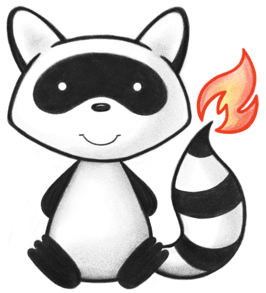
001/* 002 * #%L 003 * HAPI FHIR - Client Framework 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.client.method; 021 022import ca.uhn.fhir.context.ConfigurationException; 023import ca.uhn.fhir.context.FhirContext; 024import ca.uhn.fhir.i18n.Msg; 025import ca.uhn.fhir.rest.api.Constants; 026import ca.uhn.fhir.rest.api.SummaryEnum; 027import ca.uhn.fhir.rest.server.exceptions.InternalErrorException; 028import org.hl7.fhir.instance.model.api.IBaseResource; 029 030import java.lang.reflect.Method; 031import java.util.Collection; 032import java.util.Collections; 033import java.util.List; 034import java.util.Map; 035 036import static org.apache.commons.lang3.StringUtils.isNotBlank; 037 038public class ElementsParameter implements IParameter { 039 040 @SuppressWarnings("unchecked") 041 @Override 042 public void translateClientArgumentIntoQueryArgument( 043 FhirContext theContext, 044 Object theSourceClientArgument, 045 Map<String, List<String>> theTargetQueryArguments, 046 IBaseResource theTargetResource) 047 throws InternalErrorException { 048 if (theSourceClientArgument instanceof Collection) { 049 StringBuilder values = new StringBuilder(); 050 for (String next : (Collection<String>) theSourceClientArgument) { 051 if (isNotBlank(next)) { 052 if (values.length() > 0) { 053 values.append(','); 054 } 055 values.append(next); 056 } 057 } 058 theTargetQueryArguments.put(Constants.PARAM_ELEMENTS, Collections.singletonList(values.toString())); 059 } else { 060 String elements = (String) theSourceClientArgument; 061 if (elements != null) { 062 theTargetQueryArguments.put(Constants.PARAM_ELEMENTS, Collections.singletonList(elements)); 063 } 064 } 065 } 066 067 @Override 068 public void initializeTypes( 069 Method theMethod, 070 Class<? extends Collection<?>> theOuterCollectionType, 071 Class<? extends Collection<?>> theInnerCollectionType, 072 Class<?> theParameterType) { 073 if (theOuterCollectionType != null) { 074 throw new ConfigurationException(Msg.code(1445) + "Method '" + theMethod.getName() + "' in type '" 075 + theMethod.getDeclaringClass().getCanonicalName() + "' is of type " + SummaryEnum.class 076 + " but can not be a collection of collections"); 077 } 078 } 079}