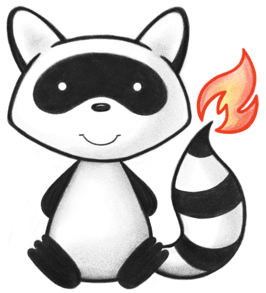
001/* 002 * #%L 003 * HAPI FHIR - Client Framework 004 * %% 005 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.client.interceptor; 021 022import ca.uhn.fhir.rest.client.api.IClientInterceptor; 023import ca.uhn.fhir.rest.client.api.IHttpRequest; 024import ca.uhn.fhir.rest.client.api.IHttpResponse; 025 026import java.io.IOException; 027 028/** 029 * HTTP interceptor to be used for adding HTTP headers containing user identifying info for auditing purposes to the request 030 */ 031public class UserInfoInterceptor implements IClientInterceptor { 032 033 public static final String HEADER_USER_ID = "fhir-user-id"; 034 public static final String HEADER_USER_NAME = "fhir-user-name"; 035 public static final String HEADER_APPLICATION_NAME = "fhir-app-name"; 036 037 private String myUserId; 038 private String myUserName; 039 private String myAppName; 040 041 public UserInfoInterceptor(String theUserId, String theUserName, String theAppName) { 042 super(); 043 myUserId = theUserId; 044 myUserName = theUserName; 045 myAppName = theAppName; 046 } 047 048 @Override 049 public void interceptRequest(IHttpRequest theRequest) { 050 if (myUserId != null) theRequest.addHeader(HEADER_USER_ID, myUserId); 051 if (myUserName != null) theRequest.addHeader(HEADER_USER_NAME, myUserName); 052 if (myAppName != null) theRequest.addHeader(HEADER_APPLICATION_NAME, myAppName); 053 } 054 055 @Override 056 public void interceptResponse(IHttpResponse theResponse) throws IOException { 057 // nothing 058 } 059}