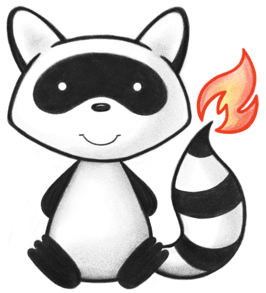
001package org.hl7.fhir.convertors.conv10_30.resources10_30; 002 003import org.hl7.fhir.convertors.context.ConversionContext10_30; 004import org.hl7.fhir.convertors.conv10_30.datatypes10_30.Reference10_30; 005import org.hl7.fhir.convertors.conv10_30.datatypes10_30.complextypes10_30.Annotation10_30; 006import org.hl7.fhir.convertors.conv10_30.datatypes10_30.complextypes10_30.CodeableConcept10_30; 007import org.hl7.fhir.convertors.conv10_30.datatypes10_30.complextypes10_30.Identifier10_30; 008import org.hl7.fhir.convertors.conv10_30.datatypes10_30.complextypes10_30.Period10_30; 009import org.hl7.fhir.convertors.conv10_30.datatypes10_30.complextypes10_30.SimpleQuantity10_30; 010import org.hl7.fhir.convertors.conv10_30.datatypes10_30.primitivetypes10_30.Boolean10_30; 011import org.hl7.fhir.convertors.conv10_30.datatypes10_30.primitivetypes10_30.String10_30; 012import org.hl7.fhir.exceptions.FHIRException; 013 014public class CarePlan10_30 { 015 016 public static org.hl7.fhir.dstu3.model.CarePlan convertCarePlan(org.hl7.fhir.dstu2.model.CarePlan src) throws FHIRException { 017 if (src == null || src.isEmpty()) 018 return null; 019 org.hl7.fhir.dstu3.model.CarePlan tgt = new org.hl7.fhir.dstu3.model.CarePlan(); 020 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyDomainResource(src, tgt); 021 for (org.hl7.fhir.dstu2.model.Identifier t : src.getIdentifier()) 022 tgt.addIdentifier(Identifier10_30.convertIdentifier(t)); 023 if (src.hasSubject()) 024 tgt.setSubject(Reference10_30.convertReference(src.getSubject())); 025 if (src.hasStatus()) 026 tgt.setStatusElement(convertCarePlanStatus(src.getStatusElement())); 027 if (src.hasContext()) 028 tgt.setContext(Reference10_30.convertReference(src.getContext())); 029 if (src.hasPeriod()) 030 tgt.setPeriod(Period10_30.convertPeriod(src.getPeriod())); 031 for (org.hl7.fhir.dstu2.model.Reference t : src.getAuthor()) tgt.addAuthor(Reference10_30.convertReference(t)); 032 for (org.hl7.fhir.dstu2.model.CodeableConcept t : src.getCategory()) 033 tgt.addCategory(CodeableConcept10_30.convertCodeableConcept(t)); 034 if (src.hasDescriptionElement()) 035 tgt.setDescriptionElement(String10_30.convertString(src.getDescriptionElement())); 036 for (org.hl7.fhir.dstu2.model.Reference t : src.getAddresses()) 037 tgt.addAddresses(Reference10_30.convertReference(t)); 038 for (org.hl7.fhir.dstu2.model.Reference t : src.getGoal()) tgt.addGoal(Reference10_30.convertReference(t)); 039 for (org.hl7.fhir.dstu2.model.CarePlan.CarePlanActivityComponent t : src.getActivity()) 040 tgt.addActivity(convertCarePlanActivityComponent(t)); 041 return tgt; 042 } 043 044 public static org.hl7.fhir.dstu2.model.CarePlan convertCarePlan(org.hl7.fhir.dstu3.model.CarePlan src) throws FHIRException { 045 if (src == null || src.isEmpty()) 046 return null; 047 org.hl7.fhir.dstu2.model.CarePlan tgt = new org.hl7.fhir.dstu2.model.CarePlan(); 048 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyDomainResource(src, tgt); 049 for (org.hl7.fhir.dstu3.model.Identifier t : src.getIdentifier()) 050 tgt.addIdentifier(Identifier10_30.convertIdentifier(t)); 051 if (src.hasSubject()) 052 tgt.setSubject(Reference10_30.convertReference(src.getSubject())); 053 if (src.hasStatus()) 054 tgt.setStatusElement(convertCarePlanStatus(src.getStatusElement())); 055 if (src.hasContext()) 056 tgt.setContext(Reference10_30.convertReference(src.getContext())); 057 if (src.hasPeriod()) 058 tgt.setPeriod(Period10_30.convertPeriod(src.getPeriod())); 059 for (org.hl7.fhir.dstu3.model.Reference t : src.getAuthor()) tgt.addAuthor(Reference10_30.convertReference(t)); 060 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getCategory()) 061 tgt.addCategory(CodeableConcept10_30.convertCodeableConcept(t)); 062 if (src.hasDescriptionElement()) 063 tgt.setDescriptionElement(String10_30.convertString(src.getDescriptionElement())); 