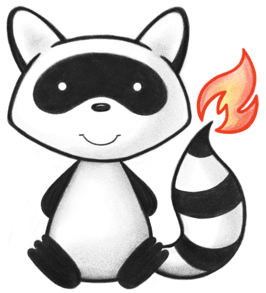
001package org.hl7.fhir.convertors.conv10_30.datatypes10_30.complextypes10_30; 002 003import org.hl7.fhir.convertors.context.ConversionContext10_30; 004import org.hl7.fhir.convertors.conv10_30.datatypes10_30.primitivetypes10_30.String10_30; 005import org.hl7.fhir.exceptions.FHIRException; 006 007public class HumanName10_30 { 008 public static org.hl7.fhir.dstu3.model.HumanName convertHumanName(org.hl7.fhir.dstu2.model.HumanName src) throws FHIRException { 009 if (src == null || src.isEmpty()) return null; 010 org.hl7.fhir.dstu3.model.HumanName tgt = new org.hl7.fhir.dstu3.model.HumanName(); 011 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 012 if (src.hasUse()) tgt.setUseElement(convertNameUse(src.getUseElement())); 013 if (src.hasTextElement()) tgt.setTextElement(String10_30.convertString(src.getTextElement())); 014 for (org.hl7.fhir.dstu2.model.StringType t : src.getFamily()) tgt.setFamily(t.getValue()); 015 for (org.hl7.fhir.dstu2.model.StringType t : src.getGiven()) tgt.addGiven(t.getValue()); 016 for (org.hl7.fhir.dstu2.model.StringType t : src.getPrefix()) tgt.addPrefix(t.getValue()); 017 for (org.hl7.fhir.dstu2.model.StringType t : src.getSuffix()) tgt.addSuffix(t.getValue()); 018 if (src.hasPeriod()) tgt.setPeriod(Period10_30.convertPeriod(src.getPeriod())); 019 return tgt; 020 } 021 022 public static org.hl7.fhir.dstu2.model.HumanName convertHumanName(org.hl7.fhir.dstu3.model.HumanName src) throws FHIRException { 023 if (src == null || src.isEmpty()) return null; 024 org.hl7.fhir.dstu2.model.HumanName tgt = new org.hl7.fhir.dstu2.model.HumanName(); 025 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 026 if (src.hasUse()) tgt.setUseElement(convertNameUse(src.getUseElement())); 027 if (src.hasTextElement()) tgt.setTextElement(String10_30.convertString(src.getTextElement())); 028 if (src.hasFamily()) tgt.addFamily(src.getFamily()); 029 for (org.hl7.fhir.dstu3.model.StringType t : src.getGiven()) tgt.addGiven(t.getValue()); 030 for (org.hl7.fhir.dstu3.model.StringType t : src.getPrefix()) tgt.addPrefix(t.getValue()); 031 for (org.hl7.fhir.dstu3.model.StringType t : src.getSuffix()) tgt.addSuffix(t.getValue()); 032 if (src.hasPeriod()) tgt.setPeriod(Period10_30.convertPeriod(src.getPeriod())); 033 return tgt; 034 } 035 036 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.HumanName.NameUse> convertNameUse(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.HumanName.NameUse> src) throws FHIRException { 037 if (src == null || src.isEmpty()) return null; 038 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.HumanName.NameUse> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.HumanName.NameUseEnumFactory()); 039 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 040 if (src.getValue() == null) { 041 tgt.setValue(org.hl7.fhir.dstu3.model.HumanName.NameUse.NULL); 042 } else { 043 switch (src.getValue()) { 044 case USUAL: 045 tgt.setValue(org.hl7.fhir.dstu3.model.HumanName.NameUse.USUAL); 046 break; 047 case OFFICIAL: 048 tgt.setValue(org.hl7.fhir.dstu3.model.HumanName.NameUse.OFFICIAL); 049 break; 050 case TEMP: 051 tgt.setValue(org.hl7.fhir.dstu3.model.HumanName.NameUse.TEMP); 052 break; 053 case NICKNAME: 054 tgt.setValue(org.hl7.fhir.dstu3.model.HumanName.NameUse.NICKNAME); 055 break; 056 case ANONYMOUS: 057 tgt.setValue(org.hl7.fhir.dstu3.model.HumanName.NameUse.ANONYMOUS); 058 break; 059 case OLD: 060 tgt.setValue(org.hl7.fhir.dstu3.model.HumanName.NameUse.OLD); 061 break; 062 case MAIDEN: 063 tgt.setValue(org.hl7.fhir.dstu3.model.HumanName.NameUse.MAIDEN); 064 break; 065 default: 066 tgt.setValue(org.hl7.fhir.dstu3.model.HumanName.NameUse.NULL); 067 break; 068 } 069 } 070 return tgt; 071 } 072 073 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.HumanName.NameUse> convertNameUse(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.HumanName.NameUse> src) throws FHIRException { 074 if (src == null || src.isEmpty()) return null; 075 org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.HumanName.NameUse> tgt = new org.hl7.fhir.dstu2.model.Enumeration<>(new org.hl7.fhir.dstu2.model.HumanName.NameUseEnumFactory()); 076 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 077 if (src.getValue() == null) { 078 tgt.setValue(org.hl7.fhir.dstu2.model.HumanName.NameUse.NULL); 079 } else { 080 switch (src.getValue()) { 081 case USUAL: 082 tgt.setValue(org.hl7.fhir.dstu2.model.HumanName.NameUse.USUAL); 083 break; 084 case OFFICIAL: 085 tgt.setValue(org.hl7.fhir.dstu2.model.HumanName.NameUse.OFFICIAL); 086 break; 087 case TEMP: 088 tgt.setValue(org.hl7.fhir.dstu2.model.HumanName.NameUse.TEMP); 089 break; 090 case NICKNAME: 091 tgt.setValue(org.hl7.fhir.dstu2.model.HumanName.NameUse.NICKNAME); 092 break; 093 case ANONYMOUS: 094 tgt.setValue(org.hl7.fhir.dstu2.model.HumanName.NameUse.ANONYMOUS); 095 break; 096 case OLD: 097 tgt.setValue(org.hl7.fhir.dstu2.model.HumanName.NameUse.OLD); 098 break; 099 case MAIDEN: 100 tgt.setValue(org.hl7.fhir.dstu2.model.HumanName.NameUse.MAIDEN); 101 break; 102 default: 103 tgt.setValue(org.hl7.fhir.dstu2.model.HumanName.NameUse.NULL); 104 break; 105 } 106 } 107 return tgt; 108 } 109}