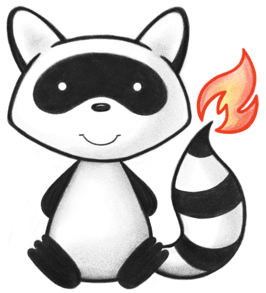
001/* 002 * #%L 003 * HAPI FHIR - Client Framework 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.client.interceptor; 021 022import ca.uhn.fhir.rest.api.Constants; 023import ca.uhn.fhir.rest.client.api.IClientInterceptor; 024import ca.uhn.fhir.rest.client.api.IHttpRequest; 025import ca.uhn.fhir.rest.client.api.IHttpResponse; 026import org.apache.commons.codec.binary.Base64; 027import org.apache.commons.lang3.StringUtils; 028import org.apache.commons.lang3.Validate; 029 030import java.io.IOException; 031 032/** 033 * HTTP interceptor to be used for adding HTTP basic auth username/password tokens 034 * to requests 035 * <p> 036 * See the <a href="https://hapifhir.io/hapi-fhir/docs/interceptors/built_in_client_interceptors.html">HAPI Documentation</a> 037 * for information on how to use this class. 038 * </p> 039 */ 040public class BasicAuthInterceptor implements IClientInterceptor { 041 042 private String myUsername; 043 private String myPassword; 044 private String myHeaderValue; 045 046 /** 047 * @param theUsername The username 048 * @param thePassword The password 049 */ 050 public BasicAuthInterceptor(String theUsername, String thePassword) { 051 this(StringUtils.defaultString(theUsername) + ":" + StringUtils.defaultString(thePassword)); 052 } 053 054 /** 055 * @param theCredentialString A credential string in the format <code>username:password</code> 056 */ 057 public BasicAuthInterceptor(String theCredentialString) { 058 Validate.notBlank(theCredentialString, "theCredentialString must not be null or blank"); 059 Validate.isTrue( 060 theCredentialString.contains(":"), "theCredentialString must be in the format 'username:password'"); 061 String encoded = Base64.encodeBase64String(theCredentialString.getBytes(Constants.CHARSET_US_ASCII)); 062 myHeaderValue = "Basic " + encoded; 063 } 064 065 @Override 066 public void interceptRequest(IHttpRequest theRequest) { 067 theRequest.addHeader(Constants.HEADER_AUTHORIZATION, myHeaderValue); 068 } 069 070 @Override 071 public void interceptResponse(IHttpResponse theResponse) throws IOException { 072 // nothing 073 } 074}