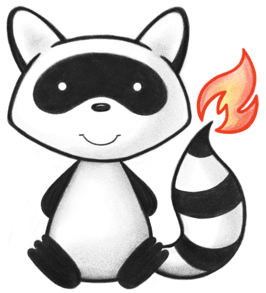
001package org.hl7.fhir.convertors.conv10_30.datatypes10_30; 002 003import java.util.List; 004import java.util.stream.Collectors; 005 006import org.hl7.fhir.convertors.context.ConversionContext10_30; 007import org.hl7.fhir.convertors.conv10_30.datatypes10_30.complextypes10_30.Coding10_30; 008import org.hl7.fhir.convertors.conv10_30.datatypes10_30.primitivetypes10_30.Boolean10_30; 009import org.hl7.fhir.convertors.conv10_30.datatypes10_30.primitivetypes10_30.Code10_30; 010import org.hl7.fhir.convertors.conv10_30.datatypes10_30.primitivetypes10_30.Id10_30; 011import org.hl7.fhir.convertors.conv10_30.datatypes10_30.primitivetypes10_30.Integer10_30; 012import org.hl7.fhir.convertors.conv10_30.datatypes10_30.primitivetypes10_30.MarkDown10_30; 013import org.hl7.fhir.convertors.conv10_30.datatypes10_30.primitivetypes10_30.String10_30; 014import org.hl7.fhir.dstu2.utils.ToolingExtensions; 015import org.hl7.fhir.dstu3.conformance.ProfileUtilities; 016import org.hl7.fhir.dstu3.model.ElementDefinition; 017import org.hl7.fhir.dstu3.model.Enumerations; 018import org.hl7.fhir.exceptions.FHIRException; 019 020public class ElementDefinition10_30 { 021 public static org.hl7.fhir.dstu3.model.ElementDefinition convertElementDefinition(org.hl7.fhir.dstu2.model.ElementDefinition src, List<String> slicePaths) throws FHIRException { 022 if (src == null || src.isEmpty()) return null; 023 org.hl7.fhir.dstu3.model.ElementDefinition tgt = new org.hl7.fhir.dstu3.model.ElementDefinition(); 024 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 025 if (src.hasPathElement()) tgt.setPathElement(String10_30.convertString(src.getPathElement())); 026 tgt.setRepresentation(src.getRepresentation().stream().map(ElementDefinition10_30::convertPropertyRepresentation).collect(Collectors.toList())); 027 if (src.hasName()) { 028 if (slicePaths.contains(src.getPath())) tgt.setSliceNameElement(String10_30.convertString(src.getNameElement())); 029 if (src.hasNameElement()) tgt.setIdElement(String10_30.convertString(src.getNameElement())); 030 } 031 if (src.hasLabel()) tgt.setLabelElement(String10_30.convertString(src.getLabelElement())); 032 for (org.hl7.fhir.dstu2.model.Coding t : src.getCode()) tgt.addCode(Coding10_30.convertCoding(t)); 033 if (src.hasSlicing()) tgt.setSlicing(convertElementDefinitionSlicingComponent(src.getSlicing())); 034 if (src.hasShort()) tgt.setShortElement(String10_30.convertString(src.getShortElement())); 035 if (src.hasDefinition()) tgt.setDefinitionElement(MarkDown10_30.convertMarkdown(src.getDefinitionElement())); 036 if (src.hasComments()) tgt.setCommentElement(MarkDown10_30.convertMarkdown(src.getCommentsElement())); 037 if (src.hasRequirements()) tgt.setRequirementsElement(MarkDown10_30.convertMarkdown(src.getRequirementsElement())); 038 for (org.hl7.fhir.dstu2.model.StringType t : src.getAlias()) tgt.addAlias(t.getValue()); 039 if (src.hasMin()) tgt.setMin(src.getMin()); 040 if (src.hasMax()) tgt.setMaxElement(String10_30.convertString(src.getMaxElement())); 041 if (src.hasBase()) tgt.setBase(convertElementDefinitionBaseComponent(src.getBase())); 042 if (src.hasNameReference()) tgt.setContentReference("#" + src.getNameReference()); 043 for (org.hl7.fhir.dstu2.model.ElementDefinition.TypeRefComponent t : src.getType()) 044 tgt.addType(convertElementDefinitionTypeComponent(t)); 045 if (src.hasDefaultValue()) 046 tgt.setDefaultValue(ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().convertType(src.getDefaultValue())); 047 if (src.hasMeaningWhenMissing()) 048 tgt.setMeaningWhenMissingElement(MarkDown10_30.convertMarkdown(src.getMeaningWhenMissingElement())); 049 if (src.hasFixed()) 050 tgt.setFixed(ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().convertType(src.getFixed())); 051 if (src.hasPattern()) 052 