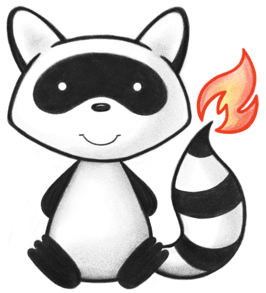
001/* 002 * #%L 003 * HAPI FHIR - Client Framework 004 * %% 005 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.client.method; 021 022import ca.uhn.fhir.context.ConfigurationException; 023import ca.uhn.fhir.context.FhirContext; 024import ca.uhn.fhir.i18n.Msg; 025import ca.uhn.fhir.model.primitive.IntegerDt; 026import ca.uhn.fhir.rest.annotation.Count; 027import ca.uhn.fhir.rest.api.Constants; 028import ca.uhn.fhir.rest.param.ParameterUtil; 029import ca.uhn.fhir.rest.server.exceptions.InternalErrorException; 030import org.hl7.fhir.instance.model.api.IBaseResource; 031 032import java.lang.reflect.Method; 033import java.util.Collection; 034import java.util.Collections; 035import java.util.List; 036import java.util.Map; 037 038public class CountParameter implements IParameter { 039 040 @Override 041 public void translateClientArgumentIntoQueryArgument( 042 FhirContext theContext, 043 Object theSourceClientArgument, 044 Map<String, List<String>> theTargetQueryArguments, 045 IBaseResource theTargetResource) 046 throws InternalErrorException { 047 if (theSourceClientArgument != null) { 048 IntegerDt since = ParameterUtil.toInteger(theSourceClientArgument); 049 if (since.isEmpty() == false) { 050 theTargetQueryArguments.put(Constants.PARAM_COUNT, Collections.singletonList(since.getValueAsString())); 051 } 052 } 053 } 054 055 @Override 056 public void initializeTypes( 057 Method theMethod, 058 Class<? extends Collection<?>> theOuterCollectionType, 059 Class<? extends Collection<?>> theInnerCollectionType, 060 Class<?> theParameterType) { 061 if (theOuterCollectionType != null) { 062 throw new ConfigurationException(Msg.code(1420) + "Method '" + theMethod.getName() + "' in type '" 063 + theMethod.getDeclaringClass().getCanonicalName() + "' is annotated with @" + Count.class.getName() 064 + " but can not be of collection type"); 065 } 066 if (!ParameterUtil.isBindableIntegerType(theParameterType)) { 067 throw new ConfigurationException(Msg.code(1421) + "Method '" + theMethod.getName() + "' in type '" 068 + theMethod.getDeclaringClass().getCanonicalName() + "' is annotated with @" + Count.class.getName() 069 + " but type '" + theParameterType + "' is an invalid type, must be Integer or IntegerType"); 070 } 071 } 072}