064 for (org.hl7.fhir.dstu3.model.Reference t : src.getAddresses()) 065 tgt.addAddresses(Reference10_30.convertReference(t)); 066 for (org.hl7.fhir.dstu3.model.Reference t : src.getGoal()) tgt.addGoal(Reference10_30.convertReference(t)); 067 for (org.hl7.fhir.dstu3.model.CarePlan.CarePlanActivityComponent t : src.getActivity()) 068 tgt.addActivity(convertCarePlanActivityComponent(t)); 069 return tgt; 070 } 071 072 public static org.hl7.fhir.dstu2.model.CarePlan.CarePlanActivityComponent convertCarePlanActivityComponent(org.hl7.fhir.dstu3.model.CarePlan.CarePlanActivityComponent src) throws FHIRException { 073 if (src == null || src.isEmpty()) 074 return null; 075 org.hl7.fhir.dstu2.model.CarePlan.CarePlanActivityComponent tgt = new org.hl7.fhir.dstu2.model.CarePlan.CarePlanActivityComponent(); 076 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 077 for (org.hl7.fhir.dstu3.model.Annotation t : src.getProgress()) 078 tgt.addProgress(Annotation10_30.convertAnnotation(t)); 079 if (src.hasReference()) 080 tgt.setReference(Reference10_30.convertReference(src.getReference())); 081 if (src.hasDetail()) 082 tgt.setDetail(convertCarePlanActivityDetailComponent(src.getDetail())); 083 return tgt; 084 } 085 086 public static org.hl7.fhir.dstu3.model.CarePlan.CarePlanActivityComponent convertCarePlanActivityComponent(org.hl7.fhir.dstu2.model.CarePlan.CarePlanActivityComponent src) throws FHIRException { 087 if (src == null || src.isEmpty()) 088 return null; 089 org.hl7.fhir.dstu3.model.CarePlan.CarePlanActivityComponent tgt = new org.hl7.fhir.dstu3.model.CarePlan.CarePlanActivityComponent(); 090 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 091 for (org.hl7.fhir.dstu2.model.Annotation t : src.getProgress()) 092 tgt.addProgress(Annotation10_30.convertAnnotation(t)); 093 if (src.hasReference()) 094 tgt.setReference(Reference10_30.convertReference(src.getReference())); 095 if (src.hasDetail()) 096 tgt.setDetail(convertCarePlanActivityDetailComponent(src.getDetail())); 097 return tgt; 098 } 099 100 public static org.hl7.fhir.dstu3.model.CarePlan.CarePlanActivityDetailComponent convertCarePlanActivityDetailComponent(org.hl7.fhir.dstu2.model.CarePlan.CarePlanActivityDetailComponent src) throws FHIRException { 101 if (src == null || src.isEmpty()) 102 return null; 103 org.hl7.fhir.dstu3.model.CarePlan.CarePlanActivityDetailComponent tgt = new org.hl7.fhir.dstu3.model.CarePlan.CarePlanActivityDetailComponent(); 104 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 105 if (src.hasCategory()) 106 tgt.setCategory(CodeableConcept10_30.convertCodeableConcept(src.getCategory())); 107 if (src.hasCode()) 108 tgt.setCode(CodeableConcept10_30.convertCodeableConcept(src.getCode())); 109 for (org.hl7.fhir.dstu2.model.CodeableConcept t : src.getReasonCode()) 110 tgt.addReasonCode(CodeableConcept10_30.convertCodeableConcept(t)); 111 for (org.hl7.fhir.dstu2.model.Reference t : src.getReasonReference()) 112 tgt.addReasonReference(Reference10_30.convertReference(t)); 113 for (org.hl7.fhir.dstu2.model.Reference t : src.getGoal()) tgt.addGoal(Reference10_30.convertReference(t)); 114 if (src.hasStatus()) 115 tgt.setStatusElement(convertCarePlanActivityStatus(src.getStatusElement())); 116 if (src.hasProhibitedElement()) 117 tgt.setProhibitedElement(Boolean10_30.convertBoolean(src.getProhibitedElement())); 118 if (src.hasScheduled()) 119 tgt.setScheduled(ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().convertType(src.getScheduled())); 120 if (src.hasLocation()) 121 tgt.setLocation(Reference10_30.convertReference(src.getLocation())); 122 for (org.hl7.fhir.dstu2.model.Reference t : src.getPerformer()) 123 tgt.addPerformer(Reference10_30.convertReference(t)); 124 if (src.hasProduct()) 125 tgt.setProduct(ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().convertType(src.getProduct())); 