tgt.setPattern(ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().convertType(src.getPattern())); 053 if (src.hasExample()) 054 tgt.addExample().setLabel("General").setValue(ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().convertType(src.getExample())); 055 if (src.hasMinValue()) 056 tgt.setMinValue(ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().convertType(src.getMinValue())); 057 if (src.hasMaxValue()) 058 tgt.setMaxValue(ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().convertType(src.getMaxValue())); 059 if (src.hasMaxLength()) tgt.setMaxLengthElement(Integer10_30.convertInteger(src.getMaxLengthElement())); 060 for (org.hl7.fhir.dstu2.model.IdType t : src.getCondition()) tgt.addCondition(t.getValue()); 061 for (org.hl7.fhir.dstu2.model.ElementDefinition.ElementDefinitionConstraintComponent t : src.getConstraint()) 062 tgt.addConstraint(convertElementDefinitionConstraintComponent(t)); 063 if (src.hasMustSupport()) tgt.setMustSupportElement(Boolean10_30.convertBoolean(src.getMustSupportElement())); 064 if (src.hasIsModifier()) tgt.setIsModifierElement(Boolean10_30.convertBoolean(src.getIsModifierElement())); 065 if (src.hasIsSummary()) tgt.setIsSummaryElement(Boolean10_30.convertBoolean(src.getIsSummaryElement())); 066 if (src.hasBinding()) tgt.setBinding(convertElementDefinitionBindingComponent(src.getBinding())); 067 for (org.hl7.fhir.dstu2.model.ElementDefinition.ElementDefinitionMappingComponent t : src.getMapping()) 068 tgt.addMapping(convertElementDefinitionMappingComponent(t)); 069 return tgt; 070 } 071 072 public static org.hl7.fhir.dstu2.model.ElementDefinition convertElementDefinition(org.hl7.fhir.dstu3.model.ElementDefinition src) throws FHIRException { 073 if (src == null || src.isEmpty()) return null; 074 org.hl7.fhir.dstu2.model.ElementDefinition tgt = new org.hl7.fhir.dstu2.model.ElementDefinition(); 075 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 076 if (src.hasPathElement()) tgt.setPathElement(String10_30.convertString(src.getPathElement())); 077 tgt.setRepresentation(src.getRepresentation().stream().map(ElementDefinition10_30::convertPropertyRepresentation).collect(Collectors.toList())); 078 if (src.hasSliceName()) tgt.setNameElement(String10_30.convertString(src.getSliceNameElement())); 079 else tgt.setNameElement(String10_30.convertString(src.getIdElement())); 080 if (src.hasLabelElement()) tgt.setLabelElement(String10_30.convertString(src.getLabelElement())); 081 for (org.hl7.fhir.dstu3.model.Coding t : src.getCode()) tgt.addCode(Coding10_30.convertCoding(t)); 082 if (src.hasSlicing()) tgt.setSlicing(convertElementDefinitionSlicingComponent(src.getSlicing())); 083 if (src.hasShortElement()) tgt.setShortElement(String10_30.convertString(src.getShortElement())); 084 if (src.hasDefinitionElement()) tgt.setDefinitionElement(MarkDown10_30.convertMarkdown(src.getDefinitionElement())); 085 if (src.hasCommentElement()) tgt.setCommentsElement(MarkDown10_30.convertMarkdown(src.getCommentElement())); 086 if (src.hasRequirementsElement()) 087 tgt.setRequirementsElement(MarkDown10_30.convertMarkdown(src.getRequirementsElement())); 088 for (org.hl7.fhir.dstu3.model.StringType t : src.getAlias()) tgt.addAlias(t.getValue()); 089 tgt.setMin(src.getMin()); 090 if (src.hasMaxElement()) tgt.setMaxElement(String10_30.convertString(src.getMaxElement())); 091 if (src.hasBase()) tgt.setBase(convertElementDefinitionBaseComponent(src.getBase())); 092 if (src.hasContentReference()) tgt.setNameReference(src.getContentReference().substring(1)); 093 for (org.hl7.fhir.dstu3.model.ElementDefinition.TypeRefComponent t : src.getType()) 094 tgt.addType(convertElementDefinitionTypeComponent(t)); 095 if (src.hasDefaultValue()) 096 tgt.setDefaultValue(ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().convertType(src.getDefaultValue())); 097 if (src.hasMeaningWhenMissingElement()) 