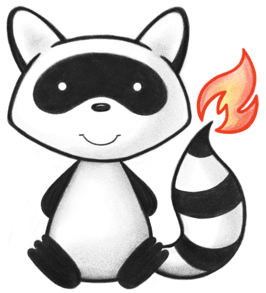
001/* 002 * #%L 003 * HAPI FHIR - Client Framework 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.client.method; 021 022import ca.uhn.fhir.context.ConfigurationException; 023import ca.uhn.fhir.context.FhirContext; 024import ca.uhn.fhir.i18n.Msg; 025import ca.uhn.fhir.model.valueset.BundleTypeEnum; 026import ca.uhn.fhir.rest.api.RestOperationTypeEnum; 027import ca.uhn.fhir.rest.server.exceptions.InternalErrorException; 028import org.hl7.fhir.instance.model.api.IBaseConformance; 029 030import java.lang.reflect.Method; 031 032public class ConformanceMethodBinding extends BaseResourceReturningMethodBinding { 033 034 public ConformanceMethodBinding(Method theMethod, FhirContext theContext, Object theProvider) { 035 super(theMethod.getReturnType(), theMethod, theContext, theProvider); 036 037 MethodReturnTypeEnum methodReturnType = getMethodReturnType(); 038 Class<?> genericReturnType = (Class<?>) theMethod.getGenericReturnType(); 039 if (methodReturnType != MethodReturnTypeEnum.RESOURCE 040 || !IBaseConformance.class.isAssignableFrom(genericReturnType)) { 041 throw new ConfigurationException( 042 Msg.code(1426) + "Conformance resource provider method '" + theMethod.getName() 043 + "' should return a Conformance resource class, returns: " + theMethod.getReturnType()); 044 } 045 } 046 047 @Override 048 public ReturnTypeEnum getReturnType() { 049 return ReturnTypeEnum.RESOURCE; 050 } 051 052 @Override 053 public HttpGetClientInvocation invokeClient(Object[] theArgs) throws InternalErrorException { 054 HttpGetClientInvocation retVal = MethodUtil.createConformanceInvocation(getContext()); 055 056 if (theArgs != null) { 057 for (int idx = 0; idx < theArgs.length; idx++) { 058 IParameter nextParam = getParameters().get(idx); 059 nextParam.translateClientArgumentIntoQueryArgument(getContext(), theArgs[idx], null, null); 060 } 061 } 062 063 return retVal; 064 } 065 066 @Override 067 public RestOperationTypeEnum getRestOperationType() { 068 return RestOperationTypeEnum.METADATA; 069 } 070 071 @Override 072 protected BundleTypeEnum getResponseBundleType() { 073 return null; 074 } 075}