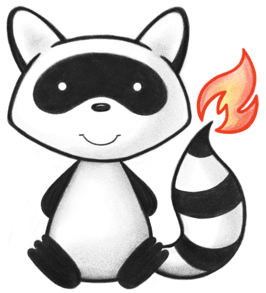
001/* 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.client.api; 021 022import ca.uhn.fhir.util.StopWatch; 023 024import java.io.IOException; 025import java.io.InputStream; 026import java.io.Reader; 027import java.util.List; 028import java.util.Map; 029 030/** 031 * An interface around the HTTP Response. 032 */ 033public interface IHttpResponse { 034 035 /** 036 * Buffer the message entity data. 037 * <p> 038 * In case the message entity is backed by an unconsumed entity input stream, 039 * all the bytes of the original entity input stream are read and stored in a 040 * local buffer. The original entity input stream is consumed. 041 * </p> 042 * <p> 043 * In case the response entity instance is not backed by an unconsumed input stream 044 * an invocation of {@code bufferEntity} method is ignored and the method returns. 045 * </p> 046 * <p> 047 * This operation is idempotent, i.e. it can be invoked multiple times with 048 * the same effect which also means that calling the {@code bufferEntity()} 049 * method on an already buffered (and thus closed) message instance is legal 050 * and has no further effect. 051 * </p> 052 * <p> 053 * Buffering the message entity data allows for multiple invocations of 054 * {@code readEntity(...)} methods on the response instance. 055 * 056 * @since 2.2 057 */ 058 void bufferEntity() throws IOException; 059 060 /** 061 * Close the response 062 */ 063 void close(); 064 065 /** 066 * Returns a reader for the response entity 067 */ 068 Reader createReader() throws IOException; 069 070 /** 071 * Get map of the response headers and corresponding string values. 072 * 073 * @return response headers as a map header keys and they values. 074 */ 075 Map<String, List<String>> getAllHeaders(); 076 077 /** 078 * Return all headers in the response with the given type 079 */ 080 List<String> getHeaders(String theName); 081 082 /** 083 * Extracts {@code Content-Type} value from the response exactly as 084 * specified by the {@code Content-Type} header. Returns {@code null} 085 * if not specified. 086 */ 087 String getMimeType(); 088 089 /** 090 * @return Returns a StopWatch that was started right before 091 * the client request was started. The time returned by this 092 * client includes any time that was spent within the HTTP 093 * library (possibly including waiting for a connection, and 094 * any network activity) 095 */ 096 StopWatch getRequestStopWatch(); 097 098 /** 099 * @return the native response, depending on the client library used 100 */ 101 Object getResponse(); 102 103 /** 104 * Get the status code associated with the response. 105 * 106 * @return the response status code. 107 */ 108 int getStatus(); 109 110 /** 111 * Get the response status information reason phrase associated with the response. 112 * 113 * @return the reason phrase. 114 */ 115 String getStatusInfo(); 116 117 /** 118 * Read the message entity input stream as an InputStream. 119 */ 120 InputStream readEntity() throws IOException; 121}