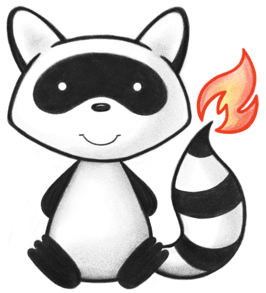
001/* 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.param; 021 022import ca.uhn.fhir.context.FhirContext; 023import ca.uhn.fhir.model.api.IQueryParameterType; 024import org.apache.commons.lang3.builder.ToStringBuilder; 025import org.apache.commons.lang3.builder.ToStringStyle; 026 027import java.math.BigDecimal; 028 029import static org.apache.commons.lang3.StringUtils.isBlank; 030import static org.apache.commons.lang3.StringUtils.isNotBlank; 031 032public class NumberParam extends BaseParamWithPrefix<NumberParam> implements IQueryParameterType { 033 034 private static final long serialVersionUID = 1L; 035 private BigDecimal myQuantity; 036 037 /** 038 * Constructor 039 */ 040 public NumberParam() { 041 super(); 042 } 043 044 /** 045 * Constructor 046 * 047 * @param theValue 048 * A value, e.g. "10" 049 */ 050 public NumberParam(int theValue) { 051 setValue(new BigDecimal(theValue)); 052 } 053 054 /** 055 * Constructor 056 * 057 * @param theValue 058 * A string value, e.g. "gt5.0" 059 */ 060 public NumberParam(String theValue) { 061 setValueAsQueryToken(null, null, null, theValue); 062 } 063 064 @Override 065 String doGetQueryParameterQualifier() { 066 return null; 067 } 068 069 @Override 070 String doGetValueAsQueryToken(FhirContext theContext) { 071 StringBuilder b = new StringBuilder(); 072 if (getPrefix() != null) { 073 b.append(ParameterUtil.escapeWithDefault(getPrefix().getValue())); 074 } 075 b.append(ParameterUtil.escapeWithDefault(myQuantity.toPlainString())); 076 return b.toString(); 077 } 078 079 @Override 080 void doSetValueAsQueryToken(FhirContext theContext, String theParamName, String theQualifier, String theValue) { 081 if (getMissing() != null && isBlank(theValue)) { 082 return; 083 } 084 String value = super.extractPrefixAndReturnRest(theValue); 085 myQuantity = null; 086 if (isNotBlank(value)) { 087 myQuantity = new BigDecimal(value); 088 } 089 } 090 091 public BigDecimal getValue() { 092 return myQuantity; 093 } 094 095 public NumberParam setValue(BigDecimal theValue) { 096 myQuantity = theValue; 097 return this; 098 } 099 100 @Override 101 public String toString() { 102 ToStringBuilder b = new ToStringBuilder(this, ToStringStyle.SIMPLE_STYLE); 103 b.append("prefix", getPrefix()); 104 b.append("value", myQuantity); 105 return b.build(); 106 } 107}