126 if (src.hasDailyAmount()) 127 tgt.setDailyAmount(SimpleQuantity10_30.convertSimpleQuantity(src.getDailyAmount())); 128 if (src.hasQuantity()) 129 tgt.setQuantity(SimpleQuantity10_30.convertSimpleQuantity(src.getQuantity())); 130 if (src.hasDescriptionElement()) 131 tgt.setDescriptionElement(String10_30.convertString(src.getDescriptionElement())); 132 return tgt; 133 } 134 135 public static org.hl7.fhir.dstu2.model.CarePlan.CarePlanActivityDetailComponent convertCarePlanActivityDetailComponent(org.hl7.fhir.dstu3.model.CarePlan.CarePlanActivityDetailComponent src) throws FHIRException { 136 if (src == null || src.isEmpty()) 137 return null; 138 org.hl7.fhir.dstu2.model.CarePlan.CarePlanActivityDetailComponent tgt = new org.hl7.fhir.dstu2.model.CarePlan.CarePlanActivityDetailComponent(); 139 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 140 if (src.hasCategory()) 141 tgt.setCategory(CodeableConcept10_30.convertCodeableConcept(src.getCategory())); 142 if (src.hasCode()) 143 tgt.setCode(CodeableConcept10_30.convertCodeableConcept(src.getCode())); 144 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getReasonCode()) 145 tgt.addReasonCode(CodeableConcept10_30.convertCodeableConcept(t)); 146 for (org.hl7.fhir.dstu3.model.Reference t : src.getReasonReference()) 147 tgt.addReasonReference(Reference10_30.convertReference(t)); 148 for (org.hl7.fhir.dstu3.model.Reference t : src.getGoal()) tgt.addGoal(Reference10_30.convertReference(t)); 149 if (src.hasStatus()) 150 tgt.setStatusElement(convertCarePlanActivityStatus(src.getStatusElement())); 151 if (src.hasProhibitedElement()) 152 tgt.setProhibitedElement(Boolean10_30.convertBoolean(src.getProhibitedElement())); 153 if (src.hasScheduled()) 154 tgt.setScheduled(ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().convertType(src.getScheduled())); 155 if (src.hasLocation()) 156 tgt.setLocation(Reference10_30.convertReference(src.getLocation())); 157 for (org.hl7.fhir.dstu3.model.Reference t : src.getPerformer()) 158 tgt.addPerformer(Reference10_30.convertReference(t)); 159 if (src.hasProduct()) 160 tgt.setProduct(ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().convertType(src.getProduct())); 161 if (src.hasDailyAmount()) 162 tgt.setDailyAmount(SimpleQuantity10_30.convertSimpleQuantity(src.getDailyAmount())); 163 if (src.hasQuantity()) 164 tgt.setQuantity(SimpleQuantity10_30.convertSimpleQuantity(src.getQuantity())); 165 if (src.hasDescriptionElement()) 166 tgt.setDescriptionElement(String10_30.convertString(src.getDescriptionElement())); 167 return tgt; 168 } 169 170 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.CarePlan.CarePlanActivityStatus> convertCarePlanActivityStatus(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.CarePlan.CarePlanActivityStatus> src) throws FHIRException { 171 if (src == null || src.isEmpty()) 172 return null; 173 org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.CarePlan.CarePlanActivityStatus> tgt = new org.hl7.fhir.dstu2.model.Enumeration<>(new org.hl7.fhir.dstu2.model.CarePlan.CarePlanActivityStatusEnumFactory()); 174 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 175 switch (src.getValue()) { 176 case NOTSTARTED: 177 tgt.setValue(org.hl7.fhir.dstu2.model.CarePlan.CarePlanActivityStatus.NOTSTARTED); 178 break; 179 case SCHEDULED: 180 tgt.setValue(org.hl7.fhir.dstu2.model.CarePlan.CarePlanActivityStatus.SCHEDULED); 181 break; 182 case INPROGRESS: 183 tgt.setValue(org.hl7.fhir.dstu2.model.CarePlan.CarePlanActivityStatus.INPROGRESS); 184 break; 185 case ONHOLD: 186 tgt.setValue(org.hl7.fhir.dstu2.model.CarePlan.CarePlanActivityStatus.ONHOLD); 187 break; 188 case COMPLETED: 189 tgt.setValue(org.hl7.fhir.dstu2.model.CarePlan.CarePlanActivityStatus.COMPLETED); 190 break; 191 case CANCELLED: 192 tgt.setValue(org.hl7.fhir.dstu2.model.CarePlan.CarePlanActivityStatus.CANCELLED); 