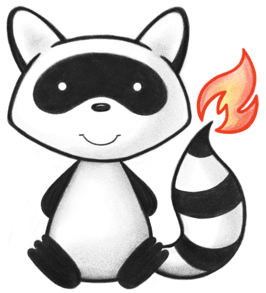
001package org.hl7.fhir.convertors.conv10_30.resources10_30; 002 003import org.hl7.fhir.convertors.advisors.impl.BaseAdvisor_10_30; 004import org.hl7.fhir.convertors.context.ConversionContext10_30; 005import org.hl7.fhir.convertors.conv10_30.datatypes10_30.complextypes10_30.Signature10_30; 006import org.hl7.fhir.convertors.conv10_30.datatypes10_30.primitivetypes10_30.Decimal10_30; 007import org.hl7.fhir.convertors.conv10_30.datatypes10_30.primitivetypes10_30.Instant10_30; 008import org.hl7.fhir.convertors.conv10_30.datatypes10_30.primitivetypes10_30.String10_30; 009import org.hl7.fhir.convertors.conv10_30.datatypes10_30.primitivetypes10_30.UnsignedInt10_30; 010import org.hl7.fhir.convertors.conv10_30.datatypes10_30.primitivetypes10_30.Uri10_30; 011import org.hl7.fhir.exceptions.FHIRException; 012import org.hl7.fhir.utilities.FhirPublication; 013 014public class Bundle10_30 { 015 016 public static org.hl7.fhir.dstu3.model.Bundle convertBundle(org.hl7.fhir.dstu2.model.Bundle src) throws FHIRException { 017 if (src == null || src.isEmpty()) 018 return null; 019 org.hl7.fhir.dstu3.model.Bundle tgt = new org.hl7.fhir.dstu3.model.Bundle(); 020 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyResource(src, tgt); 021 if (src.hasType()) 022 tgt.setTypeElement(convertBundleType(src.getTypeElement())); 023 if (src.hasTotal()) 024 tgt.setTotalElement(UnsignedInt10_30.convertUnsignedInt(src.getTotalElement())); 025 for (org.hl7.fhir.dstu2.model.Bundle.BundleLinkComponent t : src.getLink()) 026 tgt.addLink(convertBundleLinkComponent(t)); 027 for (org.hl7.fhir.dstu2.model.Bundle.BundleEntryComponent t : src.getEntry()) 028 tgt.addEntry(convertBundleEntryComponent(t)); 029 if (src.hasSignature()) 030 tgt.setSignature(Signature10_30.convertSignature(src.getSignature())); 031 return tgt; 032 } 033 034 public static org.hl7.fhir.dstu2.model.Bundle convertBundle(org.hl7.fhir.dstu3.model.Bundle src, BaseAdvisor_10_30 advisor) throws FHIRException { 035 if (src == null || src.isEmpty()) 036 return null; 037 org.hl7.fhir.dstu2.model.Bundle tgt = new org.hl7.fhir.dstu2.model.Bundle(); 038 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyResource(src, tgt); 039 if (src.hasType()) 040 tgt.setTypeElement(convertBundleType(src.getTypeElement())); 041 if (src.hasTotal()) 042 tgt.setTotalElement(UnsignedInt10_30.convertUnsignedInt(src.getTotalElement())); 043 for (org.hl7.fhir.dstu3.model.Bundle.BundleLinkComponent t : src.getLink()) 044 tgt.addLink(convertBundleLinkComponent(t)); 045 for (org.hl7.fhir.dstu3.model.Bundle.BundleEntryComponent t : src.getEntry()) 046 tgt.addEntry(convertBundleEntryComponent(t, advisor)); 047 if (src.hasSignature()) 048 tgt.setSignature(Signature10_30.convertSignature(src.getSignature())); 049 return tgt; 050 } 051 052 public static org.hl7.fhir.dstu2.model.Bundle convertBundle(org.hl7.fhir.dstu3.model.Bundle src) throws FHIRException { 053 return convertBundle(src, null); 054 } 055 056 public static org.hl7.fhir.dstu2.model.Bundle.BundleEntryComponent convertBundleEntryComponent(org.hl7.fhir.dstu3.model.Bundle.BundleEntryComponent src, BaseAdvisor_10_30 advisor) throws FHIRException { 057 if (src == null || src.isEmpty()) 058 return