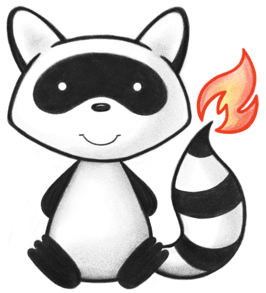
001package org.hl7.fhir.convertors.conv10_30.datatypes10_30.complextypes10_30; 002 003import org.hl7.fhir.convertors.context.ConversionContext10_30; 004import org.hl7.fhir.convertors.conv10_30.datatypes10_30.Reference10_30; 005import org.hl7.fhir.convertors.conv10_30.datatypes10_30.primitivetypes10_30.String10_30; 006import org.hl7.fhir.convertors.conv10_30.datatypes10_30.primitivetypes10_30.Uri10_30; 007import org.hl7.fhir.exceptions.FHIRException; 008 009public class Identifier10_30 { 010 public static org.hl7.fhir.dstu3.model.Identifier convertIdentifier(org.hl7.fhir.dstu2.model.Identifier src) throws FHIRException { 011 if (src == null || src.isEmpty()) return null; 012 org.hl7.fhir.dstu3.model.Identifier tgt = new org.hl7.fhir.dstu3.model.Identifier(); 013 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 014 if (src.hasUse()) tgt.setUseElement(convertIdentifierUse(src.getUseElement())); 015 if (src.hasType()) tgt.setType(CodeableConcept10_30.convertCodeableConcept(src.getType())); 016 if (src.hasSystemElement()) tgt.setSystemElement(Uri10_30.convertUri(src.getSystemElement())); 017 if (src.hasValueElement()) tgt.setValueElement(String10_30.convertString(src.getValueElement())); 018 if (src.hasPeriod()) tgt.setPeriod(Period10_30.convertPeriod(src.getPeriod())); 019 if (src.hasAssigner()) tgt.setAssigner(Reference10_30.convertReference(src.getAssigner())); 020 return tgt; 021 } 022 023 public static org.hl7.fhir.dstu2.model.Identifier convertIdentifier(org.hl7.fhir.dstu3.model.Identifier src) throws FHIRException { 024 if (src == null || src.isEmpty()) return null; 025 org.hl7.fhir.dstu2.model.Identifier tgt = new org.hl7.fhir.dstu2.model.Identifier(); 026 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 027 if (src.hasUse()) tgt.setUseElement(convertIdentifierUse(src.getUseElement())); 028 if (src.hasType()) tgt.setType(CodeableConcept10_30.convertCodeableConcept(src.getType())); 029 if (src.hasSystem()) tgt.setSystemElement(Uri10_30.convertUri(src.getSystemElement())); 030 if (src.hasValue()) tgt.setValueElement(String10_30.convertString(src.getValueElement())); 031 if (src.hasPeriod()) tgt.setPeriod(Period10_30.convertPeriod(src.getPeriod())); 032 if (src.hasAssigner()) tgt.setAssigner(Reference10_30.convertReference(src.getAssigner())); 033 return tgt; 034 } 035 036 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Identifier.IdentifierUse> convertIdentifierUse(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Identifier.IdentifierUse> src) throws FHIRException { 037 if (src == null || src.isEmpty()) return null; 038 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Identifier.IdentifierUse> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.Identifier.IdentifierUseEnumFactory()); 039 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 040 if (src.getValue() == null) { 041 tgt.setValue(org.hl7.fhir.dstu3.model.Identifier.IdentifierUse.NULL); 042 } else { 043 switch (src.getValue()) { 044 case USUAL: 045 tgt.setValue(org.hl7.fhir.dstu3.model.Identifier.IdentifierUse.USUAL); 046 break; 047 case OFFICIAL: 048 tgt.setValue(org.hl7.fhir.dstu3.model.Identifier.IdentifierUse.OFFICIAL); 049 break; 050 case TEMP: 051 tgt.setValue(org.hl7.fhir.dstu3.model.Identifier.IdentifierUse.TEMP); 052 break; 053 case SECONDARY: 054 tgt.setValue(org.hl7.fhir.dstu3.model.Identifier.IdentifierUse.SECONDARY); 055 break; 056 default: 057 tgt.setValue(org.hl7.fhir.dstu3.model.Identifier.IdentifierUse.NULL); 058 break; 059 } 060 } 061 return tgt; 062 } 063 064 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Identifier.IdentifierUse> convertIdentifierUse(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Identifier.IdentifierUse> src) throws FHIRException { 065 if (src == null || src.isEmpty()) return null; 066 org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Identifier.IdentifierUse> tgt = new org.hl7.fhir.dstu2.model.Enumeration<>(new org.hl7.fhir.dstu2.model.Identifier.IdentifierUseEnumFactory()); 067 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 068 if (src.getValue() == null) { 069 tgt.setValue(org.hl7.fhir.dstu2.model.Identifier.IdentifierUse.NULL); 070 } else { 071 switch (src.getValue()) { 072 case USUAL: 073 tgt.setValue(org.hl7.fhir.dstu2.model.Identifier.IdentifierUse.USUAL); 074 break; 075 case OFFICIAL: 076 tgt.setValue(org.hl7.fhir.dstu2.model.Identifier.IdentifierUse.OFFICIAL); 077 break; 078 case TEMP: 079 tgt.setValue(org.hl7.fhir.dstu2.model.Identifier.IdentifierUse.TEMP); 080 break; 081 case SECONDARY: 082 tgt.setValue(org.hl7.fhir.dstu2.model.Identifier.IdentifierUse.SECONDARY); 083 break; 084 default: 085 tgt.setValue(org.hl7.fhir.dstu2.model.Identifier.IdentifierUse.NULL); 086 break; 087 } 088 } 089 return tgt; 090 } 091 092}