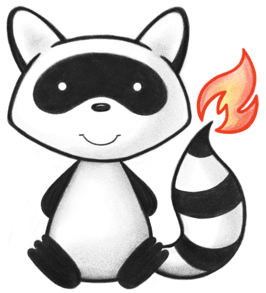
001/* 002 * #%L 003 * HAPI FHIR - Client Framework 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.client.method; 021 022import ca.uhn.fhir.context.FhirContext; 023import ca.uhn.fhir.rest.api.EncodingEnum; 024import ca.uhn.fhir.rest.api.RequestTypeEnum; 025import ca.uhn.fhir.rest.client.api.IHttpRequest; 026import ca.uhn.fhir.rest.client.impl.BaseHttpClientInvocation; 027import org.hl7.fhir.instance.model.api.IIdType; 028 029import java.util.List; 030import java.util.Map; 031 032public class HttpDeleteClientInvocation extends BaseHttpClientInvocation { 033 034 private String myUrlPath; 035 private Map<String, List<String>> myParams; 036 037 public HttpDeleteClientInvocation( 038 FhirContext theContext, IIdType theId, Map<String, List<String>> theAdditionalParams) { 039 super(theContext); 040 myUrlPath = theId.toUnqualifiedVersionless().getValue(); 041 myParams = theAdditionalParams; 042 } 043 044 public HttpDeleteClientInvocation( 045 FhirContext theContext, String theSearchUrl, Map<String, List<String>> theParams) { 046 super(theContext); 047 myUrlPath = theSearchUrl; 048 myParams = theParams; 049 } 050 051 @Override 052 public IHttpRequest asHttpRequest( 053 String theUrlBase, 054 Map<String, List<String>> theExtraParams, 055 EncodingEnum theEncoding, 056 Boolean thePrettyPrint) { 057 StringBuilder b = new StringBuilder(); 058 b.append(theUrlBase); 059 if (!theUrlBase.endsWith("/")) { 060 b.append('/'); 061 } 062 b.append(myUrlPath); 063 064 appendExtraParamsWithQuestionMark(myParams, b, b.indexOf("?") == -1); 065 appendExtraParamsWithQuestionMark(theExtraParams, b, b.indexOf("?") == -1); 066 067 return createHttpRequest(b.toString(), theEncoding, RequestTypeEnum.DELETE); 068 } 069}