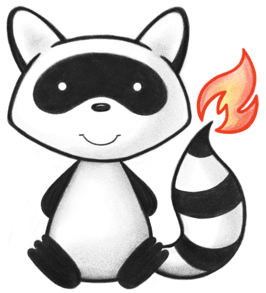
001package org.hl7.fhir.convertors.conv10_30.resources10_30; 002 003import org.hl7.fhir.convertors.context.ConversionContext10_30; 004import org.hl7.fhir.convertors.conv10_30.datatypes10_30.Reference10_30; 005import org.hl7.fhir.convertors.conv10_30.datatypes10_30.complextypes10_30.CodeableConcept10_30; 006import org.hl7.fhir.convertors.conv10_30.datatypes10_30.complextypes10_30.Coding10_30; 007import org.hl7.fhir.convertors.conv10_30.datatypes10_30.complextypes10_30.Identifier10_30; 008import org.hl7.fhir.convertors.conv10_30.datatypes10_30.primitivetypes10_30.Base64Binary10_30; 009import org.hl7.fhir.convertors.conv10_30.datatypes10_30.primitivetypes10_30.Boolean10_30; 010import org.hl7.fhir.convertors.conv10_30.datatypes10_30.primitivetypes10_30.String10_30; 011import org.hl7.fhir.dstu3.model.AuditEvent; 012import org.hl7.fhir.dstu3.model.Enumeration; 013import org.hl7.fhir.exceptions.FHIRException; 014 015public class AuditEvent10_30 { 016 017 public static org.hl7.fhir.dstu2.model.AuditEvent convertAuditEvent(org.hl7.fhir.dstu3.model.AuditEvent src) throws FHIRException { 018 if (src == null || src.isEmpty()) 019 return null; 020 org.hl7.fhir.dstu2.model.AuditEvent tgt = new org.hl7.fhir.dstu2.model.AuditEvent(); 021 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyDomainResource(src, tgt); 022 tgt.getEvent().setType(Coding10_30.convertCoding(src.getType())); 023 for (org.hl7.fhir.dstu3.model.Coding t : src.getSubtype()) tgt.getEvent().addSubtype(Coding10_30.convertCoding(t)); 024 tgt.getEvent().setActionElement(convertAuditEventAction(src.getActionElement())); 025 tgt.getEvent().setDateTime(src.getRecorded()); 026 tgt.getEvent().setOutcomeElement(convertAuditEventOutcome(src.getOutcomeElement())); 027 tgt.getEvent().setOutcomeDesc(src.getOutcomeDesc()); 028 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getPurposeOfEvent()) 029 for (org.hl7.fhir.dstu3.model.Coding cc : t.getCoding()) 030 tgt.getEvent().addPurposeOfEvent(Coding10_30.convertCoding(cc)); 031 for (org.hl7.fhir.dstu3.model.AuditEvent.AuditEventAgentComponent t : src.getAgent()) 032 tgt.addParticipant(convertAuditEventAgentComponent(t)); 033 if (src.hasSource()) 034 tgt.setSource(convertAuditEventSourceComponent(src.getSource())); 035 for (org.hl7.fhir.dstu3.model.AuditEvent.AuditEventEntityComponent t : src.getEntity()) 036 tgt.addObject(convertAuditEventEntityComponent(t)); 037 return tgt; 038 } 039 040 public static org.hl7.fhir.dstu3.model.AuditEvent convertAuditEvent(org.hl7.fhir.dstu2.model.AuditEvent src) throws FHIRException { 041 if (src == null || src.isEmpty()) 042 return null; 043 org.hl7.fhir.dstu3.model.AuditEvent tgt = new org.hl7.fhir.dstu3.model.AuditEvent(); 044 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyDomainResource(src, tgt); 045 if (src.hasEvent()) { 046 if (src.hasType()) 047 tgt.setType(Coding10_30.convertCoding(src.getEvent().getType())); 048 for (org.hl7.fhir.dstu2.model.Coding t : src.getEvent().getSubtype()) 049 tgt.addSubtype(Coding10_30.convertCoding(t)); 050 tgt.setActionElement(convertAuditEventAction(src.getEvent().getActionElement())); 051 tgt.setRecorded(src.getEvent().getDateTime()); 052 tgt.setOutcomeElement(convertAuditEventOutcome(src.getEvent().getOutcomeElement())); 053 tgt.setOutcomeDesc(src.getEvent().getOutcomeDesc()); 054 for (org.hl7.fhir.dstu2.model.Coding t : src.getEvent().getPurposeOfEvent()) 055 tgt.addPurposeOfEvent().addCoding(Coding10_30.convertCoding(t)); 056 } 057 for (org.hl7.fhir.dstu2.model.AuditEvent.AuditEventParticipantComponent t : src.getParticipant()) 058 tgt.addAgent(convertAuditEventAgentComponent(t)); 059 if (src.hasSource()) 