnum; 027import ca.uhn.fhir.context.ConfigurationException; 028import ca.uhn.fhir.context.FhirContext; 029import ca.uhn.fhir.context.FhirVersionEnum; 030import ca.uhn.fhir.context.ParserOptions; 031import ca.uhn.fhir.context.RuntimeChildChoiceDefinition; 032import ca.uhn.fhir.context.RuntimeChildContainedResources; 033import ca.uhn.fhir.context.RuntimeChildNarrativeDefinition; 034import ca.uhn.fhir.context.RuntimeResourceDefinition; 035import ca.uhn.fhir.i18n.Msg; 036import ca.uhn.fhir.model.api.IIdentifiableElement; 037import ca.uhn.fhir.model.api.IResource; 038import ca.uhn.fhir.model.api.ISupportsUndeclaredExtensions; 039import ca.uhn.fhir.model.api.ResourceMetadataKeyEnum; 040import ca.uhn.fhir.model.api.Tag; 041import ca.uhn.fhir.model.api.TagList; 042import ca.uhn.fhir.parser.path.EncodeContextPath; 043import ca.uhn.fhir.rest.api.Constants; 044import ca.uhn.fhir.rest.server.exceptions.InternalErrorException; 045import ca.uhn.fhir.util.BundleUtil; 046import ca.uhn.fhir.util.CollectionUtil; 047import ca.uhn.fhir.util.FhirTerser; 048import ca.uhn.fhir.util.MetaUtil; 049import ca.uhn.fhir.util.ResourceUtil; 050import ca.uhn.fhir.util.UrlUtil; 051import com.google.common.base.Charsets; 052import jakarta.annotation.Nullable; 053import org.apache.commons.io.IOUtils; 054import org.apache.commons.io.output.StringBuilderWriter; 055import org.apache.commons.lang3.StringUtils; 056import org.apache.commons.lang3.Validate; 057import org.apache.commons.lang3.tuple.Pair; 058import org.hl7.fhir.instance.model.api.IBase; 059import org.hl7.fhir.instance.model.api.IBaseBundle; 060import org.hl7.fhir.instance.model.api.IBaseCoding; 061import org.hl7.fhir.instance.model.api.IBaseElement; 062import org.hl7.fhir.instance.model.api.IBaseHasExtensions; 063import org.hl7.fhir.instance.model.api.IBaseHasModifierExtensions; 064import org.hl7.fhir.instance.model.api.IBaseMetaType; 065import org.hl7.fhir.instance.model.api.IBaseReference; 066import org.hl7.fhir.instance.model.api.IBaseResource; 067import org.hl7.fhir.instance.model.api.IIdType; 068import org.hl7.fhir.instance.model.api.IPrimitiveType; 069 070import java.io.IOException; 071import java.io.InputStream; 072import java.io.InputStreamReader; 073import java.io.Reader; 074import java.io.StringReader; 075import java.io.Writer; 076import java.lang.reflect.Modifier; 077import java.util.ArrayList; 078import java.util.Arrays; 079import java.util.Collection; 080import java.util.Collections; 081import java.util.HashMap; 082import java.util.HashSet; 083import java.util.List; 084import java.util.Map; 085import java.util.Objects; 086import java.util.Set; 087import java.util.stream.Collectors; 088 089import static org.apache.commons.lang3.StringUtils.isBlank; 090import static org.apache.commons.lang3.StringUtils.isNotBlank; 091import static org.apache.commons.lang3.StringUtils.startsWith; 092 093@SuppressWarnings("WeakerAccess") 094public abstract class BaseParser implements IParser { 095 096 /** 097 * Any resources that were created by the parser (i.e. by parsing a serialized resource) will have 098 * a {@link IBaseResource#getUserData(String) user data} property with this key. 099 * 100 * @since 5.0.0 101 */ 102 public static final String RESOURCE_CREATED_BY_PARSER = 103 BaseParser.class.getName() + "_" + "RESOURCE_CREATED_BY_PARSER"; 104 105 private static final org.slf4j.Logger ourLog = org.slf4j.LoggerFactory.getLogger(BaseParser.class); 106 107 private static final Set<String> notEncodeForContainedResource = 108 new HashSet<>(Arrays.asList("security", "versionId", "lastUpdated")); 109 110 private boolean myEncodeElementsAppliesToChildResourcesOnly; 111 private final FhirContext myContext; 112 private Collection<String> myDontEncodeElements; 113 private Collection<String> myEncodeElements; 114 private IIdType myEncodeForceResourceId; 115 private IParserErrorHandler myErrorHandler; 116 private boolean myOmitResourceId; 117 private List<Class<? extends IBaseResource>> myPreferTypes; 118 private String myServerBaseUrl; 119 private Boolean myStripVersionsFromReferences; 120 private Boolean myOverrideResourceIdWithBundleEntryFullUrl; 121 private boolean mySummaryMode; 122 private boolean mySuppressNarratives; 123 private Set<String> myDontStripVersionsFromReferencesAtPaths; 124 /** 125 * Constructor 126 */ 127 public BaseParser(FhirContext theContext, IParserErrorHandler theParserErrorHandler) { 128 myContext = theContext; 129 myErrorHandler = theParserErrorHandler; 130 } 131 132 protected FhirContext getContext() { 133 return myContext; 134 } 135 136 @Override 137 public IParser setDontEncodeElements(Collection<String> theDontEncodeElements) { 138 myDontEncodeElements = theDontEncodeElements; 139 return this; 140 } 141 142 @Override 143 public IParser setEncodeElements(Set<String> theEncodeElements) { 144 myEncodeElements = theEncodeElements; 145 return this; 146 } 147 148 protected Iterable<CompositeChildElement> compositeChildIterator( 149 IBase theCompositeElement, 150 final boolean theContainedResource, 151 final CompositeChildElement theParent, 152 EncodeContext theEncodeContext) { 153 BaseRuntimeElementCompositeDefinition<?> elementDef = (BaseRuntimeElementCompositeDefinition<?>) 154 myContext.getElementDefinition(theCompositeElement.getClass()); 155 return theEncodeContext 156 .getCompositeChildrenCache() 157 .computeIfAbsent(new Key(elementDef, theContainedResource, theParent, theEncodeContext), (k) -> { 158 final List<BaseRuntimeChildDefinition> children = elementDef.getChildrenAndExtension(); 159 final List<CompositeChildElement> result = new ArrayList<>(children.size()); 160 161 for (final BaseRuntimeChildDefinition child : children) { 162 CompositeChildElement myNext = new CompositeChildElement(theParent, child, theEncodeContext); 163 164 /* 165 * There are lots of reasons we might skip encoding a particular child 166 */ 167 if (myNext.getDef().getElementName().equals("id")) { 168 continue; 169 } else if (!myNext.shouldBeEncoded(theContainedResource)) { 170 continue; 171 } else if (myNext.getDef() instanceof RuntimeChildNarrativeDefinition) { 172 if (isSuppressNarratives() || isSummaryMode()) { 173 continue; 174 } 175 } else if (myNext.getDef() instanceof RuntimeChildContainedResources) { 176 if (theContainedResource) { 