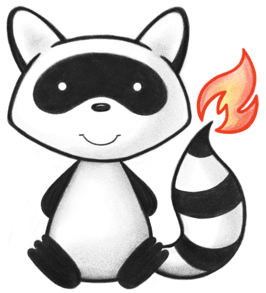
001/* 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.api; 021 022import org.apache.commons.lang3.Validate; 023 024import java.util.HashMap; 025 026/** 027 * Validation mode parameter for the $validate operation (DSTU2+ only) 028 */ 029public enum ValidationModeEnum { 030 /** 031 * The server checks the content, and then checks that the content would be acceptable as a create (e.g. that the content would not validate any uniqueness constraints) 032 */ 033 CREATE("create"), 034 035 /** 036 * The server checks the content, and then checks that it would accept it as an update against the nominated specific resource (e.g. that there are no changes to immutable fields the server does not allow to change, and checking version integrity if appropriate) 037 */ 038 UPDATE("update"), 039 040 /** 041 * The server ignores the content, and checks that the nominated resource is allowed to be deleted (e.g. checking referential integrity rules) 042 */ 043 DELETE("delete"); 044 045 private static HashMap<String, ValidationModeEnum> myCodeToValue; 046 private String myCode; 047 048 static { 049 myCodeToValue = new HashMap<String, ValidationModeEnum>(); 050 for (ValidationModeEnum next : values()) { 051 myCodeToValue.put(next.getCode(), next); 052 } 053 } 054 055 public static ValidationModeEnum forCode(String theCode) { 056 Validate.notBlank(theCode, "theCode must not be blank"); 057 return myCodeToValue.get(theCode); 058 } 059 060 public String getCode() { 061 return myCode; 062 } 063 064 private ValidationModeEnum(String theCode) { 065 myCode = theCode; 066 } 067 068 // @Override 069 // public boolean isEmpty() { 070 // return false; 071 // } 072}