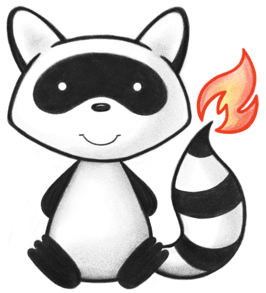
001/* 002 * #%L 003 * HAPI FHIR - Client Framework 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.client.method; 021 022import ca.uhn.fhir.context.FhirContext; 023import ca.uhn.fhir.rest.api.EncodingEnum; 024import ca.uhn.fhir.rest.api.RequestTypeEnum; 025import ca.uhn.fhir.rest.client.api.IHttpRequest; 026import ca.uhn.fhir.rest.client.api.UrlSourceEnum; 027import ca.uhn.fhir.rest.client.impl.BaseHttpClientInvocation; 028import ca.uhn.fhir.util.UrlUtil; 029import org.apache.commons.lang3.StringUtils; 030 031import java.util.HashMap; 032import java.util.List; 033import java.util.Map; 034import java.util.Map.Entry; 035 036/** 037 * @author James Agnew 038 * @author Doug Martin (Regenstrief Center for Biomedical Informatics) 039 */ 040public class HttpGetClientInvocation extends BaseHttpClientInvocation { 041 042 private final Map<String, List<String>> myParameters; 043 private final String myUrlPath; 044 private final UrlSourceEnum myUrlSource; 045 046 public HttpGetClientInvocation( 047 FhirContext theContext, Map<String, List<String>> theParameters, String... theUrlFragments) { 048 this(theContext, theParameters, UrlSourceEnum.GENERATED, theUrlFragments); 049 } 050 051 public HttpGetClientInvocation( 052 FhirContext theContext, 053 Map<String, List<String>> theParameters, 054 UrlSourceEnum theUrlSource, 055 String... theUrlFragments) { 056 super(theContext); 057 myParameters = theParameters; 058 myUrlPath = StringUtils.join(theUrlFragments, '/'); 059 myUrlSource = theUrlSource; 060 } 061 062 public HttpGetClientInvocation(FhirContext theContext, String theUrlPath) { 063 super(theContext); 064 myParameters = new HashMap<>(); 065 myUrlPath = theUrlPath; 066 myUrlSource = UrlSourceEnum.GENERATED; 067 } 068 069 private boolean addQueryParameter(StringBuilder b, boolean first, String nextKey, String nextValue) { 070 boolean retVal = first; 071 if (retVal) { 072 b.append('?'); 073 retVal = false; 074 } else { 075 b.append('&'); 076 } 077 b.append(UrlUtil.escapeUrlParam(nextKey)); 078 b.append('='); 079 b.append(UrlUtil.escapeUrlParam(nextValue)); 080 081 return retVal; 082 } 083 084 @Override 085 public IHttpRequest asHttpRequest( 086 String theUrlBase, 087 Map<String, List<String>> theExtraParams, 088 EncodingEnum theEncoding, 089 Boolean thePrettyPrint) { 090 StringBuilder b = new StringBuilder(); 091 092 if (!myUrlPath.contains("://")) { 093 b.append(theUrlBase); 094 if (!theUrlBase.endsWith("/") && !myUrlPath.startsWith("/")) { 095 b.append('/'); 096 } 097 } 098 b.append(myUrlPath); 099 100 boolean first = b.indexOf("?") == -1; 101 for (Entry<String, List<String>> next : myParameters.entrySet()) { 102 if (next.getValue() == null || next.getValue().isEmpty()) { 103 continue; 104 } 105 String nextKey = next.getKey(); 106 for (String nextValue : next.getValue()) { 107 first = addQueryParameter(b, first, nextKey, nextValue); 108 } 109 } 110 111 appendExtraParamsWithQuestionMark(theExtraParams, b, first); 112 113 IHttpRequest retVal = super.createHttpRequest(b.toString(), theEncoding, RequestTypeEnum.GET); 114 retVal.setUrlSource(myUrlSource); 115 116 return retVal; 117 } 118 119 public Map<String, List<String>> getParameters() { 120 return myParameters; 121 } 122 123 public String getUrlPath() { 124 return myUrlPath; 125 } 126}