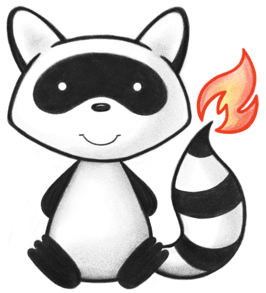
001/* 002 * #%L 003 * HAPI FHIR - Client Framework 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.client.method; 021 022import ca.uhn.fhir.context.FhirContext; 023import ca.uhn.fhir.i18n.Msg; 024import ca.uhn.fhir.rest.api.QualifiedParamList; 025import ca.uhn.fhir.rest.api.RestSearchParameterTypeEnum; 026import ca.uhn.fhir.rest.server.exceptions.InternalErrorException; 027import org.hl7.fhir.instance.model.api.IBaseResource; 028 029import java.lang.reflect.Method; 030import java.util.ArrayList; 031import java.util.Collection; 032import java.util.List; 033import java.util.Map; 034 035import static org.apache.commons.lang3.StringUtils.isNotBlank; 036 037public abstract class BaseQueryParameter implements IParameter { 038 039 public abstract List<QualifiedParamList> encode(FhirContext theContext, Object theObject) 040 throws InternalErrorException; 041 042 public abstract String getName(); 043 044 public abstract RestSearchParameterTypeEnum getParamType(); 045 046 @Override 047 public void initializeTypes( 048 Method theMethod, 049 Class<? extends Collection<?>> theOuterCollectionType, 050 Class<? extends Collection<?>> theInnerCollectionType, 051 Class<?> theParameterType) { 052 // ignore for now 053 } 054 055 public abstract boolean isRequired(); 056 057 @Override 058 public void translateClientArgumentIntoQueryArgument( 059 FhirContext theContext, 060 Object theSourceClientArgument, 061 Map<String, List<String>> theTargetQueryArguments, 062 IBaseResource theTargetResource) 063 throws InternalErrorException { 064 if (theSourceClientArgument == null) { 065 if (isRequired()) { 066 throw new NullPointerException( 067 Msg.code(1451) + "SearchParameter '" + getName() + "' is required and may not be null"); 068 } 069 } else { 070 List<QualifiedParamList> value = encode(theContext, theSourceClientArgument); 071 072 for (QualifiedParamList nextParamEntry : value) { 073 StringBuilder b = new StringBuilder(); 074 for (String str : nextParamEntry) { 075 if (b.length() > 0) { 076 b.append(","); 077 } 078 b.append(str); 079 } 080 081 String qualifier = nextParamEntry.getQualifier(); 082 String paramName = isNotBlank(qualifier) ? getName() + qualifier : getName(); 083 List<String> paramValues = 084 theTargetQueryArguments.computeIfAbsent(paramName, k -> new ArrayList<>(value.size())); 085 086 paramValues.add(b.toString()); 087 } 088 } 089 } 090}