null; 059 if (advisor.ignoreEntry(src, FhirPublication.DSTU2)) 060 return null; 061 org.hl7.fhir.dstu2.model.Bundle.BundleEntryComponent tgt = new org.hl7.fhir.dstu2.model.Bundle.BundleEntryComponent(); 062 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 063 for (org.hl7.fhir.dstu3.model.Bundle.BundleLinkComponent t : src.getLink()) 064 tgt.addLink(convertBundleLinkComponent(t)); 065 if (src.hasFullUrlElement()) 066 tgt.setFullUrlElement(Uri10_30.convertUri(src.getFullUrlElement())); 067 if (src.hasResource()) 068 tgt.setResource(ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().convertResource(src.getResource())); 069 if (src.hasSearch()) 070 tgt.setSearch(convertBundleEntrySearchComponent(src.getSearch())); 071 if (src.hasRequest()) 072 tgt.setRequest(convertBundleEntryRequestComponent(src.getRequest())); 073 if (src.hasResponse()) 074 tgt.setResponse(convertBundleEntryResponseComponent(src.getResponse())); 075 return tgt; 076 } 077 078 public static org.hl7.fhir.dstu2.model.Bundle.BundleEntryComponent convertBundleEntryComponent(org.hl7.fhir.dstu3.model.Bundle.BundleEntryComponent src) throws FHIRException { 079 return convertBundleEntryComponent(src, null); 080 } 081 082 public static org.hl7.fhir.dstu3.model.Bundle.BundleEntryComponent convertBundleEntryComponent(org.hl7.fhir.dstu2.model.Bundle.BundleEntryComponent src) throws FHIRException { 083 if (src == null || src.isEmpty()) 084 return null; 085 org.hl7.fhir.dstu3.model.Bundle.BundleEntryComponent tgt = new org.hl7.fhir.dstu3.model.Bundle.BundleEntryComponent(); 086 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 087 for (org.hl7.fhir.dstu2.model.Bundle.BundleLinkComponent t : src.getLink()) 088 tgt.addLink(convertBundleLinkComponent(t)); 089 if (src.hasFullUrlElement()) 090 tgt.setFullUrlElement(Uri10_30.convertUri(src.getFullUrlElement())); 091 if (src.hasResource()) 092 tgt.setResource(ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().convertResource(src.getResource())); 093 if (src.hasSearch()) 094 tgt.setSearch(convertBundleEntrySearchComponent(src.getSearch())); 095 if (src.hasRequest()) 096 tgt.setRequest(convertBundleEntryRequestComponent(src.getRequest())); 097 if (src.hasResponse()) 098 tgt.setResponse(convertBundleEntryResponseComponent(src.getResponse())); 099 return tgt; 100 } 101 102 public static org.hl7.fhir.dstu2.model.Bundle.BundleEntryRequestComponent convertBundleEntryRequestComponent(org.hl7.fhir.dstu3.model.Bundle.BundleEntryRequestComponent src) throws FHIRException { 103 if (src == null || src.isEmpty()) 104 return null; 105 org.hl7.fhir.dstu2.model.Bundle.BundleEntryRequestComponent tgt = new org.hl7.fhir.dstu2.model.Bundle.BundleEntryRequestComponent(); 106 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 107 if (src.hasMethod()) 108 tgt.setMethodElement(convertHTTPVerb(src.getMethodElement())); 109 if (src.hasUrlElement()) 110 tgt.setUrlElement(Uri10_30.convertUri(src.getUrlElement())); 111 if (src.hasIfNoneMatchElement()) 112 tgt.setIfNoneMatchElement(String10_30.convertString(src.getIfNoneMatchElement())); 113 if (src.hasIfModifiedSinceElement()) 114 tgt.setIfModifiedSinceElement(Instant10_30.convertInstant(src.getIfModifiedSinceElement())); 115 if (src.hasIfMatchElement()) 