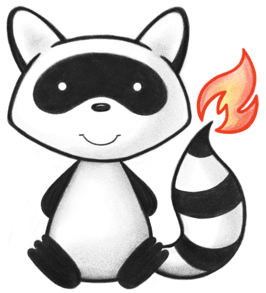
001/* 002 * #%L 003 * HAPI FHIR - Client Framework 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.client.method; 021 022import ca.uhn.fhir.context.FhirContext; 023import ca.uhn.fhir.i18n.Msg; 024import ca.uhn.fhir.rest.annotation.Delete; 025import ca.uhn.fhir.rest.api.RequestTypeEnum; 026import ca.uhn.fhir.rest.api.RestOperationTypeEnum; 027import ca.uhn.fhir.rest.client.impl.BaseHttpClientInvocation; 028import ca.uhn.fhir.rest.server.exceptions.InternalErrorException; 029import ca.uhn.fhir.rest.server.exceptions.InvalidRequestException; 030import org.hl7.fhir.instance.model.api.IBaseResource; 031import org.hl7.fhir.instance.model.api.IIdType; 032 033import java.lang.reflect.Method; 034import java.util.Collections; 035import java.util.List; 036import java.util.Map; 037import java.util.Set; 038 039public class DeleteMethodBinding extends BaseOutcomeReturningMethodBindingWithResourceIdButNoResourceBody { 040 041 public DeleteMethodBinding(Method theMethod, FhirContext theContext, Object theProvider) { 042 super( 043 theMethod, 044 theContext, 045 theProvider, 046 Delete.class, 047 theMethod.getAnnotation(Delete.class).type()); 048 } 049 050 @Override 051 public RestOperationTypeEnum getRestOperationType() { 052 return RestOperationTypeEnum.DELETE; 053 } 054 055 @Override 056 protected Set<RequestTypeEnum> provideAllowableRequestTypes() { 057 return Collections.singleton(RequestTypeEnum.DELETE); 058 } 059 060 @Override 061 protected BaseHttpClientInvocation createClientInvocation(Object[] theArgs, IBaseResource theResource) { 062 StringBuilder urlExtension = new StringBuilder(); 063 urlExtension.append(getContext().getResourceType(theResource)); 064 065 return new HttpPostClientInvocation(getContext(), theResource, urlExtension.toString()); 066 } 067 068 @Override 069 protected boolean allowVoidReturnType() { 070 return true; 071 } 072 073 @Override 074 public BaseHttpClientInvocation invokeClient(Object[] theArgs) throws InternalErrorException { 075 IIdType id = (IIdType) theArgs[getIdParameterIndex()]; 076 if (id == null) { 077 throw new NullPointerException(Msg.code(1472) + "ID can not be null"); 078 } 079 080 if (id.hasResourceType() == false) { 081 id = id.withResourceType(getResourceName()); 082 } else if (getResourceName().equals(id.getResourceType()) == false) { 083 throw new InvalidRequestException(Msg.code(1473) + "ID parameter has the wrong resource type, expected '" 084 + getResourceName() + "', found: " + id.getResourceType()); 085 } 086 087 HttpDeleteClientInvocation retVal = createDeleteInvocation(getContext(), id, Collections.emptyMap()); 088 089 for (int idx = 0; idx < theArgs.length; idx++) { 090 IParameter nextParam = getParameters().get(idx); 091 nextParam.translateClientArgumentIntoQueryArgument(getContext(), theArgs[idx], null, null); 092 } 093 094 return retVal; 095 } 096 097 public static HttpDeleteClientInvocation createDeleteInvocation( 098 FhirContext theContext, IIdType theId, Map<String, List<String>> theAdditionalParams) { 099 return new HttpDeleteClientInvocation(theContext, theId, theAdditionalParams); 100 } 101 102 @Override 103 protected String getMatchingOperation() { 104 return null; 105 } 106 107 public static HttpDeleteClientInvocation createDeleteInvocation( 108 FhirContext theContext, String theSearchUrl, Map<String, List<String>> theParams) { 109 return new HttpDeleteClientInvocation(theContext, theSearchUrl, theParams); 110 } 111}