098 tgt.setMeaningWhenMissingElement(MarkDown10_30.convertMarkdown(src.getMeaningWhenMissingElement())); 099 if (src.hasFixed()) 100 tgt.setFixed(ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().convertType(src.getFixed())); 101 if (src.hasPattern()) 102 tgt.setPattern(ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().convertType(src.getPattern())); 103 if (src.hasExample()) 104 tgt.setExample(ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().convertType(src.getExampleFirstRep().getValue())); 105 if (src.hasMinValue()) 106 tgt.setMinValue(ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().convertType(src.getMinValue())); 107 if (src.hasMaxValue()) 108 tgt.setMaxValue(ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().convertType(src.getMaxValue())); 109 if (src.hasMaxLengthElement()) tgt.setMaxLengthElement(Integer10_30.convertInteger(src.getMaxLengthElement())); 110 for (org.hl7.fhir.dstu3.model.IdType t : src.getCondition()) tgt.addCondition(t.getValue()); 111 for (org.hl7.fhir.dstu3.model.ElementDefinition.ElementDefinitionConstraintComponent t : src.getConstraint()) 112 tgt.addConstraint(convertElementDefinitionConstraintComponent(t)); 113 if (src.hasMustSupportElement()) 114 tgt.setMustSupportElement(Boolean10_30.convertBoolean(src.getMustSupportElement())); 115 if (src.hasIsModifierElement()) tgt.setIsModifierElement(Boolean10_30.convertBoolean(src.getIsModifierElement())); 116 if (src.hasIsSummaryElement()) tgt.setIsSummaryElement(Boolean10_30.convertBoolean(src.getIsSummaryElement())); 117 if (src.hasBinding()) tgt.setBinding(convertElementDefinitionBindingComponent(src.getBinding())); 118 for (org.hl7.fhir.dstu3.model.ElementDefinition.ElementDefinitionMappingComponent t : src.getMapping()) 119 tgt.addMapping(convertElementDefinitionMappingComponent(t)); 120 return tgt; 121 } 122 123 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.ElementDefinition.PropertyRepresentation> convertPropertyRepresentation(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.ElementDefinition.PropertyRepresentation> src) throws FHIRException { 124 if (src == null || src.isEmpty()) return null; 125 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.ElementDefinition.PropertyRepresentation> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.ElementDefinition.PropertyRepresentationEnumFactory()); 126 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 127 if (src.getValue() == null) { 128 tgt.setValue(null); 129} else { 130 switch(src.getValue()) { 131 case XMLATTR: 132 tgt.setValue(ElementDefinition.PropertyRepresentation.XMLATTR); 133 break; 134 default: 135 tgt.setValue(ElementDefinition.PropertyRepresentation.NULL); 136 break; 137 } 138} 139 return tgt; 140 } 141 142 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.ElementDefinition.PropertyRepresentation> convertPropertyRepresentation(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.ElementDefinition.PropertyRepresentation> src) throws FHIRException { 143 if (src == null || src.isEmpty()) return null; 144 org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.ElementDefinition.PropertyRepresentation> tgt = new org.hl7.fhir.dstu2.model.Enumeration<>(new org.hl7.fhir.dstu2.model.ElementDefinition.PropertyRepresentationEnumFactory()); 145 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 146 if (src.getValue() == null) { 147 tgt.setValue(null); 148} else { 149 switch(src.getValue()) { 150 case XMLATTR: 151 tgt.setValue(org.hl7.fhir.dstu2.model.ElementDefinition.PropertyRepresentation.XMLATTR); 152 break; 153 default: 154 tgt.setValue(org.hl7.fhir.dstu2.model.ElementDefinition.PropertyRepresentation.NULL); 155 break; 156 } 157} 158 return tgt; 159 } 160 161 public static org.hl7.fhir.dstu3.model.ElementDefinition.ElementDefinitionSlicingComponent