116 tgt.setIfMatchElement(String10_30.convertString(src.getIfMatchElement())); 117 if (src.hasIfNoneExistElement()) 118 tgt.setIfNoneExistElement(String10_30.convertString(src.getIfNoneExistElement())); 119 return tgt; 120 } 121 122 public static org.hl7.fhir.dstu3.model.Bundle.BundleEntryRequestComponent convertBundleEntryRequestComponent(org.hl7.fhir.dstu2.model.Bundle.BundleEntryRequestComponent src) throws FHIRException { 123 if (src == null || src.isEmpty()) 124 return null; 125 org.hl7.fhir.dstu3.model.Bundle.BundleEntryRequestComponent tgt = new org.hl7.fhir.dstu3.model.Bundle.BundleEntryRequestComponent(); 126 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 127 if (src.hasMethod()) 128 tgt.setMethodElement(convertHTTPVerb(src.getMethodElement())); 129 if (src.hasUrlElement()) 130 tgt.setUrlElement(Uri10_30.convertUri(src.getUrlElement())); 131 if (src.hasIfNoneMatchElement()) 132 tgt.setIfNoneMatchElement(String10_30.convertString(src.getIfNoneMatchElement())); 133 if (src.hasIfModifiedSinceElement()) 134 tgt.setIfModifiedSinceElement(Instant10_30.convertInstant(src.getIfModifiedSinceElement())); 135 if (src.hasIfMatchElement()) 136 tgt.setIfMatchElement(String10_30.convertString(src.getIfMatchElement())); 137 if (src.hasIfNoneExistElement()) 138 tgt.setIfNoneExistElement(String10_30.convertString(src.getIfNoneExistElement())); 139 return tgt; 140 } 141 142 public static org.hl7.fhir.dstu2.model.Bundle.BundleEntryResponseComponent convertBundleEntryResponseComponent(org.hl7.fhir.dstu3.model.Bundle.BundleEntryResponseComponent src) throws FHIRException { 143 if (src == null || src.isEmpty()) 144 return null; 145 org.hl7.fhir.dstu2.model.Bundle.BundleEntryResponseComponent tgt = new org.hl7.fhir.dstu2.model.Bundle.BundleEntryResponseComponent(); 146 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 147 if (src.hasStatusElement()) 148 tgt.setStatusElement(String10_30.convertString(src.getStatusElement())); 149 if (src.hasLocationElement()) 150 tgt.setLocationElement(Uri10_30.convertUri(src.getLocationElement())); 151 if (src.hasEtagElement()) 152 tgt.setEtagElement(String10_30.convertString(src.getEtagElement())); 153 if (src.hasLastModifiedElement()) 154 tgt.setLastModifiedElement(Instant10_30.convertInstant(src.getLastModifiedElement())); 155 return tgt; 156 } 157 158 public static org.hl7.fhir.dstu3.model.Bundle.BundleEntryResponseComponent convertBundleEntryResponseComponent(org.hl7.fhir.dstu2.model.Bundle.BundleEntryResponseComponent src) throws FHIRException { 159 if (src == null || src.isEmpty()) 160 return null; 161 org.hl7.fhir.dstu3.model.Bundle.BundleEntryResponseComponent tgt = new org.hl7.fhir.dstu3.model.Bundle.BundleEntryResponseComponent(); 162 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 163 if (src.hasStatusElement()) 164 tgt.setStatusElement(String10_30.convertString(src.getStatusElement())); 165 if (src.hasLocationElement()) 166 tgt.setLocationElement(Uri10_30.convertUri(src.getLocationElement())); 167 if (src.hasEtagElement()) 168 tgt.setEtagElement(String10_30.convertString(src.getEtagElement())); 169 if (src.hasLastModifiedElement()) 170 tgt.setLastModifiedElement(Instant10_30.convertInstant(src.getLastModifiedElement())); 171 return