060 tgt.setSource(convertAuditEventSourceComponent(src.getSource())); 061 for (org.hl7.fhir.dstu2.model.AuditEvent.AuditEventObjectComponent t : src.getObject()) 062 tgt.addEntity(convertAuditEventEntityComponent(t)); 063 return tgt; 064 } 065 066 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.AuditEvent.AuditEventAction> convertAuditEventAction(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.AuditEvent.AuditEventAction> src) throws FHIRException { 067 if (src == null || src.isEmpty()) 068 return null; 069 Enumeration<AuditEvent.AuditEventAction> tgt = new Enumeration<>(new AuditEvent.AuditEventActionEnumFactory()); 070 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 071 if (src.getValue() == null) { 072 tgt.setValue(null); 073 } else { 074 switch (src.getValue()) { 075 case C: 076 tgt.setValue(AuditEvent.AuditEventAction.C); 077 break; 078 case R: 079 tgt.setValue(AuditEvent.AuditEventAction.R); 080 break; 081 case U: 082 tgt.setValue(AuditEvent.AuditEventAction.U); 083 break; 084 case D: 085 tgt.setValue(AuditEvent.AuditEventAction.D); 086 break; 087 case E: 088 tgt.setValue(AuditEvent.AuditEventAction.E); 089 break; 090 default: 091 tgt.setValue(AuditEvent.AuditEventAction.NULL); 092 break; 093 } 094 } 095 return tgt; 096 } 097 098 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.AuditEvent.AuditEventAction> convertAuditEventAction(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.AuditEvent.AuditEventAction> src) throws FHIRException { 099 if (src == null || src.isEmpty()) 100 return null; 101 org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.AuditEvent.AuditEventAction> tgt = new org.hl7.fhir.dstu2.model.Enumeration<>(new org.hl7.fhir.dstu2.model.AuditEvent.AuditEventActionEnumFactory()); 102 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 103 if (src.getValue() == null) { 104 tgt.setValue(null); 105 } else { 106 switch (src.getValue()) { 107 case C: 108 tgt.setValue(org.hl7.fhir.dstu2.model.AuditEvent.AuditEventAction.C); 109 break; 110 case R: 111 tgt.setValue(org.hl7.fhir.dstu2.model.AuditEvent.AuditEventAction.R); 112 break; 113 case U: 114 tgt.setValue(org.hl7.fhir.dstu2.model.AuditEvent.AuditEventAction.U); 115 break; 116 case D: 117 tgt.setValue(org.hl7.fhir.dstu2.model.AuditEvent.AuditEventAction.D); 118 break; 119 case E: 120 tgt.setValue(org.hl7.fhir.dstu2.model.AuditEvent.AuditEventAction.E); 121 break; 122 default: 123 tgt.setValue(org.hl7.fhir.dstu2.model.AuditEvent.AuditEventAction.NULL); 124 break; 125 } 126 } 127 return tgt; 128 } 129 130 public static org.hl7.fhir.dstu3.model.AuditEvent.AuditEventAgentComponent convertAuditEventAgentComponent(org.hl7.fhir.dstu2.model.AuditEvent.AuditEventParticipantComponent src) throws FHIRException { 131 if (src == null || src.isEmpty()) 132 return null; 133 org.hl7.fhir.dstu3.model.AuditEvent.AuditEventAgentComponent tgt = new org.hl7.fhir.dstu3.model.AuditEvent.AuditEventAgentComponent(); 134 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 135 for (org.hl7.fhir.dstu2.model.CodeableConcept t : src.getRole()) 136 tgt.addRole(CodeableConcept10_30.convertCodeableConcept(t)); 137 if (src.hasReference()) 138 tgt.setReference(Reference10_30.convertReference(src.getReference())); 139 if (src.hasUserId()) 140 tgt.setUserId(Identifier10_30.convertIdentifier(src.getUserId())); 141 if (src.hasAltIdElement()) 142 tgt.setAltIdElement(String10_30.convertString(src.getAltIdElement())); 143 if (src.hasNameElement()) 144 tgt.setNameElement(String10_30.convertString(src.getNameElement())); 145 if (src.hasRequestorElement()) 146 tgt.setRequestorElement(Boolean10_30.convertBoolean(src.getRequestorElement())); 147 if (src.hasLocation()) 