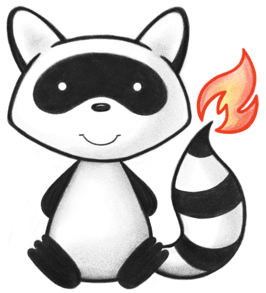
001/* 002 * #%L 003 * HAPI FHIR - Client Framework 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.client.method; 021 022import ca.uhn.fhir.context.ConfigurationException; 023import ca.uhn.fhir.context.FhirContext; 024import ca.uhn.fhir.context.RuntimeResourceDefinition; 025import ca.uhn.fhir.i18n.Msg; 026import ca.uhn.fhir.rest.annotation.Delete; 027import ca.uhn.fhir.rest.annotation.IdParam; 028import ca.uhn.fhir.rest.param.ParameterUtil; 029import org.hl7.fhir.instance.model.api.IBaseResource; 030 031import java.lang.reflect.Method; 032 033public abstract class BaseOutcomeReturningMethodBindingWithResourceIdButNoResourceBody 034 extends BaseOutcomeReturningMethodBinding { 035 036 private String myResourceName; 037 private Integer myIdParameterIndex; 038 039 public BaseOutcomeReturningMethodBindingWithResourceIdButNoResourceBody( 040 Method theMethod, 041 FhirContext theContext, 042 Object theProvider, 043 Class<?> theMethodAnnotationType, 044 Class<? extends IBaseResource> theResourceTypeFromAnnotation) { 045 super(theMethod, theContext, theMethodAnnotationType, theProvider); 046 047 Class<? extends IBaseResource> resourceType = theResourceTypeFromAnnotation; 048 if (resourceType != IBaseResource.class) { 049 RuntimeResourceDefinition def = theContext.getResourceDefinition(resourceType); 050 myResourceName = def.getName(); 051 } else { 052 throw new ConfigurationException( 053 Msg.code(1474) + "Can not determine resource type for method '" + theMethod.getName() + "' on type " 054 + theMethod.getDeclaringClass().getCanonicalName() 055 + " - Did you forget to include the resourceType() value on the @" 056 + Delete.class.getSimpleName() + " method annotation?"); 057 } 058 059 myIdParameterIndex = ParameterUtil.findIdParameterIndex(theMethod, getContext()); 060 if (myIdParameterIndex == null) { 061 throw new ConfigurationException(Msg.code(1475) + "Method '" + theMethod.getName() + "' on type '" 062 + theMethod.getDeclaringClass().getCanonicalName() + "' has no parameter annotated with the @" 063 + IdParam.class.getSimpleName() + " annotation"); 064 } 065 } 066 067 @Override 068 public String getResourceName() { 069 return myResourceName; 070 } 071 072 protected Integer getIdParameterIndex() { 073 return myIdParameterIndex; 074 } 075}