convertElementDefinitionSlicingComponent(org.hl7.fhir.dstu2.model.ElementDefinition.ElementDefinitionSlicingComponent src) throws FHIRException { 162 if (src == null || src.isEmpty()) return null; 163 org.hl7.fhir.dstu3.model.ElementDefinition.ElementDefinitionSlicingComponent tgt = new org.hl7.fhir.dstu3.model.ElementDefinition.ElementDefinitionSlicingComponent(); 164 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 165 for (org.hl7.fhir.dstu2.model.StringType t : src.getDiscriminator()) 166 tgt.addDiscriminator(ProfileUtilities.interpretR2Discriminator(t.getValue())); 167 if (src.hasDescriptionElement()) tgt.setDescriptionElement(String10_30.convertString(src.getDescriptionElement())); 168 if (src.hasOrderedElement()) tgt.setOrderedElement(Boolean10_30.convertBoolean(src.getOrderedElement())); 169 if (src.hasRules()) tgt.setRulesElement(convertSlicingRules(src.getRulesElement())); 170 return tgt; 171 } 172 173 public static org.hl7.fhir.dstu2.model.ElementDefinition.ElementDefinitionSlicingComponent convertElementDefinitionSlicingComponent(org.hl7.fhir.dstu3.model.ElementDefinition.ElementDefinitionSlicingComponent src) throws FHIRException { 174 if (src == null || src.isEmpty()) return null; 175 org.hl7.fhir.dstu2.model.ElementDefinition.ElementDefinitionSlicingComponent tgt = new org.hl7.fhir.dstu2.model.ElementDefinition.ElementDefinitionSlicingComponent(); 176 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 177 for (ElementDefinition.ElementDefinitionSlicingDiscriminatorComponent t : src.getDiscriminator()) 178 tgt.addDiscriminator(ProfileUtilities.buildR2Discriminator(t)); 179 if (src.hasDescriptionElement()) tgt.setDescriptionElement(String10_30.convertString(src.getDescriptionElement())); 180 if (src.hasOrderedElement()) tgt.setOrderedElement(Boolean10_30.convertBoolean(src.getOrderedElement())); 181 if (src.hasRules()) tgt.setRulesElement(convertSlicingRules(src.getRulesElement())); 182 return tgt; 183 } 184 185 static public org.hl7.fhir.dstu3.model.Enumeration<ElementDefinition.SlicingRules> convertSlicingRules(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.ElementDefinition.SlicingRules> src) throws FHIRException { 186 if (src == null || src.isEmpty()) return null; 187 org.hl7.fhir.dstu3.model.Enumeration<ElementDefinition.SlicingRules> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new ElementDefinition.SlicingRulesEnumFactory()); 188 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 189 if (src.getValue() == null) { 190 tgt.setValue(null); 191} else { 192 switch(src.getValue()) { 193 case CLOSED: 194 tgt.setValue(ElementDefinition.SlicingRules.CLOSED); 195 break; 196 case OPEN: 197 tgt.setValue(ElementDefinition.SlicingRules.OPEN); 198 break; 199 case OPENATEND: 200 tgt.setValue(ElementDefinition.SlicingRules.OPENATEND); 201 break; 202 default: 203 tgt.setValue(ElementDefinition.SlicingRules.NULL); 204 break; 205 } 206} 207 return tgt; 208 } 209 210 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.ElementDefinition.SlicingRules> convertSlicingRules(org.hl7.fhir.dstu3.model.Enumeration<ElementDefinition.SlicingRules> src) throws FHIRException { 211 if (src == null || src.isEmpty()) return null; 212 org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.ElementDefinition.SlicingRules> tgt = new org.hl7.fhir.dstu2.model.Enumeration<>(new org.hl7.fhir.dstu2.model.ElementDefinition.SlicingRulesEnumFactory()); 213 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 214 if (src.getValue() == null) { 215 tgt.setValue(null); 216} else { 217 switch(src.getValue()) { 218 case CLOSED: 219 tgt.setValue(org.hl7.fhir.dstu2.model.ElementDefinition.SlicingRules.CLOSED); 220 break; 221 case OPEN: 222 tgt.setValue(org.hl7.fhir.dstu2.model.ElementDefinition.SlicingRules.OPEN); 223 break; 224 case OPENATEND: 225 tgt.setValue(org.hl7.fhir.dstu2.model.ElementDefinition.SlicingRules.OPENATEND); 