148 tgt.setLocation(Reference10_30.convertReference(src.getLocation())); 149 for (org.hl7.fhir.dstu2.model.UriType t : src.getPolicy()) tgt.addPolicy(t.getValue()); 150 if (src.hasMedia()) 151 tgt.setMedia(Coding10_30.convertCoding(src.getMedia())); 152 if (src.hasNetwork()) 153 tgt.setNetwork(convertAuditEventAgentNetworkComponent(src.getNetwork())); 154 for (org.hl7.fhir.dstu2.model.Coding t : src.getPurposeOfUse()) 155 tgt.addPurposeOfUse().addCoding(Coding10_30.convertCoding(t)); 156 return tgt; 157 } 158 159 public static org.hl7.fhir.dstu2.model.AuditEvent.AuditEventParticipantComponent convertAuditEventAgentComponent(org.hl7.fhir.dstu3.model.AuditEvent.AuditEventAgentComponent src) throws FHIRException { 160 if (src == null || src.isEmpty()) 161 return null; 162 org.hl7.fhir.dstu2.model.AuditEvent.AuditEventParticipantComponent tgt = new org.hl7.fhir.dstu2.model.AuditEvent.AuditEventParticipantComponent(); 163 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 164 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getRole()) 165 tgt.addRole(CodeableConcept10_30.convertCodeableConcept(t)); 166 if (src.hasReference()) 167 tgt.setReference(Reference10_30.convertReference(src.getReference())); 168 if (src.hasUserId()) 169 tgt.setUserId(Identifier10_30.convertIdentifier(src.getUserId())); 170 if (src.hasAltIdElement()) 171 tgt.setAltIdElement(String10_30.convertString(src.getAltIdElement())); 172 if (src.hasNameElement()) 173 tgt.setNameElement(String10_30.convertString(src.getNameElement())); 174 if (src.hasRequestorElement()) 175 tgt.setRequestorElement(Boolean10_30.convertBoolean(src.getRequestorElement())); 176 if (src.hasLocation()) 177 tgt.setLocation(Reference10_30.convertReference(src.getLocation())); 178 for (org.hl7.fhir.dstu3.model.UriType t : src.getPolicy()) tgt.addPolicy(t.getValue()); 179 if (src.hasMedia()) 180 tgt.setMedia(Coding10_30.convertCoding(src.getMedia())); 181 if (src.hasNetwork()) 182 tgt.setNetwork(convertAuditEventAgentNetworkComponent(src.getNetwork())); 183 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getPurposeOfUse()) 184 for (org.hl7.fhir.dstu3.model.Coding cc : t.getCoding()) tgt.addPurposeOfUse(Coding10_30.convertCoding(cc)); 185 return tgt; 186 } 187 188 public static org.hl7.fhir.dstu2.model.AuditEvent.AuditEventParticipantNetworkComponent convertAuditEventAgentNetworkComponent(org.hl7.fhir.dstu3.model.AuditEvent.AuditEventAgentNetworkComponent src) throws FHIRException { 189 if (src == null || src.isEmpty()) 190 return null; 191 org.hl7.fhir.dstu2.model.AuditEvent.AuditEventParticipantNetworkComponent tgt = new org.hl7.fhir.dstu2.model.AuditEvent.AuditEventParticipantNetworkComponent(); 192 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 193 if (src.hasAddressElement()) 194 tgt.setAddressElement(String10_30.convertString(src.getAddressElement())); 195 if (src.hasType()) 196 tgt.setTypeElement(convertAuditEventParticipantNetworkType(src.getTypeElement())); 197 return tgt; 198 } 199 200 public static org.hl7.fhir.dstu3.model.AuditEvent.AuditEventAgentNetworkComponent convertAuditEventAgentNetworkComponent(org.hl7.fhir.dstu2.model.AuditEvent.AuditEventParticipantNetworkComponent src) throws FHIRException { 201 if (src == null || src.isEmpty()) 202 return null; 203 org.hl7.fhir.dstu3.model.AuditEvent.AuditEventAgentNetworkComponent tgt = new org.hl7.fhir.dstu3.model.AuditEvent.AuditEventAgentNetworkComponent(); 204 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 205 if (src.hasAddressElement()) 206 tgt.setAddressElement(String10_30.convertString(src.getAddressElement())); 207 if (src.hasType()) 208 tgt.setTypeElement(convertAuditEventParticipantNetworkType(src.getTypeElement())); 