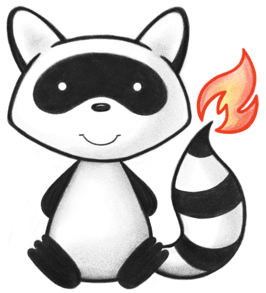
001/* 002 * #%L 003 * HAPI FHIR - Client Framework 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.client.apache; 021 022import ca.uhn.fhir.rest.client.api.BaseHttpRequest; 023import ca.uhn.fhir.rest.client.api.IHttpRequest; 024import ca.uhn.fhir.rest.client.api.IHttpResponse; 025import ca.uhn.fhir.util.StopWatch; 026import org.apache.commons.io.IOUtils; 027import org.apache.commons.lang3.Validate; 028import org.apache.http.Header; 029import org.apache.http.HttpEntity; 030import org.apache.http.HttpEntityEnclosingRequest; 031import org.apache.http.HttpResponse; 032import org.apache.http.client.HttpClient; 033import org.apache.http.client.methods.HttpRequestBase; 034import org.apache.http.entity.ContentType; 035 036import java.io.IOException; 037import java.net.URI; 038import java.nio.charset.Charset; 039import java.util.Collections; 040import java.util.HashMap; 041import java.util.LinkedList; 042import java.util.List; 043import java.util.Map; 044 045/** 046 * A Http Request based on Apache. This is an adapter around the class 047 * {@link org.apache.http.client.methods.HttpRequestBase HttpRequestBase} 048 * 049 * @author Peter Van Houte | peter.vanhoute@agfa.com | Agfa Healthcare 050 */ 051public class ApacheHttpRequest extends BaseHttpRequest implements IHttpRequest { 052 053 private HttpClient myClient; 054 private HttpRequestBase myRequest; 055 056 public ApacheHttpRequest(HttpClient theClient, HttpRequestBase theApacheRequest) { 057 this.myClient = theClient; 058 this.myRequest = theApacheRequest; 059 } 060 061 @Override 062 public void addHeader(String theName, String theValue) { 063 myRequest.addHeader(theName, theValue); 064 } 065 066 @Override 067 public IHttpResponse execute() throws IOException { 068 StopWatch responseStopWatch = new StopWatch(); 069 HttpResponse httpResponse = myClient.execute(myRequest); 070 return new ApacheHttpResponse(httpResponse, responseStopWatch); 071 } 072 073 @Override 074 public Map<String, List<String>> getAllHeaders() { 075 Map<String, List<String>> result = new HashMap<>(); 076 for (Header header : myRequest.getAllHeaders()) { 077 if (!result.containsKey(header.getName())) { 078 result.put(header.getName(), new LinkedList<>()); 079 } 080 result.get(header.getName()).add(header.getValue()); 081 } 082 return Collections.unmodifiableMap(result); 083 } 084 085 /** 086 * Get the ApacheRequest 087 * 088 * @return the ApacheRequest 089 */ 090 public HttpRequestBase getApacheRequest() { 091 return myRequest; 092 } 093 094 @Override 095 public String getHttpVerbName() { 096 return myRequest.getMethod(); 097 } 098 099 @Override 100 public void removeHeaders(String theHeaderName) { 101 Validate.notBlank(theHeaderName, "theHeaderName must not be null or blank"); 102 myRequest.removeHeaders(theHeaderName); 103 } 104 105 @Override 106 public String getRequestBodyFromStream() throws IOException { 107 if (myRequest instanceof HttpEntityEnclosingRequest) { 108 HttpEntity entity = ((HttpEntityEnclosingRequest) myRequest).getEntity(); 109 if (entity.isRepeatable()) { 110 final Header contentTypeHeader = myRequest.getFirstHeader("Content-Type"); 111 Charset charset = contentTypeHeader == null 112 ? null 113 : ContentType.parse(contentTypeHeader.getValue()).getCharset(); 114 return IOUtils.toString(entity.getContent(), charset); 115 } 116 } 117 return null; 118 } 119 120 @Override 121 public String getUri() { 122 return myRequest.getURI().toString(); 123 } 124 125 @Override 126 public void setUri(String theUrl) { 127 myRequest.setURI(URI.create(theUrl)); 128 } 129 130 @Override 131 public String toString() { 132 return myRequest.toString(); 133 } 134}