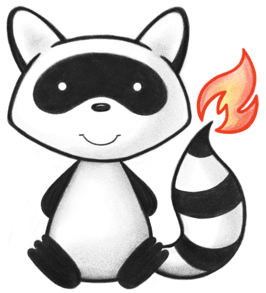
001package org.hl7.fhir.convertors.conv10_30.resources10_30; 002 003import org.hl7.fhir.convertors.context.ConversionContext10_30; 004import org.hl7.fhir.convertors.conv10_30.datatypes10_30.Reference10_30; 005import org.hl7.fhir.convertors.conv10_30.datatypes10_30.complextypes10_30.Annotation10_30; 006import org.hl7.fhir.convertors.conv10_30.datatypes10_30.complextypes10_30.CodeableConcept10_30; 007import org.hl7.fhir.convertors.conv10_30.datatypes10_30.complextypes10_30.ContactPoint10_30; 008import org.hl7.fhir.convertors.conv10_30.datatypes10_30.complextypes10_30.Identifier10_30; 009import org.hl7.fhir.convertors.conv10_30.datatypes10_30.primitivetypes10_30.DateTime10_30; 010import org.hl7.fhir.convertors.conv10_30.datatypes10_30.primitivetypes10_30.String10_30; 011import org.hl7.fhir.convertors.conv10_30.datatypes10_30.primitivetypes10_30.Uri10_30; 012import org.hl7.fhir.exceptions.FHIRException; 013 014public class Device10_30 { 015 016 public static org.hl7.fhir.dstu2.model.Device convertDevice(org.hl7.fhir.dstu3.model.Device src) throws FHIRException { 017 if (src == null || src.isEmpty()) 018 return null; 019 org.hl7.fhir.dstu2.model.Device tgt = new org.hl7.fhir.dstu2.model.Device(); 020 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyDomainResource(src, tgt); 021 for (org.hl7.fhir.dstu3.model.Identifier t : src.getIdentifier()) 022 tgt.addIdentifier(Identifier10_30.convertIdentifier(t)); 023 if (src.hasUdi()) 024 tgt.setUdi(src.getUdi().getDeviceIdentifier()); 025 if (src.hasStatus()) 026 tgt.setStatusElement(convertDeviceStatus(src.getStatusElement())); 027 if (src.hasType()) 028 tgt.setType(CodeableConcept10_30.convertCodeableConcept(src.getType())); 029 if (src.hasLotNumberElement()) 030 tgt.setLotNumberElement(String10_30.convertString(src.getLotNumberElement())); 031 if (src.hasManufacturerElement()) 032 tgt.setManufacturerElement(String10_30.convertString(src.getManufacturerElement())); 033 if (src.hasManufactureDateElement()) 034 tgt.setManufactureDateElement(DateTime10_30.convertDateTime(src.getManufactureDateElement())); 035 if (src.hasExpirationDateElement()) 036 tgt.setExpiryElement(DateTime10_30.convertDateTime(src.getExpirationDateElement())); 037 if (src.hasModelElement()) 038 tgt.setModelElement(String10_30.convertString(src.getModelElement())); 039 if (src.hasVersionElement()) 040 tgt.setVersionElement(String10_30.convertString(src.getVersionElement())); 041 if (src.hasPatient()) 042 tgt.setPatient(Reference10_30.convertReference(src.getPatient())); 043 if (src.hasOwner()) 044 tgt.setOwner(Reference10_30.convertReference(src.getOwner())); 045 for (org.hl7.fhir.dstu3.model.ContactPoint t : src.getContact()) 046 tgt.addContact(ContactPoint10_30.convertContactPoint(t)); 047 if (src.hasLocation()) 048 tgt.setLocation(Reference10_30.convertReference(src.getLocation())); 049 if (src.hasUrlElement()) 050 tgt.setUrlElement(Uri10_30.convertUri(src.getUrlElement())); 051 for (org.hl7.fhir.dstu3.model.Annotation t : src.getNote()) tgt.addNote(Annotation10_30.convertAnnotation(t)); 052 return tgt; 053 } 054 055 public static org.hl7.fhir.dstu3.model.Device convertDevice(org.hl7.fhir.dstu2.model.Device src) throws FHIRException { 056 if (src == null || src.isEmpty()) 057 return null; 058 org.hl7.fhir.dstu3.model.Device tgt = new org.hl7.fhir.dstu3.model.Device(); 059 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyDomainResource(src, tgt); 060 for (org.hl7.fhir.dstu2.model.Identifier t : src.getIdentifier()) 061 tgt.addIdentifier(Identifier10_30.convertIdentifier(t)); 062 if (src.hasUdi()) 063 tgt.setUdi((new