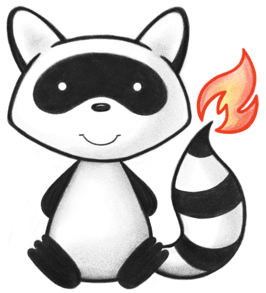
001package org.hl7.fhir.convertors.conv10_30.resources10_30; 002 003import org.hl7.fhir.convertors.context.ConversionContext10_30; 004import org.hl7.fhir.convertors.conv10_30.datatypes10_30.Reference10_30; 005import org.hl7.fhir.convertors.conv10_30.datatypes10_30.complextypes10_30.CodeableConcept10_30; 006import org.hl7.fhir.convertors.conv10_30.datatypes10_30.complextypes10_30.Identifier10_30; 007import org.hl7.fhir.convertors.conv10_30.datatypes10_30.complextypes10_30.Money10_30; 008import org.hl7.fhir.convertors.conv10_30.datatypes10_30.complextypes10_30.Period10_30; 009import org.hl7.fhir.convertors.conv10_30.datatypes10_30.primitivetypes10_30.String10_30; 010import org.hl7.fhir.dstu3.model.Account; 011import org.hl7.fhir.dstu3.model.Enumeration; 012import org.hl7.fhir.exceptions.FHIRException; 013 014public class Account10_30 { 015 016 public static org.hl7.fhir.dstu2.model.Account convertAccount(org.hl7.fhir.dstu3.model.Account src) throws FHIRException { 017 if (src == null || src.isEmpty()) 018 return null; 019 org.hl7.fhir.dstu2.model.Account tgt = new org.hl7.fhir.dstu2.model.Account(); 020 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyDomainResource(src, tgt); 021 for (org.hl7.fhir.dstu3.model.Identifier t : src.getIdentifier()) 022 tgt.addIdentifier(Identifier10_30.convertIdentifier(t)); 023 if (src.hasNameElement()) 024 tgt.setNameElement(String10_30.convertString(src.getNameElement())); 025 if (src.hasType()) 026 tgt.setType(CodeableConcept10_30.convertCodeableConcept(src.getType())); 027 if (src.hasStatus()) 028 tgt.setStatusElement(convertAccountStatus(src.getStatusElement())); 029 if (src.hasActive()) 030 tgt.setActivePeriod(Period10_30.convertPeriod(src.getActive())); 031 if (src.hasBalance()) 032 tgt.setBalance(Money10_30.convertMoney(src.getBalance())); 033 if (src.hasSubject()) 034 tgt.setSubject(Reference10_30.convertReference(src.getSubject())); 035 if (src.hasOwner()) 036 tgt.setOwner(Reference10_30.convertReference(src.getOwner())); 037 if (src.hasDescriptionElement()) 038 tgt.setDescriptionElement(String10_30.convertString(src.getDescriptionElement())); 039 return tgt; 040 } 041 042 public static org.hl7.fhir.dstu3.model.Account convertAccount(org.hl7.fhir.dstu2.model.Account src) throws FHIRException { 043 if (src == null || src.isEmpty()) 044 return null; 045 org.hl7.fhir.dstu3.model.Account tgt = new org.hl7.fhir.dstu3.model.Account(); 046 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyDomainResource(src, tgt); 047 for (org.hl7.fhir.dstu2.model.Identifier t : src.getIdentifier()) 048 tgt.addIdentifier(Identifier10_30.convertIdentifier(t)); 049 if (src.hasName()) 050 tgt.setNameElement(String10_30.convertString(src.getNameElement())); 051 if (src.hasType()) 052 tgt.setType(CodeableConcept10_30.convertCodeableConcept(src.getType())); 053 if (src.hasStatus()) 054 tgt.setStatusElement(convertAccountStatus(src.getStatusElement())); 055 if (src.hasActivePeriod()) 056 tgt.setActive(Period10_30.convertPeriod(src.getActivePeriod())); 057 if (src.hasBalance()) 058 tgt.setBalance(Money10_30.convertMoney(src.getBalance())); 059 if (src.hasSubject()) 060 tgt.setSubject(Reference10_30.convertReference(src.getSubject())); 061 if (src.hasOwner()) 062 tgt.setOwner(Reference10_30.convertReference(src.getOwner())); 063 if (src.hasDescriptionElement()) 064 tgt.setDescriptionElement(String10_30.convertString(src.getDescriptionElement())); 065 return tgt; 066 } 067 068 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Account.AccountStatus> convertAccountStatus(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Account.AccountStatus> src) throws FHIRException { 069 if (src == null || src.isEmpty()) 070 return null; 071 Enumeration<Account.AccountStatus> tgt = new Enumeration<>(new Account.AccountStatusEnumFactory()); 072 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 073 if (src.getValue() == null) { 074 tgt.setValue(null); 075 } else { 076 switch (src.getValue()) { 077 case ACTIVE: 078 tgt.setValue(Account.AccountStatus.ACTIVE); 079 break; 080 case INACTIVE: 081 tgt.setValue(Account.AccountStatus.INACTIVE); 082 break; 083 default: 084 tgt.setValue(Account.AccountStatus.NULL); 085 break; 086 } 087 } 088 return tgt; 089 } 090 091 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Account.AccountStatus> convertAccountStatus(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Account.AccountStatus> src) throws FHIRException { 092 if (src == null || src.isEmpty()) 093 return null; 094 org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Account.AccountStatus> tgt = new org.hl7.fhir.dstu2.model.Enumeration<>(new org.hl7.fhir.dstu2.model.Account.AccountStatusEnumFactory()); 095 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 096 if (src.getValue() == null) { 097 tgt.setValue(null); 098 } else { 099 switch (src.getValue()) { 100 case ACTIVE: 101 tgt.setValue(org.hl7.fhir.dstu2.model.Account.AccountStatus.ACTIVE); 102 break; 103 case INACTIVE: 104 tgt.setValue(org.hl7.fhir.dstu2.model.Account.AccountStatus.INACTIVE); 105 break; 106 default: 107 tgt.setValue(org.hl7.fhir.dstu2.model.Account.AccountStatus.NULL); 108 break; 109 } 110 } 111 return tgt; 112 } 113}