226 break; 227 default: 228 tgt.setValue(org.hl7.fhir.dstu2.model.ElementDefinition.SlicingRules.NULL); 229 break; 230 } 231} 232 return tgt; 233 } 234 235 public static ElementDefinition.ElementDefinitionBaseComponent convertElementDefinitionBaseComponent(org.hl7.fhir.dstu2.model.ElementDefinition.ElementDefinitionBaseComponent src) throws FHIRException { 236 if (src == null || src.isEmpty()) return null; 237 ElementDefinition.ElementDefinitionBaseComponent tgt = new ElementDefinition.ElementDefinitionBaseComponent(); 238 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 239 if (src.hasPathElement()) tgt.setPathElement(String10_30.convertString(src.getPathElement())); 240 if (src.hasMin()) tgt.setMin(src.getMin()); 241 if (src.hasMaxElement()) tgt.setMaxElement(String10_30.convertString(src.getMaxElement())); 242 return tgt; 243 } 244 245 public static org.hl7.fhir.dstu2.model.ElementDefinition.ElementDefinitionBaseComponent convertElementDefinitionBaseComponent(ElementDefinition.ElementDefinitionBaseComponent src) throws FHIRException { 246 if (src == null || src.isEmpty()) return null; 247 org.hl7.fhir.dstu2.model.ElementDefinition.ElementDefinitionBaseComponent tgt = new org.hl7.fhir.dstu2.model.ElementDefinition.ElementDefinitionBaseComponent(); 248 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 249 if (src.hasPathElement()) tgt.setPathElement(String10_30.convertString(src.getPathElement())); 250 if (src.hasMin()) tgt.setMin(src.getMin()); 251 if (src.hasMaxElement()) tgt.setMaxElement(String10_30.convertString(src.getMaxElement())); 252 return tgt; 253 } 254 255 public static ElementDefinition.TypeRefComponent convertElementDefinitionTypeComponent(org.hl7.fhir.dstu2.model.ElementDefinition.TypeRefComponent src) throws FHIRException { 256 if (src == null || src.isEmpty()) return null; 257 ElementDefinition.TypeRefComponent tgt = new ElementDefinition.TypeRefComponent(); 258 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 259 if (src.hasCodeElement()) tgt.setCodeElement(Code10_30.convertCodeToUri(src.getCodeElement())); 260 for (org.hl7.fhir.dstu2.model.UriType t : src.getProfile()) 261 if (src.hasTarget()) tgt.setTargetProfile(t.getValueAsString()); 262 else tgt.setProfile(t.getValue()); 263 tgt.setAggregation(src.getAggregation().stream().map(ElementDefinition10_30::convertAggregationMode).collect(Collectors.toList())); 264 return tgt; 265 } 266 267 public static org.hl7.fhir.dstu2.model.ElementDefinition.TypeRefComponent convertElementDefinitionTypeComponent(ElementDefinition.TypeRefComponent src) throws FHIRException { 268 if (src == null || src.isEmpty()) return null; 269 org.hl7.fhir.dstu2.model.ElementDefinition.TypeRefComponent tgt = new org.hl7.fhir.dstu2.model.ElementDefinition.TypeRefComponent(); 270 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 271 if (src.hasCodeElement()) tgt.setCodeElement(Code10_30.convertUriToCode(src.getCodeElement())); 272 if (src.hasTarget()) { 273 if (src.hasTargetProfile()) tgt.addProfile(src.getTargetProfile()); 274 } else if (src.hasProfile()) tgt.addProfile(src.getProfile()); 275 tgt.setAggregation(src.getAggregation().stream().map(ElementDefinition10_30::convertAggregationMode).collect(Collectors.toList())); 276 return tgt; 277 } 278 279 static public org.hl7.fhir.dstu3.model.Enumeration<ElementDefinition.AggregationMode> convertAggregationMode(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.ElementDefinition.AggregationMode> src) throws FHIRException { 280 if (src == null || src.isEmpty()) return null; 281 org.hl7.fhir.dstu3.model.Enumeration<ElementDefinition.AggregationMode> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new ElementDefinition.AggregationModeEnumFactory()); 282 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 283 if (src.getValue() == null) { 