177 continue; 178 } 179 } 180 result.add(myNext); 181 } 182 return result; 183 }); 184 } 185 186 private String determineReferenceText( 187 IBaseReference theRef, 188 CompositeChildElement theCompositeChildElement, 189 IBaseResource theResource, 190 EncodeContext theContext) { 191 IIdType ref = theRef.getReferenceElement(); 192 if (isBlank(ref.getIdPart())) { 193 String reference = ref.getValue(); 194 if (theRef.getResource() != null) { 195 IIdType containedId = theContext.getContainedResources().getResourceId(theRef.getResource()); 196 if (containedId != null && !containedId.isEmpty()) { 197 if (containedId.isLocal()) { 198 reference = containedId.getValue(); 199 } else { 200 reference = "#" + containedId.getValue(); 201 } 202 } else { 203 IIdType refId = theRef.getResource().getIdElement(); 204 if (refId != null) { 205 if (refId.hasIdPart()) { 206 if (refId.getValue().startsWith("urn:")) { 207 reference = refId.getValue(); 208 } else { 209 if (!refId.hasResourceType()) { 210 refId = refId.withResourceType(myContext 211 .getResourceDefinition(theRef.getResource()) 212 .getName()); 213 } 214 if (isStripVersionsFromReferences(theCompositeChildElement, theResource)) { 215 reference = refId.toVersionless().getValue(); 216 } else { 217 reference = refId.getValue(); 218 } 219 } 220 } 221 } 222 } 223 } 224 return reference; 225 } 226 if (!ref.hasResourceType() && !ref.isLocal() && theRef.getResource() != null) { 227 ref = ref.withResourceType( 228 myContext.getResourceDefinition(theRef.getResource()).getName()); 229 } 230 if (isNotBlank(myServerBaseUrl) && StringUtils.equals(myServerBaseUrl, ref.getBaseUrl())) { 231 if (isStripVersionsFromReferences(theCompositeChildElement, theResource)) { 232 return ref.toUnqualifiedVersionless().getValue(); 233 } 234 return ref.toUnqualified().getValue(); 235 } 236 if (isStripVersionsFromReferences(theCompositeChildElement, theResource)) { 237 return ref.toVersionless().getValue(); 238 } 239 return ref.getValue(); 240 } 241 242 protected abstract void doEncodeResourceToWriter( 243 IBaseResource theResource, Writer theWriter, EncodeContext theEncodeContext) 244 throws IOException, DataFormatException; 245 246 protected void doEncodeToWriter(IBase theElement, Writer theWriter, EncodeContext theEncodeContext) 247 throws IOException, DataFormatException { 248 throw new InternalErrorException(Msg.code(2363) + "This parser does not support encoding non-resource values"); 249 } 250 251 protected abstract <T extends IBaseResource> T doParseResource(Class<T> theResourceType, Reader theReader) 252 throws DataFormatException; 253 254 @Override 255 public String encodeResourceToString(IBaseResource theResource) throws DataFormatException { 256 Writer stringWriter = new StringBuilderWriter(); 257 try { 258 encodeResourceToWriter(theResource, stringWriter); 259 } catch (IOException e) { 260 throw new Error( 261 Msg.code(1828) + "Encountered IOException during write to string - This should not happen!", e); 262 } 263 return stringWriter.toString(); 264 } 265 266 @Override 267 public final void encodeResourceToWriter(IBaseResource theResource, Writer theWriter) 268 throws IOException, DataFormatException { 269 EncodeContext encodeContext = 270 new EncodeContext(this, myContext.getParserOptions(), new FhirTerser.ContainedResources()); 271 encodeResourceToWriter(theResource, theWriter, encodeContext); 272 } 273 274 @Override 275 public String encodeToString(IBase theElement) throws DataFormatException { 276 Writer stringWriter = new StringBuilderWriter(); 277 try { 278 encodeToWriter(theElement, stringWriter); 279 } catch (IOException e) { 280 throw new Error( 281 Msg.code(2364) + "Encountered IOException during write to string - This should not happen!", e); 282 } 283 return stringWriter.toString(); 284 } 285 286 @Override 287 public void encodeToWriter(IBase theElement, Writer theWriter) throws DataFormatException, IOException { 288 if (theElement instanceof IBaseResource) { 289 encodeResourceToWriter((IBaseResource) theElement, theWriter); 290 } else if (theElement instanceof IPrimitiveType) { 291 theWriter.write(((IPrimitiveType<?>) theElement).getValueAsString()); 292 } else { 293 EncodeContext encodeContext = 294 new EncodeContext(this, myContext.getParserOptions(), new FhirTerser.ContainedResources()); 295 encodeToWriter(theElement, theWriter, encodeContext); 296 } 297 } 298 299 @Override 300 public void parseInto(Reader theSource, IBase theTarget) throws IOException { 301 if (theTarget instanceof IPrimitiveType) { 302 ((IPrimitiveType<?>) theTarget).setValueAsString(IOUtils.toString(theSource)); 303 } else { 304 doParseIntoComplexStructure(theSource, theTarget); 305 } 306 } 307 308 protected void doParseIntoComplexStructure(Reader theSource, IBase theTarget) { 309 throw new InternalErrorException( 310 Msg.code(2633) + "This " + getEncoding() + " parser does not support parsing non-resource values"); 311 } 312 313 protected void encodeResourceToWriter(IBaseResource theResource, Writer theWriter, EncodeContext theEncodeContext) 314 throws IOException { 315 Validate.notNull(theResource, "theResource can not be null"); 316 Validate.notNull(theWriter, "theWriter can not be null"); 317 Validate.notNull(theEncodeContext, "theEncodeContext can not be null"); 318 319 if (theResource.getStructureFhirVersionEnum() != myContext.getVersion().getVersion()) { 320 throw new IllegalArgumentException(Msg.code(1829) + "This parser is for FHIR version " 321 + myContext.getVersion().getVersion() + " - Can not encode a structure for version " 322 + theResource.getStructureFhirVersionEnum()); 323 } 324 325 String resourceName = 326 myContext.getElementDefinition(theResource.getClass()).getName(); 327 theEncodeContext.pushPath(resourceName, true); 328 329 doEncodeResourceToWriter(theResource, theWriter, theEncodeContext); 330 331 theEncodeContext.popPath(); 332 } 333 334 protected void encodeToWriter(IBase theElement, Writer theWriter, EncodeContext theEncodeContext) 335 throws IOException { 336 Validate.notNull(theElement, "theElement can not be null"); 337 Validate.notNull(theWriter, "theWriter can not be null"); 338 Validate.notNull(theEncodeContext, "theEncodeContext can not be null"); 339 340 BaseRuntimeElementDefinition<?