org.hl7.fhir.dstu3.model.Device.DeviceUdiComponent()).setDeviceIdentifier(src.getUdi())); 064 if (src.hasStatus()) 065 tgt.setStatusElement(convertDeviceStatus(src.getStatusElement())); 066 if (src.hasType()) 067 tgt.setType(CodeableConcept10_30.convertCodeableConcept(src.getType())); 068 if (src.hasLotNumberElement()) 069 tgt.setLotNumberElement(String10_30.convertString(src.getLotNumberElement())); 070 if (src.hasManufacturerElement()) 071 tgt.setManufacturerElement(String10_30.convertString(src.getManufacturerElement())); 072 if (src.hasManufactureDateElement()) 073 tgt.setManufactureDateElement(DateTime10_30.convertDateTime(src.getManufactureDateElement())); 074 if (src.hasExpiryElement()) 075 tgt.setExpirationDateElement(DateTime10_30.convertDateTime(src.getExpiryElement())); 076 if (src.hasModelElement()) 077 tgt.setModelElement(String10_30.convertString(src.getModelElement())); 078 if (src.hasVersionElement()) 079 tgt.setVersionElement(String10_30.convertString(src.getVersionElement())); 080 if (src.hasPatient()) 081 tgt.setPatient(Reference10_30.convertReference(src.getPatient())); 082 if (src.hasOwner()) 083 tgt.setOwner(Reference10_30.convertReference(src.getOwner())); 084 for (org.hl7.fhir.dstu2.model.ContactPoint t : src.getContact()) 085 tgt.addContact(ContactPoint10_30.convertContactPoint(t)); 086 if (src.hasLocation()) 087 tgt.setLocation(Reference10_30.convertReference(src.getLocation())); 088 if (src.hasUrlElement()) 089 tgt.setUrlElement(Uri10_30.convertUri(src.getUrlElement())); 090 for (org.hl7.fhir.dstu2.model.Annotation t : src.getNote()) tgt.addNote(Annotation10_30.convertAnnotation(t)); 091 return tgt; 092 } 093 094 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Device.FHIRDeviceStatus> convertDeviceStatus(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Device.DeviceStatus> src) throws FHIRException { 095 if (src == null || src.isEmpty()) 096 return null; 097 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Device.FHIRDeviceStatus> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.Device.FHIRDeviceStatusEnumFactory()); 098 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 099 switch (src.getValue()) { 100 case AVAILABLE: 101 tgt.setValue(org.hl7.fhir.dstu3.model.Device.FHIRDeviceStatus.ACTIVE); 102 break; 103 case NOTAVAILABLE: 104 tgt.setValue(org.hl7.fhir.dstu3.model.Device.FHIRDeviceStatus.INACTIVE); 105 break; 106 case ENTEREDINERROR: 107 tgt.setValue(org.hl7.fhir.dstu3.model.Device.FHIRDeviceStatus.ENTEREDINERROR); 108 break; 109 default: 110 tgt.setValue(org.hl7.fhir.dstu3.model.Device.FHIRDeviceStatus.NULL); 111 break; 112 } 113 return tgt; 114 } 115 116 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Device.DeviceStatus> convertDeviceStatus(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Device.FHIRDeviceStatus> src) throws FHIRException { 117 if (src == null || src.isEmpty()) 118 return null; 119 org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Device.DeviceStatus> tgt = new org.hl7.fhir.dstu2.model.Enumeration<>(new org.hl7.fhir.dstu2.model.Device.DeviceStatusEnumFactory()); 120 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 121 switch (src.getValue()) { 122 case ACTIVE: 123 tgt.setValue(org.hl7.fhir.dstu2.model.Device.DeviceStatus.AVAILABLE); 124 break; 125 case INACTIVE: 126 tgt.setValue(org.hl7.fhir.dstu2.model.Device.DeviceStatus.NOTAVAILABLE); 127 break; 128 case ENTEREDINERROR: 129 tgt.setValue(org.hl7.fhir.dstu2.model.Device.DeviceStatus.ENTEREDINERROR); 130 break; 131 default: 132 tgt.setValue(org.hl7.fhir.dstu2.model.Device.DeviceStatus.NULL); 133 break; 134 } 135 return tgt; 136 } 137}