284 tgt.setValue(null); 285} else { 286 switch(src.getValue()) { 287 case CONTAINED: 288 tgt.setValue(ElementDefinition.AggregationMode.CONTAINED); 289 break; 290 case REFERENCED: 291 tgt.setValue(ElementDefinition.AggregationMode.REFERENCED); 292 break; 293 case BUNDLED: 294 tgt.setValue(ElementDefinition.AggregationMode.BUNDLED); 295 break; 296 default: 297 tgt.setValue(ElementDefinition.AggregationMode.NULL); 298 break; 299 } 300} 301 return tgt; 302 } 303 304 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.ElementDefinition.AggregationMode> convertAggregationMode(org.hl7.fhir.dstu3.model.Enumeration<ElementDefinition.AggregationMode> src) throws FHIRException { 305 if (src == null || src.isEmpty()) return null; 306 org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.ElementDefinition.AggregationMode> tgt = new org.hl7.fhir.dstu2.model.Enumeration<>(new org.hl7.fhir.dstu2.model.ElementDefinition.AggregationModeEnumFactory()); 307 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 308 if (src.getValue() == null) { 309 tgt.setValue(null); 310} else { 311 switch(src.getValue()) { 312 case CONTAINED: 313 tgt.setValue(org.hl7.fhir.dstu2.model.ElementDefinition.AggregationMode.CONTAINED); 314 break; 315 case REFERENCED: 316 tgt.setValue(org.hl7.fhir.dstu2.model.ElementDefinition.AggregationMode.REFERENCED); 317 break; 318 case BUNDLED: 319 tgt.setValue(org.hl7.fhir.dstu2.model.ElementDefinition.AggregationMode.BUNDLED); 320 break; 321 default: 322 tgt.setValue(org.hl7.fhir.dstu2.model.ElementDefinition.AggregationMode.NULL); 323 break; 324 } 325} 326 return tgt; 327 } 328 329 public static ElementDefinition.ElementDefinitionConstraintComponent convertElementDefinitionConstraintComponent(org.hl7.fhir.dstu2.model.ElementDefinition.ElementDefinitionConstraintComponent src) throws FHIRException { 330 if (src == null || src.isEmpty()) return null; 331 ElementDefinition.ElementDefinitionConstraintComponent tgt = new ElementDefinition.ElementDefinitionConstraintComponent(); 332 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 333 if (src.hasKeyElement()) tgt.setKeyElement(Id10_30.convertId(src.getKeyElement())); 334 if (src.hasRequirementsElement()) 335 tgt.setRequirementsElement(String10_30.convertString(src.getRequirementsElement())); 336 if (src.hasSeverity()) tgt.setSeverityElement(convertConstraintSeverity(src.getSeverityElement())); 337 if (src.hasHumanElement()) tgt.setHumanElement(String10_30.convertString(src.getHumanElement())); 338 tgt.setExpression(ToolingExtensions.readStringExtension(src, ToolingExtensions.EXT_EXPRESSION)); 339 if (src.hasXpathElement()) tgt.setXpathElement(String10_30.convertString(src.getXpathElement())); 340 return tgt; 341 } 342 343 public static org.hl7.fhir.dstu2.model.ElementDefinition.ElementDefinitionConstraintComponent convertElementDefinitionConstraintComponent(ElementDefinition.ElementDefinitionConstraintComponent src) throws FHIRException { 344 if (src == null || src.isEmpty()) return null; 345 org.hl7.fhir.dstu2.model.ElementDefinition.ElementDefinitionConstraintComponent tgt = new org.hl7.fhir.dstu2.model.ElementDefinition.ElementDefinitionConstraintComponent(); 346 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 347 if (src.hasKeyElement()) tgt.setKeyElement(Id10_30.convertId(src.getKeyElement())); 348 if (src.hasRequirementsElement()) 349 tgt.setRequirementsElement(String10_30.convertString(src.getRequirementsElement())); 350 if (src.hasSeverity()) tgt.setSeverityElement(convertConstraintSeverity(src.getSeverityElement())); 351 if (src.hasHumanElement()) tgt.setHumanElement(String10_30.convertString(src.getHumanElement())); 352 if (src.hasExpression()) 353 ToolingExtensions.addStringExtension(tgt, ToolingExtensions.EXT_EXPRESSION, src.getExpression()); 354 if (src.hasXpathElement()) tgt.setXpathElement(String10_30.convertString(src.getXpathElement())); 355 return tgt; 356 } 357 358 static public org.hl7.fhir.dstu3.model.Enumeration<ElementDefinition.ConstraintSeverity> convertConstraintSeverity(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.ElementDefinition.ConstraintSeverity> src) throws FHIRException { 359 if (src == null || src.isEmpty()) return null; 360 org.hl7.fhir.dstu3.model.Enumeration<ElementDefinition.ConstraintSeverity> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new ElementDefinition.ConstraintSeverityEnumFactory()); 361 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 362 if (src.getValue() == null) { 363 tgt.setValue(null); 364} else { 365 switch(src.getValue()) { 366 case ERROR: 367 tgt.setValue(ElementDefinition.ConstraintSeverity.ERROR); 368 break; 369 case WARNING: 370 tgt.setValue(ElementDefinition.ConstraintSeverity.WARNING); 371 break; 372 default: 373 tgt.setValue(ElementDefinition.ConstraintSeverity.NULL); 374 break; 375 } 376} 377 return tgt; 378 } 379 380 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.ElementDefinition.ConstraintSeverity> convertConstraintSeverity(org.hl7.fhir.dstu3.model.Enumeration<ElementDefinition.ConstraintSeverity> src) throws FHIRException { 381 if (src == null || src.isEmpty()) return null; 382 org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.ElementDefinition.ConstraintSeverity> tgt = new org.hl7.fhir.dstu2.model.Enumeration<>(new org.hl7.fhir.dstu2.model.ElementDefinition.ConstraintSeverityEnumFactory()); 383 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 384 if (src.getValue() == null) { 385 tgt.setValue(null); 386} else { 387 switch(src.getValue()) { 388 case ERROR: 389 tgt.setValue(org.hl7.fhir.dstu2.model.ElementDefinition.ConstraintSeverity.ERROR); 390 break; 391 case WARNING: 392 tgt.setValue(org.hl7.fhir.dstu2.model.ElementDefinition.ConstraintSeverity.WARNING); 393 break; 394 default: 395 tgt.setValue(org.hl7.fhir.dstu2.model.ElementDefinition.ConstraintSeverity.NULL); 396 break; 397 } 398} 399 return tgt; 400 } 401 402 public static ElementDefinition.ElementDefinitionBindingComponent convertElementDefinitionBindingComponent(org.hl7.fhir.dstu2.model.ElementDefinition.ElementDefinitionBindingComponent src) throws FHIRException { 403 if (src == null || src.isEmpty()) return null; 404 ElementDefinition.ElementDefinitionBindingComponent tgt = new ElementDefinition.ElementDefinitionBindingComponent(); 405 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 406 if (src.hasStrength()) tgt.setStrengthElement(convertBindingStrength(src.getStrengthElement())); 407 if (src.hasDescriptionElement()) tgt.setDescriptionElement(String10_30.convertString(src.getDescriptionElement())); 408 if (src.hasValueSet()) 409 tgt.setValueSet(ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().convertType(src.getValueSet())); 410 return tgt; 411 } 412 413 public static org.hl7.fhir.dstu2.model.ElementDefinition.ElementDefinitionBindingComponent convertElementDefinitionBindingComponent(ElementDefinition.ElementDefinitionBindingComponent src) throws FHIRException { 414 if (src == null || src.isEmpty()) return null; 415 org.hl7.fhir.dstu2.model.ElementDefinition.ElementDefinitionBindingComponent tgt = new org.hl7.fhir.dstu2.model.ElementDefinition.ElementDefinitionBindingComponent(); 416 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 417 if (src.hasStrength()) tgt.setStrengthElement(convertBindingStrength(src.getStrengthElement())); 418 if (src.hasDescriptionElement()) tgt.setDescriptionElement(String10_30.convertString(src.getDescriptionElement())); 419 if (src.hasValueSet()) 420 tgt.setValueSet(ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().convertType(src.getValueSet())); 421 return tgt; 422 } 423 424 