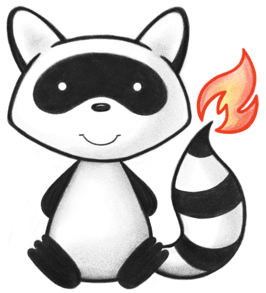
001/*- 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.util; 021 022public class HapiExtensions { 023 024 /** 025 * <p> 026 * This extension should be of type <code>string</code> and should be 027 * placed on the <code>Subscription.channel</code> element 028 * </p> 029 */ 030 public static final String EXT_SUBSCRIPTION_SUBJECT_TEMPLATE = 031 "http://hapifhir.io/fhir/StructureDefinition/subscription-email-subject-template"; 032 033 /** 034 * This extension URL indicates whether a REST HOOK delivery should 035 * include the version ID when delivering. 036 * <p> 037 * This extension should be of type <code>boolean</code> and should be 038 * placed on the <code>Subscription.channel</code> element. 039 * </p> 040 */ 041 public static final String EXT_SUBSCRIPTION_RESTHOOK_STRIP_VERSION_IDS = 042 "http://hapifhir.io/fhir/StructureDefinition/subscription-resthook-strip-version-ids"; 043 044 /** 045 * This extension URL indicates whether a REST HOOK delivery should 046 * reload the resource and deliver the latest version always. This 047 * could be useful for example if a resource which triggers a 048 * subscription gets updated many times in short succession and there 049 * is no value in delivering the older versions. 050 * <p> 051 * Note that if the resource is now deleted, this may cause 052 * the delivery to be cancelled altogether. 053 * </p> 054 * 055 * <p> 056 * This extension should be of type <code>boolean</code> and should be 057 * placed on the <code>Subscription.channel</code> element. 058 * </p> 059 */ 060 public static final String EXT_SUBSCRIPTION_RESTHOOK_DELIVER_LATEST_VERSION = 061 "http://hapifhir.io/fhir/StructureDefinition/subscription-resthook-deliver-latest-version"; 062 063 /** 064 * Indicate which strategy will be used to match this subscription 065 */ 066 public static final String EXT_SUBSCRIPTION_MATCHING_STRATEGY = 067 "http://hapifhir.io/fhir/StructureDefinition/subscription-matching-strategy"; 068 069 /** 070 * <p> 071 * This extension should be of type <code>string</code> and should be 072 * placed on the <code>Subscription.channel</code> element 073 * </p> 074 */ 075 public static final String EXT_SUBSCRIPTION_EMAIL_FROM = 076 "http://hapifhir.io/fhir/StructureDefinition/subscription-email-from"; 077 078 /** 079 * Extension ID for external binary references 080 */ 081 public static final String EXT_EXTERNALIZED_BINARY_ID = 082 "http://hapifhir.io/fhir/StructureDefinition/externalized-binary-id"; 083 084 /** 085 * For subscription, deliver a bundle containing a search result instead of just a single resource 086 */ 087 public static final String EXT_SUBSCRIPTION_PAYLOAD_SEARCH_CRITERIA = 088 "http://hapifhir.io/fhir/StructureDefinition/subscription-payload-search-criteria"; 089 090 /** 091 * Message added to expansion valueset 092 */ 093 public static final String EXT_VALUESET_EXPANSION_MESSAGE = 094 "http://hapifhir.io/fhir/StructureDefinition/valueset-expansion-message"; 095 096 /** 097 * Extension URL for extension on a SearchParameter indicating that text values should not be indexed 098 */ 099 public static final String EXT_SEARCHPARAM_TOKEN_SUPPRESS_TEXT_INDEXING = 100 "http://hapifhir.io/fhir/StructureDefinition/searchparameter-token-suppress-text-index"; 101 /** 102 * <p> 103 * This extension represents the equivalent of the 104 * <code>Resource.meta.source</code> field within R4+ resources, and is for 105 * use in DSTU3 resources. It should contain a value of type <code>uri</code> 106 * and will be located on the Resource.meta 107 * </p> 108 */ 109 public static final String EXT_META_SOURCE = "http://hapifhir.io/fhir/StructureDefinition/resource-meta-source"; 110 111 public static final String EXT_SP_UNIQUE = "http://hapifhir.io/fhir/StructureDefinition/sp-unique"; 112 113 /** 114 * URL for extension on a Search Parameter which determines whether it should be enabled for searching for resources 115 */ 116 public static final String EXT_SEARCHPARAM_ENABLED_FOR_SEARCHING = 117 "http://hapifhir.io/fhir/StructureDefinition/searchparameter-enabled-for-searching"; 118 119 /** 120 * URL for extension on a Phonetic String SearchParameter indicating that text values should be phonetically indexed with the named encoder 121 */ 122 public static final String EXT_SEARCHPARAM_PHONETIC_ENCODER = 123 "http://hapifhir.io/fhir/StructureDefinition/searchparameter-phonetic-encoder"; 124 125 /** 126 * URL for boolean extension added to all placeholder resources 127 */ 128 public static final String EXT_RESOURCE_PLACEHOLDER = 129 "http://hapifhir.io/fhir/StructureDefinition/resource-placeholder"; 130 131 /** 132 * URL for extension in a Group Bulk Export which identifies the golden patient of a given exported resource. 