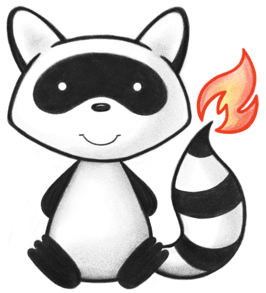
001/* 002 * #%L 003 * HAPI FHIR - Client Framework 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.client.interceptor; 021 022import ca.uhn.fhir.rest.api.Constants; 023import ca.uhn.fhir.rest.client.api.IClientInterceptor; 024import ca.uhn.fhir.rest.client.api.IHttpRequest; 025import ca.uhn.fhir.rest.client.api.IHttpResponse; 026import ca.uhn.fhir.util.CoverageIgnore; 027import org.apache.commons.lang3.Validate; 028 029/** 030 * HTTP interceptor to be used for adding HTTP Authorization using "bearer tokens" to requests. Bearer tokens are used for protocols such as OAUTH2 (see the 031 * <a href="http://tools.ietf.org/html/rfc6750">RFC 6750</a> specification on bearer token usage for more information). 032 * <p> 033 * This interceptor adds a header resembling the following:<br> 034 * <code>Authorization: Bearer dsfu9sd90fwp34.erw0-reu</code><br> 035 * where the token portion (at the end of the header) is supplied by the invoking code. 036 * </p> 037 * <p> 038 * See the <a href="https://hapifhir.io/hapi-fhir/docs/interceptors/built_in_client_interceptors.html">HAPI Documentation</a> for information on how to use this class. 039 * </p> 040 */ 041public class BearerTokenAuthInterceptor implements IClientInterceptor { 042 043 private String myToken; 044 045 /** 046 * Constructor. If this constructor is used, a token must be supplied later 047 */ 048 @CoverageIgnore 049 public BearerTokenAuthInterceptor() { 050 // nothing 051 } 052 053 /** 054 * Constructor 055 * 056 * @param theToken 057 * The bearer token to use (must not be null) 058 */ 059 public BearerTokenAuthInterceptor(String theToken) { 060 Validate.notNull(theToken, "theToken must not be null"); 061 myToken = theToken; 062 } 063 064 /** 065 * Returns the bearer token to use 066 */ 067 public String getToken() { 068 return myToken; 069 } 070 071 @Override 072 public void interceptRequest(IHttpRequest theRequest) { 073 theRequest.addHeader( 074 Constants.HEADER_AUTHORIZATION, (Constants.HEADER_AUTHORIZATION_VALPREFIX_BEARER + myToken)); 075 } 076 077 @Override 078 public void interceptResponse(IHttpResponse theResponse) { 079 // nothing 080 } 081 082 /** 083 * Sets the bearer token to use 084 */ 085 public void setToken(String theToken) { 086 Validate.notNull(theToken, "theToken must not be null"); 087 myToken = theToken; 088 } 089}