tgt; 172 } 173 174 public static org.hl7.fhir.dstu2.model.Bundle.BundleEntrySearchComponent convertBundleEntrySearchComponent(org.hl7.fhir.dstu3.model.Bundle.BundleEntrySearchComponent src) throws FHIRException { 175 if (src == null || src.isEmpty()) 176 return null; 177 org.hl7.fhir.dstu2.model.Bundle.BundleEntrySearchComponent tgt = new org.hl7.fhir.dstu2.model.Bundle.BundleEntrySearchComponent(); 178 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 179 if (src.hasMode()) 180 tgt.setModeElement(convertSearchEntryMode(src.getModeElement())); 181 if (src.hasScoreElement()) 182 tgt.setScoreElement(Decimal10_30.convertDecimal(src.getScoreElement())); 183 return tgt; 184 } 185 186 public static org.hl7.fhir.dstu3.model.Bundle.BundleEntrySearchComponent convertBundleEntrySearchComponent(org.hl7.fhir.dstu2.model.Bundle.BundleEntrySearchComponent src) throws FHIRException { 187 if (src == null || src.isEmpty()) 188 return null; 189 org.hl7.fhir.dstu3.model.Bundle.BundleEntrySearchComponent tgt = new org.hl7.fhir.dstu3.model.Bundle.BundleEntrySearchComponent(); 190 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 191 if (src.hasMode()) 192 tgt.setModeElement(convertSearchEntryMode(src.getModeElement())); 193 if (src.hasScoreElement()) 194 tgt.setScoreElement(Decimal10_30.convertDecimal(src.getScoreElement())); 195 return tgt; 196 } 197 198 public static org.hl7.fhir.dstu2.model.Bundle.BundleLinkComponent convertBundleLinkComponent(org.hl7.fhir.dstu3.model.Bundle.BundleLinkComponent src) throws FHIRException { 199 if (src == null || src.isEmpty()) 200 return null; 201 org.hl7.fhir.dstu2.model.Bundle.BundleLinkComponent tgt = new org.hl7.fhir.dstu2.model.Bundle.BundleLinkComponent(); 202 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 203 if (src.hasRelationElement()) 204 tgt.setRelationElement(String10_30.convertString(src.getRelationElement())); 205 if (src.hasUrlElement()) 206 tgt.setUrlElement(Uri10_30.convertUri(src.getUrlElement())); 207 return tgt; 208 } 209 210 public static org.hl7.fhir.dstu3.model.Bundle.BundleLinkComponent convertBundleLinkComponent(org.hl7.fhir.dstu2.model.Bundle.BundleLinkComponent src) throws FHIRException { 211 if (src == null || src.isEmpty()) 212 return null; 213 org.hl7.fhir.dstu3.model.Bundle.BundleLinkComponent tgt = new org.hl7.fhir.dstu3.model.Bundle.BundleLinkComponent(); 214 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 215 if (src.hasRelationElement()) 216 tgt.setRelationElement(String10_30.convertString(src.getRelationElement())); 217 if (src.hasUrlElement()) 218 tgt.setUrlElement(Uri10_30.convertUri(src.getUrlElement())); 219 return tgt; 220 } 221 222 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Bundle.BundleType> convertBundleType(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Bundle.BundleType> src) throws FHIRException { 223 if (src == null || src.isEmpty()) 224 return null; 225 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Bundle.BundleType> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.Bundle.BundleTypeEnumFactory()); 226 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 227 switch (src.getValue()) { 228 case DOCUMENT: 229 tgt.setValue(org.hl7.fhir.dstu3.model.Bundle.BundleType.DOCUMENT); 230 