> def = myContext.getElementDefinition(theElement.getClass()); 341 String elementName = def.getName(); 342 theEncodeContext.pushPath(elementName, true); 343 344 doEncodeToWriter(theElement, theWriter, theEncodeContext); 345 346 theEncodeContext.popPath(); 347 } 348 349 private void filterCodingsWithNoCodeOrSystem(List<? extends IBaseCoding> tagList) { 350 for (int i = 0; i < tagList.size(); i++) { 351 if (isBlank(tagList.get(i).getCode()) && isBlank(tagList.get(i).getSystem())) { 352 tagList.remove(i); 353 i--; 354 } 355 } 356 } 357 358 protected IIdType fixContainedResourceId(String theValue) { 359 IIdType retVal = (IIdType) myContext.getElementDefinition("id").newInstance(); 360 if (StringUtils.isNotBlank(theValue) && theValue.charAt(0) == '#') { 361 retVal.setValue(theValue.substring(1)); 362 } else { 363 retVal.setValue(theValue); 364 } 365 return retVal; 366 } 367 368 @SuppressWarnings("unchecked") 369 ChildNameAndDef getChildNameAndDef(BaseRuntimeChildDefinition theChild, IBase theValue) { 370 Class<? extends IBase> type = theValue.getClass(); 371 String childName = theChild.getChildNameByDatatype(type); 372 BaseRuntimeElementDefinition<?> childDef = theChild.getChildElementDefinitionByDatatype(type); 373 if (childDef == null) { 374 // if (theValue instanceof IBaseExtension) { 375 // return null; 376 // } 377 378 /* 379 * For RI structures Enumeration class, this replaces the child def 380 * with the "code" one. This is messy, and presumably there is a better 381 * way.. 382 */ 383 BaseRuntimeElementDefinition<?> elementDef = myContext.getElementDefinition(type); 384 if (elementDef.getName().equals("code")) { 385 Class<? extends IBase> type2 = 386 myContext.getElementDefinition("code").getImplementingClass(); 387 childDef = theChild.getChildElementDefinitionByDatatype(type2); 388 childName = theChild.getChildNameByDatatype(type2); 389 } 390 391 // See possibly the user has extended a built-in type without 392 // declaring it anywhere, as in XmlParserDstu3Test#testEncodeUndeclaredBlock 393 if (childDef == null) { 394 Class<?> nextSuperType = theValue.getClass(); 395 while (IBase.class.isAssignableFrom(nextSuperType) && childDef == null) { 396 if (Modifier.isAbstract(nextSuperType.getModifiers()) == false) { 397 BaseRuntimeElementDefinition<?> def = 398 myContext.getElementDefinition((Class<? extends IBase>) nextSuperType); 399 Class<?> nextChildType = def.getImplementingClass(); 400 childDef = theChild.getChildElementDefinitionByDatatype((Class<? extends IBase>) nextChildType); 401 childName = theChild.getChildNameByDatatype((Class<? extends IBase>) nextChildType); 402 } 403 nextSuperType = nextSuperType.getSuperclass(); 404 } 405 } 406 407 if (childDef == null) { 408 throwExceptionForUnknownChildType(theChild, type); 409 } 410 } 411 412 return new ChildNameAndDef(childName, childDef); 413 } 414 415 protected String getCompositeElementId(IBase theElement) { 416 String elementId = null; 417 if (!(theElement instanceof IBaseResource)) { 418 if (theElement instanceof IBaseElement) { 419 elementId = ((IBaseElement) theElement).getId(); 420 } else if (theElement instanceof IIdentifiableElement) { 421 elementId = ((IIdentifiableElement) theElement).getElementSpecificId(); 422 } 423 } 424 return elementId; 425 } 426 427 @Override 428 public Set<String> getDontStripVersionsFromReferencesAtPaths() { 429 return myDontStripVersionsFromReferencesAtPaths; 430 } 431 432 @Override 433 public IIdType getEncodeForceResourceId() { 434 return myEncodeForceResourceId; 435 } 436 437 @Override 438 public BaseParser setEncodeForceResourceId(IIdType theEncodeForceResourceId) { 439 myEncodeForceResourceId = theEncodeForceResourceId; 440 return this; 441 } 442 443 protected IParserErrorHandler getErrorHandler() { 444 return myErrorHandler; 445 } 446 447 protected List<Map.Entry<ResourceMetadataKeyEnum<?>, Object>> getExtensionMetadataKeys(IResource resource) { 448 List<Map.Entry<ResourceMetadataKeyEnum<?>, Object>> extensionMetadataKeys = new ArrayList<>(); 449 for (Map.Entry<ResourceMetadataKeyEnum<?>, Object> entry : 450 resource.getResourceMetadata().entrySet()) { 451 if (entry.getKey() instanceof ResourceMetadataKeyEnum.ExtensionResourceMetadataKey) { 452 extensionMetadataKeys.add(entry); 453 } 454 } 455 456 return extensionMetadataKeys; 457 } 458 459 protected String getExtensionUrl(final String extensionUrl) { 460 String url = extensionUrl; 461 if (StringUtils.isNotBlank(extensionUrl) && StringUtils.isNotBlank(myServerBaseUrl)) { 462 url = !UrlUtil.isValid(extensionUrl) && extensionUrl.startsWith("/") 463 ? myServerBaseUrl + extensionUrl 464 : extensionUrl; 465 } 466 return url; 467 } 468 469 protected TagList getMetaTagsForEncoding(IResource theIResource, EncodeContext theEncodeContext) { 470 TagList tags = ResourceMetadataKeyEnum.TAG_LIST.get(theIResource); 471 if (shouldAddSubsettedTag(theEncodeContext)) { 472 tags = new TagList(tags); 473 tags.add(new Tag(getSubsettedCodeSystem(), Constants.TAG_SUBSETTED_CODE, subsetDescription())); 474 } 475 476 return tags; 477 } 478 479 @Override 480 public List<Class<? extends IBaseResource>> getPreferTypes() { 481 return myPreferTypes; 482 } 483 484 @Override 485 public void setPreferTypes(List<Class<? extends IBaseResource>> thePreferTypes) { 486 if (thePreferTypes != null) { 487 ArrayList<Class<? extends IBaseResource>> types = new ArrayList<>(); 488 for (Class<? extends IBaseResource> next : thePreferTypes) { 489 if (Modifier.isAbstract(next.getModifiers()) == false) { 490 types.add(next); 491 } 492 } 493 myPreferTypes = Collections.unmodifiableList(types); 494 } else { 495 myPreferTypes = thePreferTypes; 496 } 497 } 498 499 @SuppressWarnings("deprecation") 500 protected <T extends IPrimitiveType<String>> List<T> getProfileTagsForEncoding( 501 IBaseResource theResource, List<T> theProfiles) { 502 switch (myContext.getAddProfileTagWhenEncoding()) { 503 case NEVER: 504 return theProfiles; 505 case ONLY_FOR_CUSTOM: 506 RuntimeResourceDefinition resDef = myContext.getResourceDefinition(theResource); 507 if (resDef.isStandardType()) { 508 return theProfiles; 509 } 510 break; 511 case ALWAYS: 512 break; 513 } 514 515 RuntimeResourceDefinition nextDef = myContext.getResourceDefinition(theResource); 516 String profile = nextDef.getResourceProfile(myServerBaseUrl); 517 if (isNotBlank(profile)) { 518 for (T next : theProfiles) { 519 if (profile.equals(next.getValue())) { 520 return theProfiles; 521 } 522 } 523 524 List<T> newList = new ArrayList<>(theProfiles); 525 526 BaseRuntimeElementDefinition<?