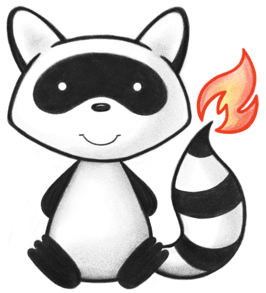
001/* 002 * #%L 003 * HAPI FHIR - Client Framework 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.client.method; 021 022import ca.uhn.fhir.context.FhirContext; 023import ca.uhn.fhir.model.valueset.BundleTypeEnum; 024import ca.uhn.fhir.rest.annotation.Validate; 025import ca.uhn.fhir.rest.api.Constants; 026import ca.uhn.fhir.rest.api.EncodingEnum; 027import ca.uhn.fhir.rest.client.impl.BaseHttpClientInvocation; 028import ca.uhn.fhir.util.ParametersUtil; 029import org.hl7.fhir.instance.model.api.IBaseParameters; 030import org.hl7.fhir.instance.model.api.IBaseResource; 031 032import java.lang.reflect.Method; 033import java.util.ArrayList; 034import java.util.List; 035 036public class ValidateMethodBindingDstu2Plus extends OperationMethodBinding { 037 038 public ValidateMethodBindingDstu2Plus( 039 Class<? extends IBaseResource> theReturnTypeFromRp, 040 Method theMethod, 041 FhirContext theContext, 042 Object theProvider, 043 Validate theAnnotation) { 044 super( 045 null, 046 theReturnTypeFromRp, 047 theMethod, 048 theContext, 049 theProvider, 050 true, 051 Constants.EXTOP_VALIDATE, 052 theAnnotation.type(), 053 BundleTypeEnum.COLLECTION); 054 055 List<IParameter> newParams = new ArrayList<>(); 056 int idx = 0; 057 for (IParameter next : getParameters()) { 058 if (next instanceof ResourceParameter) { 059 if (IBaseResource.class.isAssignableFrom(((ResourceParameter) next).getResourceType())) { 060 Class<?> parameterType = theMethod.getParameterTypes()[idx]; 061 if (String.class.equals(parameterType) || EncodingEnum.class.equals(parameterType)) { 062 newParams.add(next); 063 } else { 064 OperationParameter parameter = new OperationParameter( 065 theContext, Constants.EXTOP_VALIDATE, Constants.EXTOP_VALIDATE_RESOURCE, 0, 1); 066 parameter.initializeTypes(theMethod, null, null, parameterType); 067 newParams.add(parameter); 068 } 069 } else { 070 newParams.add(next); 071 } 072 } else { 073 newParams.add(next); 074 } 075 idx++; 076 } 077 setParameters(newParams); 078 } 079 080 public static BaseHttpClientInvocation createValidateInvocation(FhirContext theContext, IBaseResource theResource) { 081 IBaseParameters parameters = 082 (IBaseParameters) theContext.getResourceDefinition("Parameters").newInstance(); 083 ParametersUtil.addParameterToParameters(theContext, parameters, "resource", theResource); 084 085 String resourceName = theContext.getResourceType(theResource); 086 String resourceId = theResource.getIdElement().getIdPart(); 087 088 return createOperationInvocation( 089 theContext, resourceName, resourceId, null, Constants.EXTOP_VALIDATE, parameters, false); 090 } 091}