break; 231 case MESSAGE: 232 tgt.setValue(org.hl7.fhir.dstu3.model.Bundle.BundleType.MESSAGE); 233 break; 234 case TRANSACTION: 235 tgt.setValue(org.hl7.fhir.dstu3.model.Bundle.BundleType.TRANSACTION); 236 break; 237 case TRANSACTIONRESPONSE: 238 tgt.setValue(org.hl7.fhir.dstu3.model.Bundle.BundleType.TRANSACTIONRESPONSE); 239 break; 240 case BATCH: 241 tgt.setValue(org.hl7.fhir.dstu3.model.Bundle.BundleType.BATCH); 242 break; 243 case BATCHRESPONSE: 244 tgt.setValue(org.hl7.fhir.dstu3.model.Bundle.BundleType.BATCHRESPONSE); 245 break; 246 case HISTORY: 247 tgt.setValue(org.hl7.fhir.dstu3.model.Bundle.BundleType.HISTORY); 248 break; 249 case SEARCHSET: 250 tgt.setValue(org.hl7.fhir.dstu3.model.Bundle.BundleType.SEARCHSET); 251 break; 252 case COLLECTION: 253 tgt.setValue(org.hl7.fhir.dstu3.model.Bundle.BundleType.COLLECTION); 254 break; 255 default: 256 tgt.setValue(org.hl7.fhir.dstu3.model.Bundle.BundleType.NULL); 257 break; 258 } 259 return tgt; 260 } 261 262 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Bundle.BundleType> convertBundleType(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Bundle.BundleType> src) throws FHIRException { 263 if (src == null || src.isEmpty()) 264 return null; 265 org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Bundle.BundleType> tgt = new org.hl7.fhir.dstu2.model.Enumeration<>(new org.hl7.fhir.dstu2.model.Bundle.BundleTypeEnumFactory()); 266 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 267 switch (src.getValue()) { 268 case DOCUMENT: 269 tgt.setValue(org.hl7.fhir.dstu2.model.Bundle.BundleType.DOCUMENT); 270 break; 271 case MESSAGE: 272 tgt.setValue(org.hl7.fhir.dstu2.model.Bundle.BundleType.MESSAGE); 273 break; 274 case TRANSACTION: 275 tgt.setValue(org.hl7.fhir.dstu2.model.Bundle.BundleType.TRANSACTION); 276 break; 277 case TRANSACTIONRESPONSE: 278 tgt.setValue(org.hl7.fhir.dstu2.model.Bundle.BundleType.TRANSACTIONRESPONSE); 279 break; 280 case BATCH: 281 tgt.setValue(org.hl7.fhir.dstu2.model.Bundle.BundleType.BATCH); 282 break; 283 case BATCHRESPONSE: 284 tgt.setValue(org.hl7.fhir.dstu2.model.Bundle.BundleType.BATCHRESPONSE); 285 break; 286 case HISTORY: 287 tgt.setValue(org.hl7.fhir.dstu2.model.Bundle.BundleType.HISTORY); 288 break; 289 case SEARCHSET: 290 tgt.setValue(org.hl7.fhir.dstu2.model.Bundle.BundleType.SEARCHSET); 291 break; 292 case COLLECTION: 293 tgt.setValue(org.hl7.fhir.dstu2.model.Bundle.BundleType.COLLECTION); 294 break; 295 default: 296 tgt.setValue(org.hl7.fhir.dstu2.model.Bundle.BundleType.NULL); 297 break; 298 } 299 return tgt; 300 } 301 302 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Bundle.HTTPVerb> convertHTTPVerb(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Bundle.HTTPVerb> src) throws FHIRException { 303 if (src == null || src.isEmpty()) 304 return null; 305 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Bundle.HTTPVerb> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.Bundle.HTTPVerbEnumFactory()); 306 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 307 switch (src.getValue()) { 308 case GET: 309 tgt.setValue(org.hl7.fhir.dstu3.model.Bundle.HTTPVerb.GET); 310 break; 311 case POST: 312 tgt.setValue(org.hl7.fhir.dstu3.model.Bundle.HTTPVerb.POST); 313 break; 314 case