> idElement = myContext.getElementDefinition("id"); 527 @SuppressWarnings("unchecked") 528 T newId = (T) idElement.newInstance(); 529 newId.setValue(profile); 530 531 newList.add(newId); 532 return newList; 533 } 534 535 return theProfiles; 536 } 537 538 protected String getServerBaseUrl() { 539 return myServerBaseUrl; 540 } 541 542 @Override 543 public Boolean getStripVersionsFromReferences() { 544 return myStripVersionsFromReferences; 545 } 546 547 /** 548 * If set to <code>true</code> (default is <code>false</code>), narratives will not be included in the encoded 549 * values. 550 * 551 * @deprecated Use {@link #isSuppressNarratives()} 552 */ 553 @Deprecated 554 public boolean getSuppressNarratives() { 555 return mySuppressNarratives; 556 } 557 558 protected boolean isChildContained( 559 BaseRuntimeElementDefinition<?> childDef, boolean theIncludedResource, EncodeContext theContext) { 560 return (childDef.getChildType() == ChildTypeEnum.CONTAINED_RESOURCES 561 || childDef.getChildType() == ChildTypeEnum.CONTAINED_RESOURCE_LIST) 562 && theContext.getContainedResources().isEmpty() == false 563 && theIncludedResource == false; 564 } 565 566 @Override 567 public boolean isEncodeElementsAppliesToChildResourcesOnly() { 568 return myEncodeElementsAppliesToChildResourcesOnly; 569 } 570 571 @Override 572 public void setEncodeElementsAppliesToChildResourcesOnly(boolean theEncodeElementsAppliesToChildResourcesOnly) { 573 myEncodeElementsAppliesToChildResourcesOnly = theEncodeElementsAppliesToChildResourcesOnly; 574 } 575 576 @Override 577 public boolean isOmitResourceId() { 578 return myOmitResourceId; 579 } 580 581 private boolean isOverrideResourceIdWithBundleEntryFullUrl() { 582 Boolean overrideResourceIdWithBundleEntryFullUrl = myOverrideResourceIdWithBundleEntryFullUrl; 583 if (overrideResourceIdWithBundleEntryFullUrl != null) { 584 return overrideResourceIdWithBundleEntryFullUrl; 585 } 586 587 return myContext.getParserOptions().isOverrideResourceIdWithBundleEntryFullUrl(); 588 } 589 590 private boolean isStripVersionsFromReferences( 591 CompositeChildElement theCompositeChildElement, IBaseResource theResource) { 592 593 if (theResource != null) { 594 Set<String> autoVersionReferencesAtPathExtensions = MetaUtil.getAutoVersionReferencesAtPath( 595 theResource.getMeta(), myContext.getResourceType(theResource)); 596 597 if (!autoVersionReferencesAtPathExtensions.isEmpty() 598 && theCompositeChildElement.anyPathMatches(autoVersionReferencesAtPathExtensions)) { 599 return false; 600 } 601 } 602 603 Boolean stripVersionsFromReferences = myStripVersionsFromReferences; 604 if (stripVersionsFromReferences != null) { 605 return stripVersionsFromReferences; 606 } 607 608 if (!myContext.getParserOptions().isStripVersionsFromReferences()) { 609 return false; 610 } 611 612 Set<String> dontStripVersionsFromReferencesAtPaths = getDontStripVersionsFromReferencesAtPaths(); 613 if (dontStripVersionsFromReferencesAtPaths != null 614 && !dontStripVersionsFromReferencesAtPaths.isEmpty() 615 && theCompositeChildElement.anyPathMatches(dontStripVersionsFromReferencesAtPaths)) { 616 return false; 617 } 618 619 dontStripVersionsFromReferencesAtPaths = 620 myContext.getParserOptions().getDontStripVersionsFromReferencesAtPaths(); 621 return dontStripVersionsFromReferencesAtPaths.isEmpty() 622 || !theCompositeChildElement.anyPathMatches(dontStripVersionsFromReferencesAtPaths); 623 } 624 625 @Override 626 public boolean isSummaryMode() { 627 return mySummaryMode; 628 } 629 630 /** 631 * If set to <code>true</code> (default is <code>false</code>), narratives will not be included in the encoded 632 * values. 633 * 634 * @since 1.2 635 */ 636 public boolean isSuppressNarratives() { 637 return mySuppressNarratives; 638 } 639 640 @Override 641 public IBaseResource parseResource(InputStream theInputStream) throws DataFormatException { 642 return parseResource(new InputStreamReader(theInputStream, Charsets.UTF_8)); 643 } 644 645 @Override 646 public <T extends IBaseResource> T parseResource(Class<T> theResourceType, InputStream theInputStream) 647 throws DataFormatException { 648 return parseResource(theResourceType, new InputStreamReader(theInputStream, Constants.CHARSET_UTF8)); 649 } 650 651 @Override 652 public <T extends IBaseResource> T parseResource(Class<T> theResourceType, Reader theReader) 653 throws DataFormatException { 654 655 /* 656 * We do this so that the context can verify that the structure is for 657 * the correct FHIR version 658 */ 659 Reader readerToUse = theReader; 660 if (theResourceType != null) { 661 myContext.getResourceDefinition(theResourceType); 662 if (myContext.isStoreResourceJson()) { 663 readerToUse = new PreserveStringReader(theReader); 664 } 665 } 666 667 // Actually do the parse 668 T retVal = doParseResource(theResourceType, readerToUse); 669 670 if (theResourceType != null && myContext.isStoreResourceJson()) { 671 PreserveStringReader psr = (PreserveStringReader) readerToUse; 672 if (psr.hasString()) { 673 try { 674 ResourceUtil.addRawDataToResource(retVal, getEncoding(), psr.toString()); 675 psr.close(); 676 } catch (IOException ex) { 677 ourLog.warn( 678 "Unable to store raw JSON on resource. This won't affect functionality, but validation will use the resource itself, which may result in different validation results than a $validation operation.", 679 ex); 680 } 681 } 682 } 683 684 RuntimeResourceDefinition def = myContext.getResourceDefinition(retVal); 685 if ("Bundle".equals(def.getName())) { 686 687 if (isOverrideResourceIdWithBundleEntryFullUrl()) { 688 BundleUtil.processEntries(myContext, (IBaseBundle) retVal, t -> { 689 String fullUrl = t.getFullUrl(); 690 if (fullUrl != null) { 691 IBaseResource resource = t.getResource(); 692 if (resource != null) { 693 IIdType resourceId = resource.getIdElement(); 694 if (isBlank(resourceId.getValue())) { 695 resourceId.setValue(fullUrl); 696 } else { 697 if (fullUrl.startsWith("urn:") 698 && fullUrl.length() 699 > resourceId.getIdPart().length() 700 && fullUrl.charAt(fullUrl.length() 701 - resourceId.getIdPart().length() 702 - 1) 703 == ':' 704 && fullUrl.endsWith(resourceId.getIdPart())) { 705 resourceId.setValue(fullUrl); 706 } else { 707 IIdType fullUrlId = myContext.getVersion().newIdType(); 708 fullUrlId.setValue(fullUrl); 709 if (myContext.getVersion().getVersion().isOlderThan(FhirVersionEnum.DSTU3)) { 710 IIdType newId = fullUrlId; 711 if (!newId.hasVersionIdPart() && resourceId.hasVersionIdPart()) { 712 newId = newId.withVersion(resourceId.getVersionIdPart()); 713 } 714 resourceId.setValue(newId.getValue()); 715 } else if (StringUtils.equals(fullUrlId.getIdPart(), resourceId.getIdPart())) { 716 if (fullUrlId.hasBaseUrl()) { 717 IIdType newResourceId = resourceId.withServerBase( 718 fullUrlId.getBaseUrl(), resourceId.getResourceType()); 719 resourceId.setValue(newResourceId.getValue()); 720 } 721 } 722 } 723 } 724 } 725 } 726 }); 727 } 728 } 729 730 return retVal; 731 } 732 733 @SuppressWarnings("cast") 734 @Override 735 public <T extends IBaseResource> T parseResource(Class<T> theResourceType, String theMessageString) { 736 StringReader reader = new StringReader(theMessageString); 737 return parseResource(theResourceType, reader); 738 } 739 740 @Override 741 public IBaseResource parseResource(Reader theReader) throws ConfigurationException, DataFormatException { 742 return parseResource(null, theReader); 743 } 744 745 @Override 746 public IBaseResource parseResource(String theMessageString) throws ConfigurationException, DataFormatException { 747 return parseResource(null, theMessageString); 748 } 749 750 protected List<? extends IBase> preProcessValues( 751 BaseRuntimeChildDefinition theMetaChildUncast, 752 IBaseResource theResource, 753 List<? extends IBase> theValues, 754 CompositeChildElement theCompositeChildElement, 755 EncodeContext theEncodeContext) { 756 if (myContext.getVersion().getVersion().isRi()) { 757 758 /* 759 * If we're encoding the meta tag, we do some massaging of the meta values before 760 * encoding. But if there is no meta element at all, we create one since we're possibly going to be 761 * adding things to it 762 */ 763 if (theValues.isEmpty() && theMetaChildUncast.getElementName().equals("meta")) { 764 BaseRuntimeElementDefinition<?> metaChild = theMetaChildUncast.getChildByName("meta"); 765 if (IBaseMetaType.class.isAssignableFrom(metaChild.getImplementingClass())) { 766 IBaseMetaType newType = (IBaseMetaType) metaChild.newInstance(); 767 theValues = Collections.singletonList(newType); 768 } 769 } 770 771 if (theValues.size() == 1 && theValues.get(0) instanceof IBaseMetaType) { 772 773 IBaseMetaType metaValue = (IBaseMetaType) theValues.get(0); 774 try { 775 metaValue = (IBaseMetaType) 776 metaValue.getClass().getMethod("copy").invoke(metaValue); 777 } catch (Exception e) { 778 throw new InternalErrorException(Msg.code(1830) + "Failed to duplicate meta", e); 779 } 780 781 if (isBlank(metaValue.getVersionId())) { 782 if (theResource.getIdElement().hasVersionIdPart()) { 783 metaValue.setVersionId(theResource.getIdElement().getVersionIdPart()); 784 } 785 } 786 787 filterCodingsWithNoCodeOrSystem(metaValue.getTag()); 788 filterCodingsWithNoCodeOrSystem(metaValue.getSecurity()); 789 790 List<? extends IPrimitiveType<String>> newProfileList = 791 getProfileTagsForEncoding(theResource, metaValue.getProfile()); 792 List<? extends IPrimitiveType<String>> oldProfileList = metaValue.getProfile(); 793 if (oldProfileList != newProfileList) { 794 oldProfileList.clear(); 795 for (IPrimitiveType<String> next : newProfileList) { 796 if (isNotBlank(next.getValue())) { 797 metaValue.addProfile(next.getValue()); 798 } 799 } 800 } 801 802 if (shouldAddSubsettedTag(theEncodeContext)) { 803 IBaseCoding coding = metaValue.addTag(); 804 coding.setCode(Constants.TAG_SUBSETTED_CODE); 805 coding.setSystem(getSubsettedCodeSystem()); 806 coding.setDisplay(subsetDescription()); 807 } 808 809 return Collections.singletonList(metaValue); 810 } 811 } 812 813 @SuppressWarnings("unchecked") 814 List<IBase> retVal = (List<IBase>) theValues; 815 816 for (int i = 0; i < retVal.size(); i++) { 817 IBase next = retVal.get(i); 818 819 /* 820 * If we have automatically contained any resources via 821 * their references, this ensures that we output the new 822 * local reference 823 */ 824 if (next instanceof IBaseReference) { 825 IBaseReference nextRef = (IBaseReference) next; 826 String refText = 827 determineReferenceText(nextRef, theCompositeChildElement, theResource, theEncodeContext); 828 if (!StringUtils.equals(refText, nextRef.getReferenceElement().getValue())) { 829 830 if (retVal == theValues) { 831 retVal = new ArrayList<>(theValues); 832 } 833 IBaseReference newRef = (IBaseReference) 834 myContext.getElementDefinition(nextRef.getClass()).newInstance(); 835 myContext.newTerser().cloneInto(nextRef, newRef, true); 836 newRef.setReference(refText); 837 retVal.set(i, newRef); 838 } 839 } 840 } 841 842 return retVal; 843 } 844 845 private String getSubsettedCodeSystem() { 846 if (myContext.getVersion().getVersion().isEqualOrNewerThan(FhirVersionEnum.R4)) { 847 return Constants.TAG_SUBSETTED_SYSTEM_R4; 848 } else { 849 return Constants.TAG_SUBSETTED_SYSTEM_DSTU3; 850 } 851 } 852 853 @Override 854 public IParser setDontStripVersionsFromReferencesAtPaths(String... thePaths) { 855 if (thePaths == null) { 856 setDontStripVersionsFromReferencesAtPaths((List<String>) null); 857 } else { 858 setDontStripVersionsFromReferencesAtPaths(Arrays.asList(thePaths)); 859 } 860 return this; 861 } 862 863 @SuppressWarnings("unchecked") 864 @Override 865 public IParser setDontStripVersionsFromReferencesAtPaths(Collection<String> thePaths) { 866 if (thePaths == null) { 867 myDontStripVersionsFromReferencesAtPaths = Collections.emptySet(); 868 } else if (thePaths instanceof HashSet) { 869 myDontStripVersionsFromReferencesAtPaths = (Set<String>) ((HashSet<String>) thePaths).clone(); 870 } else { 871 myDontStripVersionsFromReferencesAtPaths = new HashSet<>(thePaths); 872 } 873 return this; 874 } 875 876 @Override 877 public IParser setOmitResourceId(boolean theOmitResourceId) { 878 myOmitResourceId = theOmitResourceId; 879 return this; 880 } 881 882 @Override 883 public IParser setOverrideResourceIdWithBundleEntryFullUrl(Boolean