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Enumerations.BindingStrength> convertBindingStrength(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Enumerations.BindingStrength> src) throws FHIRException { 425 if (src == null || src.isEmpty()) return null; 426 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Enumerations.BindingStrength> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.Enumerations.BindingStrengthEnumFactory()); 427 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 428 if (src.getValue() == null) { 429 tgt.setValue(null); 430} else { 431 switch(src.getValue()) { 432 case REQUIRED: 433 tgt.setValue(Enumerations.BindingStrength.REQUIRED); 434 break; 435 case EXTENSIBLE: 436 tgt.setValue(Enumerations.BindingStrength.EXTENSIBLE); 437 break; 438 case PREFERRED: 439 tgt.setValue(Enumerations.BindingStrength.PREFERRED); 440 break; 441 case EXAMPLE: 442 tgt.setValue(Enumerations.BindingStrength.EXAMPLE); 443 break; 444 default: 445 tgt.setValue(Enumerations.BindingStrength.NULL); 446 break; 447 } 448} 449 return tgt; 450 } 451 452 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Enumerations.BindingStrength> convertBindingStrength(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Enumerations.BindingStrength> src) throws FHIRException { 453 if (src == null || src.isEmpty()) return null; 454 org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Enumerations.BindingStrength> tgt = new org.hl7.fhir.dstu2.model.Enumeration<>(new org.hl7.fhir.dstu2.model.Enumerations.BindingStrengthEnumFactory()); 455 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 456 if (src.getValue() == null) { 457 tgt.setValue(null); 458} else { 459 switch(src.getValue()) { 460 case REQUIRED: 461 tgt.setValue(org.hl7.fhir.dstu2.model.Enumerations.BindingStrength.REQUIRED); 462 break; 463 case EXTENSIBLE: 464 tgt.setValue(org.hl7.fhir.dstu2.model.Enumerations.BindingStrength.EXTENSIBLE); 465 break; 466 case PREFERRED: 467 tgt.setValue(org.hl7.fhir.dstu2.model.Enumerations.BindingStrength.PREFERRED); 468 break; 469 case EXAMPLE: 470 tgt.setValue(org.hl7.fhir.dstu2.model.Enumerations.BindingStrength.EXAMPLE); 471 break; 472 default: 473 tgt.setValue(org.hl7.fhir.dstu2.model.Enumerations.BindingStrength.NULL); 474 break; 475 } 476} 477 return tgt; 478 } 479 480 public static ElementDefinition.ElementDefinitionMappingComponent convertElementDefinitionMappingComponent(org.hl7.fhir.dstu2.model.ElementDefinition.ElementDefinitionMappingComponent src) throws FHIRException { 481 if (src == null || src.isEmpty()) return null; 482 ElementDefinition.ElementDefinitionMappingComponent tgt = new ElementDefinition.ElementDefinitionMappingComponent(); 483 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 484 if (src.hasIdentityElement()) tgt.setIdentityElement(Id10_30.convertId(src.getIdentityElement())); 485 if (src.hasLanguageElement()) tgt.setLanguageElement(Code10_30.convertCode(src.getLanguageElement())); 486 if (src.hasMapElement()) tgt.setMapElement(String10_30.convertString(src.getMapElement())); 487 return tgt; 488 } 489 490 public static org.hl7.fhir.dstu2.model.ElementDefinition.ElementDefinitionMappingComponent convertElementDefinitionMappingComponent(ElementDefinition.ElementDefinitionMappingComponent src) throws FHIRException { 491 if (src == null || src.isEmpty()) return null; 492 org.hl7.fhir.dstu2.model.ElementDefinition.ElementDefinitionMappingComponent tgt = new org.hl7.fhir.dstu2.model.ElementDefinition.ElementDefinitionMappingComponent(); 493 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 494 if (src.hasIdentityElement()) tgt.setIdentityElement(Id10_30.convertId(src.getIdentityElement())); 495 if (src.hasLanguageElement()) tgt.setLanguageElement(Code10_30.convertCode(src.getLanguageElement())); 496 if (src.hasMapElement()) tgt.setMapElement(String10_30.convertString(src.getMapElement())); 497 return tgt; 498 } 499}