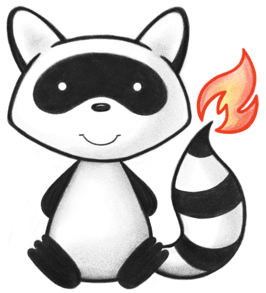
001package org.hl7.fhir.convertors.advisors.interfaces; 002 003import java.util.ArrayList; 004import java.util.List; 005 006import javax.annotation.Nonnull; 007 008import org.hl7.fhir.exceptions.FHIRException; 009import org.hl7.fhir.instance.model.api.IBaseExtension; 010import org.hl7.fhir.r4.model.Bundle; 011import org.hl7.fhir.r4.model.CodeSystem; 012import org.hl7.fhir.r4.model.Extension; 013import org.hl7.fhir.r4.model.Type; 014import org.hl7.fhir.r4.model.ValueSet; 015 016public abstract class BaseAdvisor40<T extends IBaseExtension> extends BaseAdvisor { 017 018 private final List<CodeSystem> cslist = new ArrayList<>(); 019 020 public final List<CodeSystem> getCslist() { 021 return this.cslist; 022 } 023 024 public void handleCodeSystem(@Nonnull CodeSystem tgtcs, 025 @Nonnull ValueSet source) { 026 tgtcs.setId(source.getId()); 027 tgtcs.setValueSet(source.getUrl()); 028 this.cslist.add(tgtcs); 029 } 030 031 public boolean ignoreEntry(@Nonnull Bundle.BundleEntryComponent src, 032 @Nonnull org.hl7.fhir.utilities.FhirPublication targetVersion) { 033 return false; 034 } 035 036 public CodeSystem getCodeSystem(@Nonnull ValueSet src) throws FHIRException { 037 return null; 038 } 039 040 public boolean ignoreExtension(@Nonnull String path, 041 @Nonnull Extension ext) throws FHIRException { 042 return ((ext.getUrl() != null) && (this.ignoreExtension(path, ext.getUrl())) 043 || (this.ignoreType(path, ext.getValue()))); 044 } 045 046 public boolean ignoreExtension(@Nonnull String path, 047 @Nonnull T ext) throws FHIRException { 048 return ((ext.getUrl() != null) && this.ignoreExtension(path, ext.getUrl())) 049 || (this.ignoreType(path, ext.getValue())); 050 } 051 052 public boolean ignoreExtension(@Nonnull String path, 053 @Nonnull String url) throws FHIRException { 054 return false; 055 } 056 057 public boolean ignoreType(@Nonnull String path, 058 @Nonnull Type type) throws FHIRException { 059 return false; 060 } 061 062 public boolean ignoreType(@Nonnull String path, 063 @Nonnull Object type) throws FHIRException { 064 return false; 065 } 066 067 public boolean useAdvisorForExtension(@Nonnull String path, 068 @Nonnull Extension ext) throws FHIRException { 069 return false; 070 } 071 072 public boolean useAdvisorForExtension(@Nonnull String path, 073 @Nonnull T ext) throws FHIRException { 074 return false; 075 } 076 077 public void handleExtension(@Nonnull String path, 078 @Nonnull Extension src, 079 @Nonnull T tgt) throws FHIRException { 080 } 081 082 public void handleExtension(@Nonnull String path, 083 @Nonnull T src, 084 @Nonnull Extension tgt) throws FHIRException { 085 } 086}