PUT: 315 tgt.setValue(org.hl7.fhir.dstu3.model.Bundle.HTTPVerb.PUT); 316 break; 317 case DELETE: 318 tgt.setValue(org.hl7.fhir.dstu3.model.Bundle.HTTPVerb.DELETE); 319 break; 320 default: 321 tgt.setValue(org.hl7.fhir.dstu3.model.Bundle.HTTPVerb.NULL); 322 break; 323 } 324 return tgt; 325 } 326 327 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Bundle.HTTPVerb> convertHTTPVerb(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Bundle.HTTPVerb> src) throws FHIRException { 328 if (src == null || src.isEmpty()) 329 return null; 330 org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Bundle.HTTPVerb> tgt = new org.hl7.fhir.dstu2.model.Enumeration<>(new org.hl7.fhir.dstu2.model.Bundle.HTTPVerbEnumFactory()); 331 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 332 switch (src.getValue()) { 333 case GET: 334 tgt.setValue(org.hl7.fhir.dstu2.model.Bundle.HTTPVerb.GET); 335 break; 336 case POST: 337 tgt.setValue(org.hl7.fhir.dstu2.model.Bundle.HTTPVerb.POST); 338 break; 339 case PUT: 340 tgt.setValue(org.hl7.fhir.dstu2.model.Bundle.HTTPVerb.PUT); 341 break; 342 case DELETE: 343 tgt.setValue(org.hl7.fhir.dstu2.model.Bundle.HTTPVerb.DELETE); 344 break; 345 default: 346 tgt.setValue(org.hl7.fhir.dstu2.model.Bundle.HTTPVerb.NULL); 347 break; 348 } 349 return tgt; 350 } 351 352 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Bundle.SearchEntryMode> convertSearchEntryMode(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Bundle.SearchEntryMode> src) throws FHIRException { 353 if (src == null || src.isEmpty()) 354 return null; 355 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Bundle.SearchEntryMode> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.Bundle.SearchEntryModeEnumFactory()); 356 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 357 switch (src.getValue()) { 358 case MATCH: 359 tgt.setValue(org.hl7.fhir.dstu3.model.Bundle.SearchEntryMode.MATCH); 360 break; 361 case INCLUDE: 362 tgt.setValue(org.hl7.fhir.dstu3.model.Bundle.SearchEntryMode.INCLUDE); 363 break; 364 case OUTCOME: 365 tgt.setValue(org.hl7.fhir.dstu3.model.Bundle.SearchEntryMode.OUTCOME); 366 break; 367 default: 368 tgt.setValue(org.hl7.fhir.dstu3.model.Bundle.SearchEntryMode.NULL); 369 break; 370 } 371 return tgt; 372 } 373 374 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Bundle.SearchEntryMode> convertSearchEntryMode(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Bundle.SearchEntryMode> src) throws FHIRException { 375 if (src == null || src.isEmpty()) 376 return null; 377 org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Bundle.SearchEntryMode> tgt = new org.hl7.fhir.dstu2.model.Enumeration<>(new org.hl7.fhir.dstu2.model.Bundle.SearchEntryModeEnumFactory()); 378 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 379 switch (src.getValue()) { 380 case MATCH: 381 tgt.setValue(org.hl7.fhir.dstu2.model.Bundle.SearchEntryMode.MATCH); 382 break; 383 case INCLUDE: 384 tgt.setValue(org.hl7.fhir.dstu2.model.Bundle.SearchEntryMode.INCLUDE); 385 break; 386 case OUTCOME: 387 tgt.setValue(org.hl7.fhir.dstu2.model.Bundle.SearchEntryMode.OUTCOME); 388 break; 389 default: 390 tgt.setValue(org.hl7.fhir.dstu2.model.Bundle.SearchEntryMode.NULL); 391 break; 392 } 393 return tgt; 394 } 395}