theOverrideResourceIdWithBundleEntryFullUrl) { 884 myOverrideResourceIdWithBundleEntryFullUrl = theOverrideResourceIdWithBundleEntryFullUrl; 885 return this; 886 } 887 888 @Override 889 public IParser setParserErrorHandler(IParserErrorHandler theErrorHandler) { 890 Validate.notNull(theErrorHandler, "theErrorHandler must not be null"); 891 myErrorHandler = theErrorHandler; 892 return this; 893 } 894 895 @Override 896 public IParser setServerBaseUrl(String theUrl) { 897 myServerBaseUrl = isNotBlank(theUrl) ? theUrl : null; 898 return this; 899 } 900 901 @Override 902 public IParser setStripVersionsFromReferences(Boolean theStripVersionsFromReferences) { 903 myStripVersionsFromReferences = theStripVersionsFromReferences; 904 return this; 905 } 906 907 @Override 908 public IParser setSummaryMode(boolean theSummaryMode) { 909 mySummaryMode = theSummaryMode; 910 return this; 911 } 912 913 @Override 914 public IParser setSuppressNarratives(boolean theSuppressNarratives) { 915 mySuppressNarratives = theSuppressNarratives; 916 return this; 917 } 918 919 protected boolean shouldAddSubsettedTag(EncodeContext theEncodeContext) { 920 if (isSummaryMode()) { 921 return true; 922 } 923 if (isSuppressNarratives()) { 924 return true; 925 } 926 if (theEncodeContext.myEncodeElementPaths != null) { 927 if (isEncodeElementsAppliesToChildResourcesOnly() 928 && theEncodeContext.getResourcePath().size() < 2) { 929 return false; 930 } 931 932 String currentResourceName = theEncodeContext 933 .getResourcePath() 934 .get(theEncodeContext.getResourcePath().size() - 1) 935 .getName(); 936 return theEncodeContext.myEncodeElementsAppliesToResourceTypes == null 937 || theEncodeContext.myEncodeElementsAppliesToResourceTypes.contains(currentResourceName); 938 } 939 940 return false; 941 } 942 943 protected boolean shouldEncodeResourceId(IBaseResource theResource, EncodeContext theEncodeContext) { 944 boolean retVal = true; 945 if (isOmitResourceId() && theEncodeContext.getPath().size() == 1) { 946 retVal = false; 947 } else { 948 if (theEncodeContext.myDontEncodeElementPaths != null) { 949 String resourceName = myContext.getResourceType(theResource); 950 if (theEncodeContext.myDontEncodeElementPaths.stream() 951 .anyMatch(t -> t.equalsPath(resourceName + ".id"))) { 952 retVal = false; 953 } else if (theEncodeContext.myDontEncodeElementPaths.stream().anyMatch(t -> t.equalsPath("*.id"))) { 954 retVal = false; 955 } else if (theEncodeContext.getResourcePath().size() == 1 956 && theEncodeContext.myDontEncodeElementPaths.stream().anyMatch(t -> t.equalsPath("id"))) { 957 retVal = false; 958 } 959 } 960 } 961 return retVal; 962 } 963 964 /** 965 * Used for DSTU2 only 966 */ 967 protected boolean shouldEncodeResourceMeta(IResource theResource, EncodeContext theEncodeContext) { 968 return shouldEncodePath(theResource, "meta", theEncodeContext); 969 } 970 971 /** 972 * Used for DSTU2 only 973 */ 974 protected boolean shouldEncodePath(IResource theResource, String thePath, EncodeContext theEncodeContext) { 975 if (theEncodeContext.myDontEncodeElementPaths != null) { 976 String resourceName = myContext.getResourceType(theResource); 977 if (theEncodeContext.myDontEncodeElementPaths.stream() 978 .anyMatch(t -> t.equalsPath(resourceName + "." + thePath))) { 979 return false; 980 } else { 981 return theEncodeContext.myDontEncodeElementPaths.stream().noneMatch(t -> t.equalsPath("*." + thePath)); 982 } 983 } 984 return true; 985 } 986 987 private String subsetDescription() { 988 return "Resource encoded in summary mode"; 989 } 990 991 protected void throwExceptionForUnknownChildType( 992 BaseRuntimeChildDefinition nextChild, Class<? extends IBase> theType) { 993 if (nextChild instanceof BaseRuntimeDeclaredChildDefinition) { 994 StringBuilder b = new StringBuilder(); 995 b.append(nextChild.getElementName()); 996 b.append(" has type "); 997 b.append(theType.getName()); 998 b.append(" but this is not a valid type for this element"); 999 if (nextChild instanceof RuntimeChildChoiceDefinition) { 1000 RuntimeChildChoiceDefinition choice = (RuntimeChildChoiceDefinition) nextChild; 1001 b.append(" - Expected one of: ").append(choice.getValidChildTypes()); 1002 } 1003 throw new DataFormatException(Msg.code(1831) + b); 1004 } 1005 throw new DataFormatException(Msg.code(1832) + nextChild + " has no child of type " + theType); 1006 } 1007 1008 protected boolean shouldEncodeResource(String theName, EncodeContext theEncodeContext) { 1009 if (theEncodeContext.myDontEncodeElementPaths != null) { 1010 for (EncodeContextPath next : theEncodeContext.myDontEncodeElementPaths) { 1011 if (next.equalsPath(theName)) { 1012 return false; 1013 } 1014 } 1015 } 1016 return true; 1017 } 1018 1019 protected void containResourcesInReferences(IBaseResource theResource, EncodeContext theContext) { 1020 1021 /* 1022 * If a UUID is present in Bundle.entry.fullUrl but no value is present 1023 * in Bundle.entry.resource.id, the resource has a discrete identity which 1024 * should be copied into the resource ID. It will not be serialized in 1025 * Resource.id because it's a placeholder/UUID value, but its presence there 1026 * informs the serializer that we don't need to contain this resource. 1027 */ 1028 if (theResource instanceof IBaseBundle) { 1029 List<Pair<String, IBaseResource>> entries = 1030 BundleUtil.getBundleEntryFullUrlsAndResources(getContext(), (IBaseBundle) theResource); 1031 for (Pair<String, IBaseResource> nextEntry : entries) { 1032 String fullUrl = nextEntry.getKey(); 1033 IBaseResource resource = nextEntry.getValue(); 1034 if (startsWith(fullUrl, "urn:")) { 1035 if (resource != null && resource.getIdElement().getValue() == null) { 1036 resource.getIdElement().setValue(fullUrl); 1037 } 1038 } 1039 } 1040 } 1041 1042 theContext.setContainedResources(getContext().newTerser().containResources(theResource)); 1043 } 1044 1045 static class ChildNameAndDef { 1046 1047 private final BaseRuntimeElementDefinition<?> myChildDef; 1048 private final String myChildName; 1049 1050 public ChildNameAndDef(String theChildName, BaseRuntimeElementDefinition<?> theChildDef) { 1051 myChildName = theChildName; 1052 myChildDef = theChildDef; 1053 } 1054 1055 public BaseRuntimeElementDefinition<?