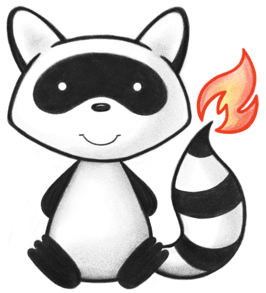
001/*- 002 * #%L 003 * HAPI FHIR - Client Framework 004 * %% 005 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.client.method; 021 022import ca.uhn.fhir.context.FhirContext; 023import ca.uhn.fhir.rest.server.exceptions.InternalErrorException; 024import org.hl7.fhir.instance.model.api.IBaseResource; 025 026import java.lang.reflect.Method; 027import java.util.Collection; 028import java.util.List; 029import java.util.Map; 030 031public class ResourceParameter implements IParameter { 032 033 private Class<? extends IBaseResource> myResourceType; 034 035 /** 036 * Constructor 037 */ 038 @SuppressWarnings("unchecked") 039 public ResourceParameter(Class<?> theParameterType) { 040 if (IBaseResource.class.isAssignableFrom(theParameterType)) { 041 myResourceType = (Class<? extends IBaseResource>) theParameterType; 042 } 043 } 044 045 @Override 046 public void initializeTypes( 047 Method theMethod, 048 Class<? extends Collection<?>> theOuterCollectionType, 049 Class<? extends Collection<?>> theInnerCollectionType, 050 Class<?> theParameterType) { 051 // ignore for now 052 } 053 054 @Override 055 public void translateClientArgumentIntoQueryArgument( 056 FhirContext theContext, 057 Object theSourceClientArgument, 058 Map<String, List<String>> theTargetQueryArguments, 059 IBaseResource theTargetResource) 060 throws InternalErrorException { 061 // ignore, as this is handles as a special case 062 } 063 064 public enum Mode { 065 BODY, 066 BODY_BYTE_ARRAY, 067 ENCODING, 068 RESOURCE 069 } 070 071 public Class<? extends IBaseResource> getResourceType() { 072 return myResourceType; 073 } 074}