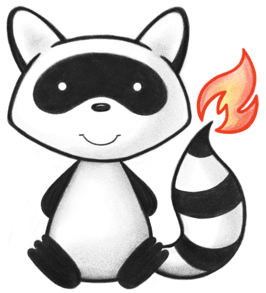
001/*- 002 * #%L 003 * HAPI FHIR - Client Framework 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.client.method; 021 022import ca.uhn.fhir.context.FhirContext; 023import ca.uhn.fhir.i18n.Msg; 024import ca.uhn.fhir.rest.annotation.Update; 025import ca.uhn.fhir.rest.api.RequestTypeEnum; 026import ca.uhn.fhir.rest.api.RestOperationTypeEnum; 027import ca.uhn.fhir.rest.client.impl.BaseHttpClientInvocation; 028import ca.uhn.fhir.rest.param.ParameterUtil; 029import ca.uhn.fhir.rest.server.exceptions.InvalidRequestException; 030import org.hl7.fhir.instance.model.api.IBaseResource; 031import org.hl7.fhir.instance.model.api.IIdType; 032 033import java.lang.reflect.Method; 034import java.util.Collections; 035import java.util.Set; 036 037import static org.apache.commons.lang3.StringUtils.isBlank; 038 039public class UpdateMethodBinding extends BaseOutcomeReturningMethodBindingWithResourceParam { 040 041 private Integer myIdParameterIndex; 042 043 public UpdateMethodBinding(Method theMethod, FhirContext theContext, Object theProvider) { 044 super(theMethod, theContext, Update.class, theProvider); 045 046 myIdParameterIndex = ParameterUtil.findIdParameterIndex(theMethod, getContext()); 047 } 048 049 @Override 050 protected BaseHttpClientInvocation createClientInvocation(Object[] theArgs, IBaseResource theResource) { 051 IIdType idDt = (IIdType) theArgs[myIdParameterIndex]; 052 if (idDt == null) { 053 throw new NullPointerException(Msg.code(1447) + "ID can not be null"); 054 } 055 056 FhirContext context = getContext(); 057 058 HttpPutClientInvocation retVal = MethodUtil.createUpdateInvocation(theResource, null, idDt, context); 059 060 for (int idx = 0; idx < theArgs.length; idx++) { 061 IParameter nextParam = getParameters().get(idx); 062 nextParam.translateClientArgumentIntoQueryArgument(getContext(), theArgs[idx], null, null); 063 } 064 065 return retVal; 066 } 067 068 @Override 069 protected String getMatchingOperation() { 070 return null; 071 } 072 073 @Override 074 public RestOperationTypeEnum getRestOperationType() { 075 return RestOperationTypeEnum.UPDATE; 076 } 077 078 /* 079 * @Override public boolean incomingServerRequestMatchesMethod(RequestDetails theRequest) { if 080 * (super.incomingServerRequestMatchesMethod(theRequest)) { if (myVersionIdParameterIndex != null) { if 081 * (theRequest.getVersionId() == null) { return false; } } else { if (theRequest.getVersionId() != null) { return 082 * false; } } return true; } else { return false; } } 083 */ 084 085 @Override 086 protected Set<RequestTypeEnum> provideAllowableRequestTypes() { 087 return Collections.singleton(RequestTypeEnum.PUT); 088 } 089 090 @Override 091 protected void validateResourceIdAndUrlIdForNonConditionalOperation( 092 IBaseResource theResource, String theResourceId, String theUrlId, String theMatchUrl) { 093 if (isBlank(theMatchUrl)) { 094 if (isBlank(theUrlId)) { 095 String msg = getContext() 096 .getLocalizer() 097 .getMessage(BaseOutcomeReturningMethodBindingWithResourceParam.class, "noIdInUrlForUpdate"); 098 throw new InvalidRequestException(Msg.code(1448) + msg); 099 } 100 if (isBlank(theResourceId)) { 101 String msg = getContext() 102 .getLocalizer() 103 .getMessage(BaseOutcomeReturningMethodBindingWithResourceParam.class, "noIdInBodyForUpdate"); 104 throw new InvalidRequestException(Msg.code(1449) + msg); 105 } 106 if (!theResourceId.equals(theUrlId)) { 107 String msg = getContext() 108 .getLocalizer() 109 .getMessage( 110 BaseOutcomeReturningMethodBindingWithResourceParam.class, 111 "incorrectIdForUpdate", 112 theResourceId, 113 theUrlId); 114 throw new InvalidRequestException(Msg.code(1450) + msg); 115 } 116 } else { 117 theResource.setId((IIdType) null); 118 } 119 } 120}