> getChildDef() { 1056 return myChildDef; 1057 } 1058 1059 public String getChildName() { 1060 return myChildName; 1061 } 1062 } 1063 1064 /** 1065 * EncodeContext is a shared state object that is passed around the 1066 * encode process 1067 */ 1068 class EncodeContext extends EncodeContextPath { 1069 private final Map<Key, List<BaseParser.CompositeChildElement>> myCompositeChildrenCache = new HashMap<>(); 1070 private final List<EncodeContextPath> myEncodeElementPaths; 1071 private final Set<String> myEncodeElementsAppliesToResourceTypes; 1072 private final List<EncodeContextPath> myDontEncodeElementPaths; 1073 private FhirTerser.ContainedResources myContainedResources; 1074 1075 public EncodeContext( 1076 BaseParser theParser, 1077 ParserOptions theParserOptions, 1078 FhirTerser.ContainedResources theContainedResources) { 1079 Collection<String> encodeElements = theParser.myEncodeElements; 1080 Collection<String> dontEncodeElements = theParser.myDontEncodeElements; 1081 if (isSummaryMode()) { 1082 encodeElements = CollectionUtil.nullSafeUnion( 1083 encodeElements, theParserOptions.getEncodeElementsForSummaryMode()); 1084 dontEncodeElements = CollectionUtil.nullSafeUnion( 1085 dontEncodeElements, theParserOptions.getDontEncodeElementsForSummaryMode()); 1086 } 1087 1088 if (encodeElements == null || encodeElements.isEmpty()) { 1089 myEncodeElementPaths = null; 1090 } else { 1091 myEncodeElementPaths = 1092 encodeElements.stream().map(EncodeContextPath::new).collect(Collectors.toList()); 1093 } 1094 if (dontEncodeElements == null || dontEncodeElements.isEmpty()) { 1095 myDontEncodeElementPaths = null; 1096 } else { 1097 myDontEncodeElementPaths = 1098 dontEncodeElements.stream().map(EncodeContextPath::new).collect(Collectors.toList()); 1099 } 1100 1101 myContainedResources = theContainedResources; 1102 1103 myEncodeElementsAppliesToResourceTypes = 1104 ParserUtil.determineApplicableResourceTypesForTerserPaths(myEncodeElementPaths); 1105 } 1106 1107 private Map<Key, List<BaseParser.CompositeChildElement>> getCompositeChildrenCache() { 1108 return myCompositeChildrenCache; 1109 } 1110 1111 public FhirTerser.ContainedResources getContainedResources() { 1112 return myContainedResources; 1113 } 1114 1115 public void setContainedResources(FhirTerser.ContainedResources theContainedResources) { 1116 myContainedResources = theContainedResources; 1117 } 1118 } 1119 1120 protected class CompositeChildElement { 1121 private final BaseRuntimeChildDefinition myDef; 1122 private final CompositeChildElement myParent; 1123 private final RuntimeResourceDefinition myResDef; 1124 private final EncodeContext myEncodeContext; 1125 1126 public CompositeChildElement( 1127 CompositeChildElement theParent, 1128 @Nullable BaseRuntimeChildDefinition theDef, 1129 EncodeContext theEncodeContext) { 1130 myDef = theDef; 1131 myParent = theParent; 1132 myResDef = null; 1133 myEncodeContext = theEncodeContext; 1134 1135 if (ourLog.isTraceEnabled()) { 1136 if (theParent != null) { 1137 StringBuilder path = theParent.buildPath(); 1138 if (path != null) { 1139 path.append('.'); 1140 if (myDef != null) { 1141 path.append(myDef.getElementName()); 1142 } 1143 ourLog.trace(" * Next path: {}", path); 1144 } 1145 } 1146 } 1147 } 1148 1149 public CompositeChildElement(RuntimeResourceDefinition theResDef, EncodeContext theEncodeContext) { 1150 myResDef = theResDef; 1151 myDef = null; 1152 myParent = null; 1153 myEncodeContext = theEncodeContext; 1154 } 1155 1156 @Override 1157 public String toString() { 1158 return myDef.getElementName(); 1159 } 1160 1161 private void addParent(CompositeChildElement theParent, StringBuilder theB) { 1162 if (theParent != null) { 1163 if (theParent.myResDef != null) { 1164 theB.append(theParent.myResDef.getName()); 1165 return; 1166 } 1167 1168 if (theParent.myParent != null) { 1169 addParent(theParent.myParent, theB); 1170 } 1171 1172 if (theParent.myDef != null) { 1173 if (theB.length() > 0) { 1174 theB.append('.'); 1175 } 1176 theB.append(theParent.myDef.getElementName()); 1177 } 1178 } 1179 } 1180 1181 public boolean anyPathMatches(Set<String> thePaths) { 1182 StringBuilder b = new StringBuilder(); 1183 addParent(this, b); 1184 1185 String path = b.toString(); 1186 return thePaths.contains(path); 1187 } 1188 1189 private StringBuilder buildPath() { 1190 if (myResDef != null) { 1191 StringBuilder b = new StringBuilder(); 1192 b.append(myResDef.getName()); 1193 return b; 1194 } else if (myParent != null) { 1195 StringBuilder b = myParent.buildPath(); 1196 if (b != null && myDef != null) { 1197 b.append('.'); 1198 b.append(myDef.getElementName()); 1199 } 1200 return b; 1201 } else { 1202 return null; 1203 } 1204 } 1205 1206 private boolean checkIfParentShouldBeEncodedAndBuildPath() { 1207 List<EncodeContextPath> encodeElements = myEncodeContext.myEncodeElementPaths; 1208 1209 String currentResourceName = myEncodeContext 1210 .getResourcePath() 1211 .get(myEncodeContext.getResourcePath().size() - 1) 1212 .getName(); 1213 if (myEncodeContext.myEncodeElementsAppliesToResourceTypes != null 1214 && !myEncodeContext.myEncodeElementsAppliesToResourceTypes.contains(currentResourceName)) { 1215 encodeElements = null; 1216 } 1217 1218 boolean retVal = checkIfPathMatchesForEncoding(encodeElements, true); 1219 1220 /* 1221 * We force the meta tag to be encoded even if it's not specified as an element in the 1222 * elements filter, specifically because we'll need it in order to automatically add 1223 * the SUBSETTED tag 1224 */ 1225 if (!retVal) { 1226 if ("meta".equals(myEncodeContext.getLeafResourcePathFirstField()) 1227 && shouldAddSubsettedTag(myEncodeContext)) { 1228 // The next element is a child of the <meta> element 1229 retVal = true; 1230 } else if ("meta".equals(myDef.getElementName()) && shouldAddSubsettedTag(myEncodeContext)) { 1231 // The next element is the <meta> element 1232 retVal = true; 1233 } 1234 } 1235 1236 return retVal; 1237 } 1238 1239 private boolean checkIfParentShouldNotBeEncodedAndBuildPath() { 1240 return checkIfPathMatchesForEncoding(myEncodeContext.myDontEncodeElementPaths, false); 1241 } 1242 1243 private boolean checkIfPathMatchesForEncoding( 1244 List<EncodeContextPath> theElements, boolean theCheckingForEncodeElements) { 1245 1246 boolean retVal = false; 1247 if (myDef != null) { 1248 