133 */ 134 public static final String ASSOCIATED_GOLDEN_RESOURCE_EXTENSION_URL = 135 "https://hapifhir.org/associated-patient-golden-resource/"; 136 137 /** 138 * This extension provides an example value for a parameter value for 139 * a REST operation (eg for an OperationDefinition) 140 */ 141 public static final String EXT_OP_PARAMETER_EXAMPLE_VALUE = 142 "http://hapifhir.io/fhir/StructureDefinition/op-parameter-example-value"; 143 144 /** 145 * This extension provides a way for subscribers to provide 146 * a "retry-count". 147 * If provided, subscriptions will be retried this many times 148 * (to a total of retry-count + 1 (for original attempt) 149 */ 150 public static final String EX_RETRY_COUNT = 151 "http://hapifhir.io/fhir/StructureDefinition/subscription-delivery-retry-count"; 152 153 /** 154 * This extension provides a way for subscribers to indicate if DELETE messages must be sent (default is ignoring them) 155 */ 156 public static final String EX_SEND_DELETE_MESSAGES = 157 "http://hapifhir.io/fhir/StructureDefinition/subscription-send-delete-messages"; 158 159 /** 160 * This entension allows subscriptions to be marked as cross partition and with correct settings, listen to incoming resources from all partitions. 161 */ 162 public static final String EXTENSION_SUBSCRIPTION_CROSS_PARTITION = 163 "https://smilecdr.com/fhir/ns/StructureDefinition/subscription-cross-partition"; 164 165 /** 166 * This extension is used for "uplifted refchains" on search parameters. See the 167 * HAPI FHIR documentation for an explanation of how these work. 168 */ 169 public static final String EXTENSION_SEARCHPARAM_UPLIFT_REFCHAIN = 170 "https://smilecdr.com/fhir/ns/StructureDefinition/searchparameter-uplift-refchain"; 171 172 /** 173 * This extension is used to enable auto version references at path for resource instances. 174 * This extension should be of type <code>string</code> and should be 175 * placed on the <code>Resource.meta</code> element. 176 * It is allowed to add multiple extensions with different paths. 177 */ 178 public static final String EXTENSION_AUTO_VERSION_REFERENCES_AT_PATH = 179 "http://hapifhir.io/fhir/StructureDefinition/auto-version-references-at-path"; 180 181 /** 182 * This extension is used for "uplifted refchains" on search parameters. See the 183 * HAPI FHIR documentation for an explanation of how these work. 184 */ 185 public static final String EXTENSION_SEARCHPARAM_UPLIFT_REFCHAIN_PARAM_CODE = "code"; 186 /** 187 * This extension is used for "uplifted refchains" on search parameters. See the 188 * HAPI FHIR documentation for an explanation of how these work. 189 */ 190 public static final String EXTENSION_SEARCHPARAM_UPLIFT_REFCHAIN_ELEMENT_NAME = "element-name"; 191 192 public static final String EXTENSION_SEARCHPARAM_CUSTOM_BASE_RESOURCE = 193 "http://hl7.org/fhir/tools/CustomBaseResource"; 194 public static final String EXTENSION_SEARCHPARAM_CUSTOM_TARGET_RESOURCE = 195 "http://hl7.org/fhir/tools/CustomTargetResource"; 196 197 /** 198 * When an OperationOutcome returned by the JPA server includes a declaration that a placeholder 199 * reference was automatically created, this extension indicates the ID of the placeholder. 200 */ 201 public static final String EXTENSION_PLACEHOLDER_ID = 202 "http://hapifhir.io/fhir/StructureDefinition/oo-placeholder-id"; 203 204 /** 205 * When a resource is replacing another resource, this extension can be added 206 * to include a reference to the resource that is being replaced. 207 */ 208 public static final String EXTENSION_REPLACES = "http://hapifhir.io/fhir/StructureDefinition/replaces"; 209 210 /** 211 * When a resource is replaced by another resource, this extension can be added 212 * to include a reference to the resource that is replacing it. 213 */ 214 public static final String EXTENSION_REPLACED_BY = "http://hapifhir.io/fhir/StructureDefinition/replaced-by"; 215 216 /** 217 * This extension is to specify the partition IDs for a request entry in a transaction bundle. 218 * This allows overriding partition id for a request entry in a transaction bundle so that a single transaction can 219 * have entries destined for different storage partitions. The expected value is a comma-separated string 220 * of partition IDs. 221 */ 222 public static final String EXTENSION_TRANSACTION_ENTRY_PARTITION_IDS = 223 "http://hapifhir.io/fhir/ns/StructureDefinition/request-partition-ids"; 224 /** 225 * Non instantiable 226 */ 227 private HapiExtensions() {} 228}