209 return tgt; 210 } 211 212 public static org.hl7.fhir.dstu3.model.AuditEvent.AuditEventEntityComponent convertAuditEventEntityComponent(org.hl7.fhir.dstu2.model.AuditEvent.AuditEventObjectComponent src) throws FHIRException { 213 if (src == null || src.isEmpty()) 214 return null; 215 org.hl7.fhir.dstu3.model.AuditEvent.AuditEventEntityComponent tgt = new org.hl7.fhir.dstu3.model.AuditEvent.AuditEventEntityComponent(); 216 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 217 if (src.hasIdentifier()) 218 tgt.setIdentifier(Identifier10_30.convertIdentifier(src.getIdentifier())); 219 if (src.hasReference()) 220 tgt.setReference(Reference10_30.convertReference(src.getReference())); 221 if (src.hasType()) 222 tgt.setType(Coding10_30.convertCoding(src.getType())); 223 if (src.hasRole()) 224 tgt.setRole(Coding10_30.convertCoding(src.getRole())); 225 if (src.hasLifecycle()) 226 tgt.setLifecycle(Coding10_30.convertCoding(src.getLifecycle())); 227 for (org.hl7.fhir.dstu2.model.Coding t : src.getSecurityLabel()) tgt.addSecurityLabel(Coding10_30.convertCoding(t)); 228 if (src.hasNameElement()) 229 tgt.setNameElement(String10_30.convertString(src.getNameElement())); 230 if (src.hasDescriptionElement()) 231 tgt.setDescriptionElement(String10_30.convertString(src.getDescriptionElement())); 232 if (src.hasQueryElement()) 233 tgt.setQueryElement(Base64Binary10_30.convertBase64Binary(src.getQueryElement())); 234 for (org.hl7.fhir.dstu2.model.AuditEvent.AuditEventObjectDetailComponent t : src.getDetail()) 235 tgt.addDetail(convertAuditEventEntityDetailComponent(t)); 236 return tgt; 237 } 238 239 public static org.hl7.fhir.dstu2.model.AuditEvent.AuditEventObjectComponent convertAuditEventEntityComponent(org.hl7.fhir.dstu3.model.AuditEvent.AuditEventEntityComponent src) throws FHIRException { 240 if (src == null || src.isEmpty()) 241 return null; 242 org.hl7.fhir.dstu2.model.AuditEvent.AuditEventObjectComponent tgt = new org.hl7.fhir.dstu2.model.AuditEvent.AuditEventObjectComponent(); 243 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 244 if (src.hasIdentifier()) 245 tgt.setIdentifier(Identifier10_30.convertIdentifier(src.getIdentifier())); 246 if (src.hasReference()) 247 tgt.setReference(Reference10_30.convertReference(src.getReference())); 248 if (src.hasType()) 249 tgt.setType(Coding10_30.convertCoding(src.getType())); 250 if (src.hasRole()) 251 tgt.setRole(Coding10_30.convertCoding(src.getRole())); 252 if (src.hasLifecycle()) 253 tgt.setLifecycle(Coding10_30.convertCoding(src.getLifecycle())); 254 for (org.hl7.fhir.dstu3.model.Coding t : src.getSecurityLabel()) tgt.addSecurityLabel(Coding10_30.convertCoding(t)); 255 if (src.hasNameElement()) 256 tgt.setNameElement(String10_30.convertString(src.getNameElement())); 257 if (src.hasDescriptionElement()) 258 tgt.setDescriptionElement(String10_30.convertString(src.getDescriptionElement())); 259 if (src.hasQueryElement()) 260 tgt.setQueryElement(Base64Binary10_30.convertBase64Binary(src.getQueryElement())); 261 for (org.hl7.fhir.dstu3.model.AuditEvent.AuditEventEntityDetailComponent t : src.getDetail()) 262 tgt.addDetail(convertAuditEventEntityDetailComponent(t)); 263 return tgt; 264 } 265 266 public static org.hl7.fhir.dstu2.model.AuditEvent.AuditEventObjectDetailComponent convertAuditEventEntityDetailComponent(org.hl7.fhir.dstu3.model.AuditEvent.AuditEventEntityDetailComponent src) throws FHIRException { 267 if (src == null || src.isEmpty()) 268 return null; 269 org.hl7.fhir.dstu2.model.AuditEvent.AuditEventObjectDetailComponent tgt = new org.hl7.fhir.dstu2.model.AuditEvent.AuditEventObjectDetailComponent(); 270 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 