193 break; 194 default: 195 tgt.setValue(org.hl7.fhir.dstu2.model.CarePlan.CarePlanActivityStatus.NULL); 196 break; 197 } 198 return tgt; 199 } 200 201 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.CarePlan.CarePlanActivityStatus> convertCarePlanActivityStatus(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.CarePlan.CarePlanActivityStatus> src) throws FHIRException { 202 if (src == null || src.isEmpty()) 203 return null; 204 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.CarePlan.CarePlanActivityStatus> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.CarePlan.CarePlanActivityStatusEnumFactory()); 205 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 206 switch (src.getValue()) { 207 case NOTSTARTED: 208 tgt.setValue(org.hl7.fhir.dstu3.model.CarePlan.CarePlanActivityStatus.NOTSTARTED); 209 break; 210 case SCHEDULED: 211 tgt.setValue(org.hl7.fhir.dstu3.model.CarePlan.CarePlanActivityStatus.SCHEDULED); 212 break; 213 case INPROGRESS: 214 tgt.setValue(org.hl7.fhir.dstu3.model.CarePlan.CarePlanActivityStatus.INPROGRESS); 215 break; 216 case ONHOLD: 217 tgt.setValue(org.hl7.fhir.dstu3.model.CarePlan.CarePlanActivityStatus.ONHOLD); 218 break; 219 case COMPLETED: 220 tgt.setValue(org.hl7.fhir.dstu3.model.CarePlan.CarePlanActivityStatus.COMPLETED); 221 break; 222 case CANCELLED: 223 tgt.setValue(org.hl7.fhir.dstu3.model.CarePlan.CarePlanActivityStatus.CANCELLED); 224 break; 225 default: 226 tgt.setValue(org.hl7.fhir.dstu3.model.CarePlan.CarePlanActivityStatus.NULL); 227 break; 228 } 229 return tgt; 230 } 231 232 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.CarePlan.CarePlanStatus> convertCarePlanStatus(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.CarePlan.CarePlanStatus> src) throws FHIRException { 233 if (src == null || src.isEmpty()) 234 return null; 235 org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.CarePlan.CarePlanStatus> tgt = new org.hl7.fhir.dstu2.model.Enumeration<>(new org.hl7.fhir.dstu2.model.CarePlan.CarePlanStatusEnumFactory()); 236 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 237 switch (src.getValue()) { 238 case DRAFT: 239 tgt.setValue(org.hl7.fhir.dstu2.model.CarePlan.CarePlanStatus.DRAFT); 240 break; 241 case ACTIVE: 242 tgt.setValue(org.hl7.fhir.dstu2.model.CarePlan.CarePlanStatus.ACTIVE); 243 break; 244 case COMPLETED: 245 tgt.setValue(org.hl7.fhir.dstu2.model.CarePlan.CarePlanStatus.COMPLETED); 246 break; 247 case CANCELLED: 248 tgt.setValue(org.hl7.fhir.dstu2.model.CarePlan.CarePlanStatus.CANCELLED); 249 break; 250 default: 251 tgt.setValue(org.hl7.fhir.dstu2.model.CarePlan.CarePlanStatus.NULL); 252 break; 253 } 254 return tgt; 255 } 256 257 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.CarePlan.CarePlanStatus> convertCarePlanStatus(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.CarePlan.CarePlanStatus> src) throws FHIRException { 258 if (src == null || src.isEmpty()) 259 return null; 260 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.CarePlan.CarePlanStatus> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.CarePlan.CarePlanStatusEnumFactory()); 261 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 262 switch (src.getValue()) { 263 case PROPOSED: 264 tgt.setValue(org.hl7.fhir.dstu3.model.CarePlan.CarePlanStatus.DRAFT); 265 break; 266 case DRAFT: 267 tgt.setValue(org.hl7.fhir.dstu3.model.CarePlan.CarePlanStatus.DRAFT); 268 break; 269 case ACTIVE: 270 tgt.setValue(org.hl7.fhir.dstu3.model.CarePlan.CarePlanStatus.ACTIVE); 271 break; 272 case COMPLETED: 273 tgt.setValue(org.hl7.fhir.dstu3.model.CarePlan.CarePlanStatus.COMPLETED); 274 break; 275 case CANCELLED: 276 tgt.setValue(org.hl7.fhir.dstu3.model.CarePlan.CarePlanStatus.CANCELLED); 277 break; 278 default: 279 tgt.setValue(org.hl7.fhir.dstu3.model.CarePlan.CarePlanStatus.NULL); 280 break; 281 } 282 return tgt; 283 } 284}