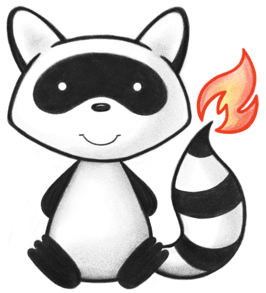
001/* 002 * #%L 003 * HAPI FHIR - Client Framework 004 * %% 005 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.client.method; 021 022import ca.uhn.fhir.context.FhirContext; 023import ca.uhn.fhir.context.FhirVersionEnum; 024import ca.uhn.fhir.i18n.Msg; 025import ca.uhn.fhir.rest.annotation.Create; 026import ca.uhn.fhir.rest.api.RequestTypeEnum; 027import ca.uhn.fhir.rest.api.RestOperationTypeEnum; 028import ca.uhn.fhir.rest.client.impl.BaseHttpClientInvocation; 029import ca.uhn.fhir.rest.server.exceptions.InvalidRequestException; 030import org.hl7.fhir.instance.model.api.IBaseResource; 031import org.hl7.fhir.instance.model.api.IIdType; 032 033import java.lang.reflect.Method; 034import java.util.Collections; 035import java.util.Set; 036 037import static org.apache.commons.lang3.StringUtils.isNotBlank; 038 039public class CreateMethodBinding extends BaseOutcomeReturningMethodBindingWithResourceParam { 040 041 public CreateMethodBinding(Method theMethod, FhirContext theContext, Object theProvider) { 042 super(theMethod, theContext, Create.class, theProvider); 043 } 044 045 @Override 046 protected BaseHttpClientInvocation createClientInvocation(Object[] theArgs, IBaseResource theResource) { 047 FhirContext context = getContext(); 048 049 BaseHttpClientInvocation retVal = MethodUtil.createCreateInvocation(theResource, context); 050 051 if (theArgs != null) { 052 for (int idx = 0; idx < theArgs.length; idx++) { 053 IParameter nextParam = getParameters().get(idx); 054 nextParam.translateClientArgumentIntoQueryArgument(getContext(), theArgs[idx], null, null); 055 } 056 } 057 058 return retVal; 059 } 060 061 @Override 062 protected String getMatchingOperation() { 063 return null; 064 } 065 066 @Override 067 public RestOperationTypeEnum getRestOperationType() { 068 return RestOperationTypeEnum.CREATE; 069 } 070 071 @Override 072 protected Set<RequestTypeEnum> provideAllowableRequestTypes() { 073 return Collections.singleton(RequestTypeEnum.POST); 074 } 075 076 @Override 077 protected void validateResourceIdAndUrlIdForNonConditionalOperation( 078 IBaseResource theResource, String theResourceId, String theUrlId, String theMatchUrl) { 079 if (isNotBlank(theUrlId)) { 080 String msg = getContext() 081 .getLocalizer() 082 .getMessage(BaseOutcomeReturningMethodBindingWithResourceParam.class, "idInUrlForCreate", theUrlId); 083 throw new InvalidRequestException(Msg.code(1411) + msg); 084 } 085 if (getContext().getVersion().getVersion().isOlderThan(FhirVersionEnum.DSTU3)) { 086 if (isNotBlank(theResourceId)) { 087 String msg = getContext() 088 .getLocalizer() 089 .getMessage( 090 BaseOutcomeReturningMethodBindingWithResourceParam.class, 091 "idInBodyForCreate", 092 theResourceId); 093 throw new InvalidRequestException(Msg.code(1412) + msg); 094 } 095 } else { 096 theResource.setId((IIdType) null); 097 } 098 } 099}