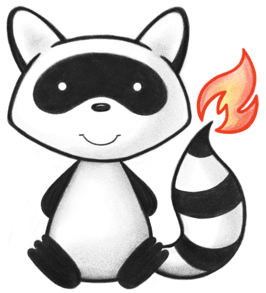
001/* 002 * #%L 003 * HAPI FHIR - Client Framework 004 * %% 005 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.client.method; 021 022import ca.uhn.fhir.context.FhirContext; 023import ca.uhn.fhir.rest.api.EncodingEnum; 024import ca.uhn.fhir.rest.api.RequestTypeEnum; 025import ca.uhn.fhir.rest.client.api.IHttpClient; 026import ca.uhn.fhir.rest.client.api.IHttpRequest; 027import ca.uhn.fhir.rest.client.impl.BaseHttpClientInvocation; 028import org.hl7.fhir.instance.model.api.IIdType; 029 030import java.util.List; 031import java.util.Map; 032 033public class HttpPatchClientInvocation extends BaseHttpClientInvocation { 034 035 private String myUrlPath; 036 private Map<String, List<String>> myParams; 037 private String myContents; 038 private String myContentType; 039 040 public HttpPatchClientInvocation(FhirContext theContext, IIdType theId, String theContentType, String theContents) { 041 super(theContext); 042 myUrlPath = theId.toUnqualifiedVersionless().getValue(); 043 myContentType = theContentType; 044 myContents = theContents; 045 } 046 047 public HttpPatchClientInvocation( 048 FhirContext theContext, String theUrlPath, String theContentType, String theContents) { 049 super(theContext); 050 myUrlPath = theUrlPath; 051 myContentType = theContentType; 052 myContents = theContents; 053 } 054 055 @Override 056 public IHttpRequest asHttpRequest( 057 String theUrlBase, 058 Map<String, List<String>> theExtraParams, 059 EncodingEnum theEncoding, 060 Boolean thePrettyPrint) { 061 StringBuilder b = new StringBuilder(); 062 b.append(theUrlBase); 063 if (!theUrlBase.endsWith("/")) { 064 b.append('/'); 065 } 066 b.append(myUrlPath); 067 068 appendExtraParamsWithQuestionMark(myParams, b, b.indexOf("?") == -1); 069 appendExtraParamsWithQuestionMark(theExtraParams, b, b.indexOf("?") == -1); 070 071 return createHttpRequest(b.toString(), theEncoding, RequestTypeEnum.PATCH); 072 } 073 074 @Override 075 protected IHttpRequest createHttpRequest(String theUrl, EncodingEnum theEncoding, RequestTypeEnum theRequestType) { 076 IHttpClient httpClient = getRestfulClientFactory() 077 .getHttpClient(new StringBuilder(theUrl), null, null, theRequestType, getHeaders()); 078 return httpClient.createByteRequest(getContext(), myContents, myContentType, null); 079 } 080}