271 if (src.hasTypeElement()) 272 tgt.setTypeElement(String10_30.convertString(src.getTypeElement())); 273 if (src.hasValueElement()) 274 tgt.setValueElement(Base64Binary10_30.convertBase64Binary(src.getValueElement())); 275 return tgt; 276 } 277 278 public static org.hl7.fhir.dstu3.model.AuditEvent.AuditEventEntityDetailComponent convertAuditEventEntityDetailComponent(org.hl7.fhir.dstu2.model.AuditEvent.AuditEventObjectDetailComponent src) throws FHIRException { 279 if (src == null || src.isEmpty()) 280 return null; 281 org.hl7.fhir.dstu3.model.AuditEvent.AuditEventEntityDetailComponent tgt = new org.hl7.fhir.dstu3.model.AuditEvent.AuditEventEntityDetailComponent(); 282 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 283 if (src.hasTypeElement()) 284 tgt.setTypeElement(String10_30.convertString(src.getTypeElement())); 285 if (src.hasValueElement()) 286 tgt.setValueElement(Base64Binary10_30.convertBase64Binary(src.getValueElement())); 287 return tgt; 288 } 289 290 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.AuditEvent.AuditEventOutcome> convertAuditEventOutcome(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.AuditEvent.AuditEventOutcome> src) throws FHIRException { 291 if (src == null || src.isEmpty()) 292 return null; 293 Enumeration<AuditEvent.AuditEventOutcome> tgt = new Enumeration<>(new AuditEvent.AuditEventOutcomeEnumFactory()); 294 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 295 if (src.getValue() == null) { 296 tgt.setValue(null); 297 } else { 298 switch (src.getValue()) { 299 case _0: 300 tgt.setValue(AuditEvent.AuditEventOutcome._0); 301 break; 302 case _4: 303 tgt.setValue(AuditEvent.AuditEventOutcome._4); 304 break; 305 case _8: 306 tgt.setValue(AuditEvent.AuditEventOutcome._8); 307 break; 308 case _12: 309 tgt.setValue(AuditEvent.AuditEventOutcome._12); 310 break; 311 default: 312 tgt.setValue(AuditEvent.AuditEventOutcome.NULL); 313 break; 314 } 315 } 316 return tgt; 317 } 318 319 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.AuditEvent.AuditEventOutcome> convertAuditEventOutcome(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.AuditEvent.AuditEventOutcome> src) throws FHIRException { 320 if (src == null || src.isEmpty()) 321 return null; 322 org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.AuditEvent.AuditEventOutcome> tgt = new org.hl7.fhir.dstu2.model.Enumeration<>(new org.hl7.fhir.dstu2.model.AuditEvent.AuditEventOutcomeEnumFactory()); 323 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 324 if (src.getValue() == null) { 325 tgt.setValue(null); 326 } else { 327 switch (src.getValue()) { 328 case _0: 329 tgt.setValue(org.hl7.fhir.dstu2.model.AuditEvent.AuditEventOutcome._0); 330 break; 331 case _4: 332 tgt.setValue(org.hl7.fhir.dstu2.model.AuditEvent.AuditEventOutcome._4); 333 break; 334 case _8: 335 tgt.setValue(org.hl7.fhir.dstu2.model.AuditEvent.AuditEventOutcome._8); 336 break; 337 case _12: 338 tgt.setValue(org.hl7.fhir.dstu2.model.AuditEvent.AuditEventOutcome._12); 339 break; 340 default: 341 tgt.setValue(org.hl7.fhir.dstu2.model.AuditEvent.AuditEventOutcome.NULL); 342 break; 343 } 344 } 345 return tgt; 346 } 347 348 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.AuditEvent.AuditEventParticipantNetworkType> convertAuditEventParticipantNetworkType(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.AuditEvent.AuditEventAgentNetworkType> src) throws FHIRException { 349 if (src == null || src.isEmpty()) 350 return null; 351 org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.AuditEvent.AuditEventParticipantNetworkType> tgt = new org.hl7.fhir.dstu2.model.Enumeration<>(new