myEncodeContext.pushPath(myDef.getElementName(), false); 1249 } 1250 1251 if (theCheckingForEncodeElements 1252 && isEncodeElementsAppliesToChildResourcesOnly() 1253 && myEncodeContext.getResourcePath().size() < 2) { 1254 retVal = true; 1255 } else if (theElements == null) { 1256 retVal = true; 1257 } else { 1258 EncodeContextPath currentResourcePath = myEncodeContext.getCurrentResourcePath(); 1259 ourLog.trace("Current resource path: {}", currentResourcePath); 1260 for (EncodeContextPath next : theElements) { 1261 1262 if (next.startsWith(currentResourcePath, true)) { 1263 if (theCheckingForEncodeElements 1264 || next.getPath().size() 1265 == currentResourcePath.getPath().size()) { 1266 retVal = true; 1267 break; 1268 } 1269 } 1270 1271 if (next.getPath().get(next.getPath().size() - 1).getName().equals("(mandatory)")) { 1272 if (myDef.getMin() > 0) { 1273 retVal = true; 1274 break; 1275 } 1276 if (currentResourcePath.getPath().size() 1277 > next.getPath().size()) { 1278 retVal = true; 1279 break; 1280 } 1281 } 1282 } 1283 } 1284 1285 if (myDef != null) { 1286 myEncodeContext.popPath(); 1287 } 1288 1289 return retVal; 1290 } 1291 1292 public BaseRuntimeChildDefinition getDef() { 1293 return myDef; 1294 } 1295 1296 public CompositeChildElement getParent() { 1297 return myParent; 1298 } 1299 1300 public boolean shouldBeEncoded(boolean theContainedResource) { 1301 boolean retVal = true; 1302 if (isSummaryMode() && (getDef() == null || !getDef().isSummary())) { 1303 String resourceName = myEncodeContext.getLeafResourceName(); 1304 // Technically the spec says we shouldn't include extensions in CapabilityStatement 1305 // but we will do so because there are people who depend on this behaviour, at least 1306 // as of 2019-07. See 1307 // https://github.com/smart-on-fhir/Swift-FHIR/issues/26 1308 // for example. 1309 if (("Conformance".equals(resourceName) || "CapabilityStatement".equals(resourceName)) 1310 && ("extension".equals(myDef.getElementName()) 1311 || "extension".equals(myEncodeContext.getLeafElementName()))) { 1312 // skip 1313 } else { 1314 retVal = false; 1315 } 1316 } 1317 if (myEncodeContext.myEncodeElementPaths != null) { 1318 retVal = checkIfParentShouldBeEncodedAndBuildPath(); 1319 } 1320 if (retVal && myEncodeContext.myDontEncodeElementPaths != null) { 1321 retVal = !checkIfParentShouldNotBeEncodedAndBuildPath(); 1322 } 1323 if (theContainedResource) { 1324 retVal = !notEncodeForContainedResource.contains(myDef.getElementName()); 1325 } 1326 1327 return retVal; 1328 } 1329 1330 @Override 1331 public int hashCode() { 1332 final int prime = 31; 1333 int result = 1; 1334 result = prime * result + ((myDef == null) ? 0 : myDef.hashCode()); 1335 result = prime * result + ((myParent == null) ? 0 : myParent.hashCode()); 1336 result = prime * result + ((myResDef == null) ? 0 : myResDef.hashCode()); 1337 result = prime * result + ((myEncodeContext == null) ? 0 : myEncodeContext.hashCode()); 1338 return result; 1339 } 1340 1341 @Override 1342 public boolean equals(Object obj) { 1343 if (this == obj) return true; 1344 1345 if (obj instanceof CompositeChildElement) { 1346 final CompositeChildElement that = (CompositeChildElement) obj; 1347 return Objects.equals(this.getEnclosingInstance(), that.getEnclosingInstance()) 1348 && Objects.equals(this.myDef, that.myDef) 1349 && Objects.equals(this.myParent, that.myParent) 1350 && Objects.equals(this.myResDef, that.myResDef) 1351 && Objects.equals(this.myEncodeContext, that.myEncodeContext); 1352 } 1353 return false; 1354 } 1355 1356 private BaseParser getEnclosingInstance() { 1357 return BaseParser.this; 1358 } 1359 } 1360 1361 private static class Key { 1362 private final BaseRuntimeElementCompositeDefinition<?> resDef; 1363 private final boolean theContainedResource; 1364 private final BaseParser.CompositeChildElement theParent; 1365 private final BaseParser.EncodeContext theEncodeContext; 1366 1367 public Key( 1368 BaseRuntimeElementCompositeDefinition<?> resDef, 1369 final boolean theContainedResource, 1370 final BaseParser.CompositeChildElement theParent, 1371 BaseParser.EncodeContext theEncodeContext) { 1372 this.resDef = resDef; 1373 this.theContainedResource = theContainedResource; 1374 this.theParent = theParent; 1375 this.theEncodeContext = theEncodeContext; 1376 } 1377 1378 @Override 1379 public int hashCode() { 1380 final int prime = 31; 1381 int result = 1; 1382 result = prime * result + ((resDef == null) ? 0 : resDef.hashCode()); 1383 result = prime * result + (theContainedResource ? 1231 : 1237); 1384 result = prime * result + ((theParent == null) ? 0 : theParent.hashCode()); 1385 result = prime * result + ((theEncodeContext == null) ? 0 : theEncodeContext.hashCode()); 1386 return result; 1387 } 1388 1389 @Override 1390 public boolean equals(final Object obj) { 1391 if (this == obj) { 1392 return true; 1393 } 1394 if (obj instanceof Key) { 1395 final Key that = (Key) obj; 1396 return Objects.equals(this.resDef, that.resDef) 1397 && this.theContainedResource == that.theContainedResource 1398 && Objects.equals(this.theParent, that.theParent) 1399 && Objects.equals(this.theEncodeContext, that.theEncodeContext); 1400 } 1401 return false; 1402 } 1403 } 1404 1405 protected static <T> List<T> extractMetadataListNotNull(IResource resource, ResourceMetadataKeyEnum<List<T>> key) { 1406 List<? extends T> securityLabels = key.get(resource); 1407 if (securityLabels == null) { 1408 securityLabels = Collections.emptyList(); 1409 } 1410 return new ArrayList<>(securityLabels); 1411 } 1412 1413 static boolean hasNoExtensions(IBase theElement) { 1414 if (theElement instanceof ISupportsUndeclaredExtensions) { 1415 ISupportsUndeclaredExtensions res = (ISupportsUndeclaredExtensions) theElement; 1416 if (res.getUndeclaredExtensions().size() > 0 1417 || res.getUndeclaredModifierExtensions().size() > 0) { 1418 return false; 1419 } 1420 } 1421 if (theElement instanceof IBaseHasExtensions) { 1422 IBaseHasExtensions res = (IBaseHasExtensions) theElement; 1423 if (res.hasExtension()) { 1424 return false; 1425 } 1426 } 1427 if (theElement instanceof IBaseHasModifierExtensions) { 1428 IBaseHasModifierExtensions res = (IBaseHasModifierExtensions) theElement; 1429 return !res.hasModifierExtension(); 1430 } 1431 return true; 1432 } 1433}