org.hl7.fhir.dstu2.model.AuditEvent.AuditEventParticipantNetworkTypeEnumFactory()); 352 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 353 if (src.getValue() == null) { 354 tgt.setValue(null); 355 } else { 356 switch (src.getValue()) { 357 case _1: 358 tgt.setValue(org.hl7.fhir.dstu2.model.AuditEvent.AuditEventParticipantNetworkType._1); 359 break; 360 case _2: 361 tgt.setValue(org.hl7.fhir.dstu2.model.AuditEvent.AuditEventParticipantNetworkType._2); 362 break; 363 case _3: 364 tgt.setValue(org.hl7.fhir.dstu2.model.AuditEvent.AuditEventParticipantNetworkType._3); 365 break; 366 case _4: 367 tgt.setValue(org.hl7.fhir.dstu2.model.AuditEvent.AuditEventParticipantNetworkType._4); 368 break; 369 case _5: 370 tgt.setValue(org.hl7.fhir.dstu2.model.AuditEvent.AuditEventParticipantNetworkType._5); 371 break; 372 default: 373 tgt.setValue(org.hl7.fhir.dstu2.model.AuditEvent.AuditEventParticipantNetworkType.NULL); 374 break; 375 } 376 } 377 return tgt; 378 } 379 380 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.AuditEvent.AuditEventAgentNetworkType> convertAuditEventParticipantNetworkType(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.AuditEvent.AuditEventParticipantNetworkType> src) throws FHIRException { 381 if (src == null || src.isEmpty()) 382 return null; 383 Enumeration<AuditEvent.AuditEventAgentNetworkType> tgt = new Enumeration<>(new AuditEvent.AuditEventAgentNetworkTypeEnumFactory()); 384 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 385 if (src.getValue() == null) { 386 tgt.setValue(null); 387 } else { 388 switch (src.getValue()) { 389 case _1: 390 tgt.setValue(AuditEvent.AuditEventAgentNetworkType._1); 391 break; 392 case _2: 393 tgt.setValue(AuditEvent.AuditEventAgentNetworkType._2); 394 break; 395 case _3: 396 tgt.setValue(AuditEvent.AuditEventAgentNetworkType._3); 397 break; 398 case _4: 399 tgt.setValue(AuditEvent.AuditEventAgentNetworkType._4); 400 break; 401 case _5: 402 tgt.setValue(AuditEvent.AuditEventAgentNetworkType._5); 403 break; 404 default: 405 tgt.setValue(AuditEvent.AuditEventAgentNetworkType.NULL); 406 break; 407 } 408 } 409 return tgt; 410 } 411 412 public static org.hl7.fhir.dstu2.model.AuditEvent.AuditEventSourceComponent convertAuditEventSourceComponent(org.hl7.fhir.dstu3.model.AuditEvent.AuditEventSourceComponent src) throws FHIRException { 413 if (src == null || src.isEmpty()) 414 return null; 415 org.hl7.fhir.dstu2.model.AuditEvent.AuditEventSourceComponent tgt = new org.hl7.fhir.dstu2.model.AuditEvent.AuditEventSourceComponent(); 416 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 417 if (src.hasSiteElement()) 418 tgt.setSiteElement(String10_30.convertString(src.getSiteElement())); 419 if (src.hasIdentifier()) 420 tgt.setIdentifier(Identifier10_30.convertIdentifier(src.getIdentifier())); 421 for (org.hl7.fhir.dstu3.model.Coding t : src.getType()) tgt.addType(Coding10_30.convertCoding(t)); 422 return tgt; 423 } 424 425 public static org.hl7.fhir.dstu3.model.AuditEvent.AuditEventSourceComponent convertAuditEventSourceComponent(org.hl7.fhir.dstu2.model.AuditEvent.AuditEventSourceComponent src) throws FHIRException { 426 if (src == null || src.isEmpty()) 427 return null; 428 org.hl7.fhir.dstu3.model.AuditEvent.AuditEventSourceComponent tgt = new org.hl7.fhir.dstu3.model.AuditEvent.AuditEventSourceComponent(); 429 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 430 if (src.hasSiteElement()) 431 tgt.setSiteElement(String10_30.convertString(src.getSiteElement())); 432 if (src.hasIdentifier()) 433 tgt.setIdentifier(Identifier10_30.convertIdentifier(src.getIdentifier())); 434 for (org.hl7.fhir.dstu2.model.Coding t : src.getType()) tgt.addType(Coding10_30.convertCoding(t)); 435 return tgt; 436 } 437}